在Python中保存爬取的图片,可以使用requests库、os库和PIL库等。首先,使用requests库从网页下载图片数据,然后使用os库确保存储目录存在,最后将图片保存到本地。本文将详细介绍如何使用Python保存爬取的图片,并提供实际代码示例。
一、安装所需库
在开始之前,确保你已经安装了所需的Python库。可以使用pip命令安装requests库和PIL库(Pillow)。
pip install requests
pip install pillow
二、爬取图片的基本步骤
- 导入所需库
- 定义图片URL
- 发送HTTP请求
- 保存图片数据
- 处理异常情况
1、导入所需库
首先,我们需要导入requests
库来发送HTTP请求,os
库来操作文件系统,以及PIL
库中的Image
模块来处理图片。
import requests
import os
from PIL import Image
from io import BytesIO
2、定义图片URL
我们需要定义一个图片的URL,以便后续发送HTTP请求获取图片数据。
image_url = 'https://example.com/image.jpg'
3、发送HTTP请求
使用requests
库的get
方法发送HTTP请求,获取图片数据。
response = requests.get(image_url)
4、保存图片数据
如果请求成功,我们将图片数据保存到本地。首先,确保存储目录存在,然后将图片数据写入文件。
if response.status_code == 200:
# 确保存储目录存在
save_path = 'images'
if not os.path.exists(save_path):
os.makedirs(save_path)
# 保存图片数据
image_path = os.path.join(save_path, 'image.jpg')
with open(image_path, 'wb') as f:
f.write(response.content)
else:
print(f'Failed to retrieve image. HTTP Status code: {response.status_code}')
5、处理异常情况
在实际应用中,可能会遇到各种异常情况,例如网络连接问题、图片URL无效等。我们需要添加异常处理代码,以便在出现异常时能够及时发现并处理。
try:
response = requests.get(image_url)
response.raise_for_status() # 如果请求失败,抛出HTTPError异常
if response.status_code == 200:
# 确保存储目录存在
save_path = 'images'
if not os.path.exists(save_path):
os.makedirs(save_path)
# 保存图片数据
image_path = os.path.join(save_path, 'image.jpg')
with open(image_path, 'wb') as f:
f.write(response.content)
print(f'Image saved successfully: {image_path}')
else:
print(f'Failed to retrieve image. HTTP Status code: {response.status_code}')
except requests.exceptions.RequestException as e:
print(f'Error occurred: {e}')
三、进阶用法
在实际项目中,我们可能需要处理更多复杂的情况,例如批量爬取图片、处理不同格式的图片等。下面介绍一些进阶用法。
1、批量爬取图片
如果需要批量爬取图片,可以将图片URL存储在列表中,然后遍历列表逐个爬取图片。
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
]
save_path = 'images'
if not os.path.exists(save_path):
os.makedirs(save_path)
for i, url in enumerate(image_urls):
try:
response = requests.get(url)
response.raise_for_status()
if response.status_code == 200:
image_path = os.path.join(save_path, f'image_{i + 1}.jpg')
with open(image_path, 'wb') as f:
f.write(response.content)
print(f'Image saved successfully: {image_path}')
else:
print(f'Failed to retrieve image from {url}. HTTP Status code: {response.status_code}')
except requests.exceptions.RequestException as e:
print(f'Error occurred while retrieving image from {url}: {e}')
2、处理不同格式的图片
在实际应用中,图片可能有不同的格式,例如JPEG、PNG等。我们可以使用PIL库中的Image
模块来处理不同格式的图片,并将其保存为指定的格式。
image_url = 'https://example.com/image.png'
try:
response = requests.get(image_url)
response.raise_for_status()
if response.status_code == 200:
image = Image.open(BytesIO(response.content))
save_path = 'images'
if not os.path.exists(save_path):
os.makedirs(save_path)
image_path = os.path.join(save_path, 'image.jpg')
image.save(image_path, format='JPEG')
print(f'Image saved successfully: {image_path}')
else:
print(f'Failed to retrieve image. HTTP Status code: {response.status_code}')
except requests.exceptions.RequestException as e:
print(f'Error occurred: {e}')
3、使用多线程加速爬取
在批量爬取图片时,可以使用多线程来加速爬取过程。Python的threading
模块可以帮助我们实现多线程爬取。
import threading
def download_image(url, save_path, index):
try:
response = requests.get(url)
response.raise_for_status()
if response.status_code == 200:
image_path = os.path.join(save_path, f'image_{index + 1}.jpg')
with open(image_path, 'wb') as f:
f.write(response.content)
print(f'Image saved successfully: {image_path}')
else:
print(f'Failed to retrieve image from {url}. HTTP Status code: {response.status_code}')
except requests.exceptions.RequestException as e:
print(f'Error occurred while retrieving image from {url}: {e}')
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
]
save_path = 'images'
if not os.path.exists(save_path):
os.makedirs(save_path)
threads = []
for i, url in enumerate(image_urls):
thread = threading.Thread(target=download_image, args=(url, save_path, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
通过使用多线程,我们可以显著提高批量爬取图片的效率。
总结:
本文介绍了在Python中保存爬取的图片的基本方法,主要包括导入所需库、定义图片URL、发送HTTP请求、保存图片数据以及处理异常情况。同时,还介绍了一些进阶用法,例如批量爬取图片、处理不同格式的图片以及使用多线程加速爬取。希望这些内容对你有所帮助。如果你有更多需求或问题,可以进一步查阅相关文档或社区资源。
相关问答FAQs:
如何在Python中下载并保存图片?
在Python中,可以使用requests库来下载图片。首先,通过requests.get()获取图片的URL,然后使用open()函数以二进制模式写入文件。示例代码如下:
import requests
url = '图片的URL'
response = requests.get(url)
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
确保在运行代码前安装了requests库,可以通过pip install requests
进行安装。
在保存图片时,有哪些常见的格式可以选择?
常见的图片格式包括JPEG、PNG、GIF、BMP等。选择适合你需求的格式,例如JPEG适合照片,PNG适合需要透明背景的图像,GIF适合简单动画。保存时只需在文件名中使用相应的后缀即可。
如何处理下载过程中可能出现的错误?
在下载图片时,可能会遇到网络错误或URL无效等问题。可以通过try-except语句来捕获这些异常,并进行相应的处理。例如,检查响应状态码以确保请求成功:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f'下载图片时出错: {e}')
这样可以保证程序的健壮性,避免因错误导致崩溃。
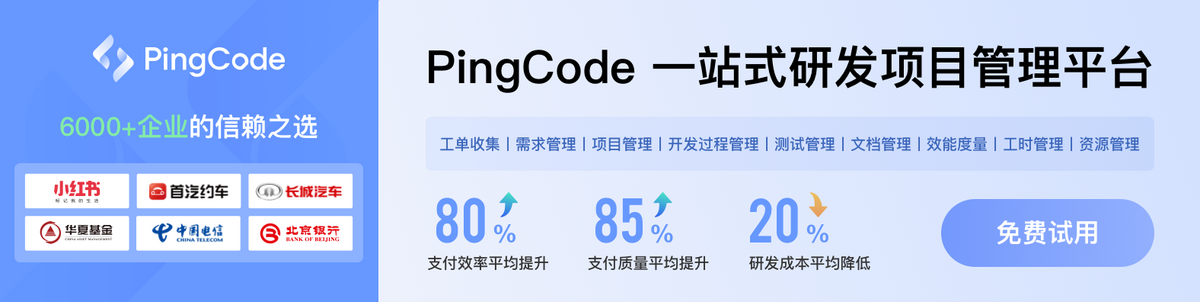