使用os库、使用pathlib库、使用sys库
Python提供了多种方法来进入目录下,常见的方法包括使用os
库、pathlib
库和sys
库。os库是最常用的库之一,它提供了丰富的函数来操作文件和目录,例如os.chdir()
可以改变当前工作目录。pathlib库是Python 3.4引入的一个模块,它提供了面向对象的路径操作方式。sys库则可以在程序启动时通过命令行参数指定工作目录。
一、使用os库
os
库是Python标准库的一部分,提供了许多与操作系统进行交互的方法。改变当前工作目录的方法是os.chdir()
。
1.1 如何使用os.chdir()
os.chdir(path)
函数用于改变当前的工作目录。path
参数可以是相对路径也可以是绝对路径。
import os
使用绝对路径
os.chdir('/path/to/directory')
使用相对路径
os.chdir('../relative/directory')
在这个例子中,os.chdir()
函数将当前工作目录更改为指定路径。使用绝对路径时,路径从根目录开始;使用相对路径时,路径相对于当前工作目录。
1.2 获取当前工作目录
为了确保工作目录已经成功更改,可以使用os.getcwd()
函数获取当前工作目录。
current_directory = os.getcwd()
print(f"Current working directory: {current_directory}")
这个代码片段将输出当前的工作目录,确保我们已经正确更改了目录。
二、使用pathlib库
pathlib
库是Python 3.4引入的一个模块,提供了面向对象的路径操作方式。pathlib.Path
类可以用来表示路径,并且可以方便地进行路径操作。
2.1 如何使用pathlib.Path
使用pathlib.Path
类,我们可以更改当前工作目录。
from pathlib import Path
使用绝对路径
path = Path('/path/to/directory')
path.cwd()
使用相对路径
relative_path = Path('../relative/directory')
relative_path.cwd()
在这个例子中,我们使用Path
类创建了路径对象,然后调用cwd()
方法更改当前工作目录。
2.2 获取当前工作目录
同样,我们可以使用pathlib
库获取当前工作目录。
current_directory = Path.cwd()
print(f"Current working directory: {current_directory}")
这个代码片段将输出当前的工作目录,确保我们已经正确更改了目录。
三、使用sys库
sys
库提供了访问与Python解释器相关的变量和函数。通过命令行参数,我们可以在程序启动时指定工作目录。
3.1 如何使用sys.argv
使用sys.argv
,我们可以在程序启动时传递目录路径作为参数。
import sys
import os
if len(sys.argv) > 1:
directory = sys.argv[1]
os.chdir(directory)
else:
print("Please provide a directory path")
在这个例子中,我们使用sys.argv[1]
获取命令行参数并更改当前工作目录。如果没有提供参数,程序将提示用户提供目录路径。
3.2 获取当前工作目录
同样,我们可以使用os.getcwd()
函数获取当前工作目录。
current_directory = os.getcwd()
print(f"Current working directory: {current_directory}")
这个代码片段将输出当前的工作目录,确保我们已经正确更改了目录。
四、综合使用
在实际开发中,我们可以综合使用以上方法来更改和获取工作目录。以下是一个综合示例,展示了如何使用os
库、pathlib
库和sys
库来操作工作目录。
import os
from pathlib import Path
import sys
def change_directory(path):
if isinstance(path, Path):
os.chdir(path)
elif isinstance(path, str):
os.chdir(Path(path))
else:
raise ValueError("Invalid path type")
def get_current_directory():
return Path.cwd()
if __name__ == "__main__":
if len(sys.argv) > 1:
directory = sys.argv[1]
change_directory(directory)
else:
print("Please provide a directory path")
current_directory = get_current_directory()
print(f"Current working directory: {current_directory}")
在这个示例中,我们定义了两个函数:change_directory
和get_current_directory
。change_directory
函数接受路径参数,可以是Path
对象或者字符串,并更改当前工作目录。get_current_directory
函数返回当前工作目录。主程序部分通过命令行参数获取目录路径并更改工作目录,然后输出当前工作目录。
五、错误处理和异常捕获
在实际使用中,可能会遇到一些错误和异常。例如,指定的目录不存在或者没有权限访问。为了提高程序的健壮性,我们需要进行错误处理和异常捕获。
5.1 使用try-except捕获异常
我们可以使用try-except
块捕获异常,并进行相应的处理。
import os
from pathlib import Path
def change_directory(path):
try:
os.chdir(path)
except FileNotFoundError:
print(f"Error: Directory '{path}' not found")
except PermissionError:
print(f"Error: Permission denied for directory '{path}'")
except Exception as e:
print(f"Error: {e}")
def get_current_directory():
return Path.cwd()
if __name__ == "__main__":
directory = input("Enter the directory path: ")
change_directory(directory)
current_directory = get_current_directory()
print(f"Current working directory: {current_directory}")
在这个示例中,我们在change_directory
函数中使用try-except
块捕获异常,并输出相应的错误信息。这样可以避免程序因为未处理的异常而终止。
5.2 使用assert进行路径验证
在更改工作目录之前,我们可以使用assert
语句验证路径是否有效。
import os
from pathlib import Path
def change_directory(path):
assert Path(path).exists(), f"Error: Directory '{path}' does not exist"
assert Path(path).is_dir(), f"Error: Path '{path}' is not a directory"
os.chdir(path)
def get_current_directory():
return Path.cwd()
if __name__ == "__main__":
directory = input("Enter the directory path: ")
change_directory(directory)
current_directory = get_current_directory()
print(f"Current working directory: {current_directory}")
在这个示例中,我们使用assert
语句验证路径是否存在以及是否是一个目录。如果路径无效,程序将抛出AssertionError
并输出相应的错误信息。
六、总结
通过本文,我们详细介绍了Python中如何进入目录下的方法,主要包括使用os
库、pathlib
库和sys
库。我们还展示了如何进行错误处理和异常捕获,确保程序的健壮性。使用os库、使用pathlib库、使用sys库是更改和获取工作目录的有效方法,希望这些内容能够帮助您在实际开发中更好地操作目录和文件。
相关问答FAQs:
如何在Python中更改当前工作目录?
在Python中,可以使用os
模块的chdir()
函数更改当前工作目录。首先,导入os
模块,然后调用os.chdir('目标目录路径')
,这将使程序的工作目录切换到指定的路径。可以使用os.getcwd()
函数来查看当前工作目录。
在Python中如何列出目录中的文件和子目录?
要列出当前目录或指定目录中的文件和子目录,可以使用os.listdir()
函数。传入目标目录的路径作为参数,函数将返回该目录下所有文件和子目录的列表。例如,os.listdir('目标目录路径')
将返回该路径下的所有项目。
如何在Python中创建新目录?
在Python中,可以使用os
模块的mkdir()
函数创建新目录。使用方法是先导入os
模块,然后调用os.mkdir('新目录名称')
。如果需要创建多级目录,可以使用os.makedirs('路径/到/新目录')
,它会递归创建所有不存在的目录。
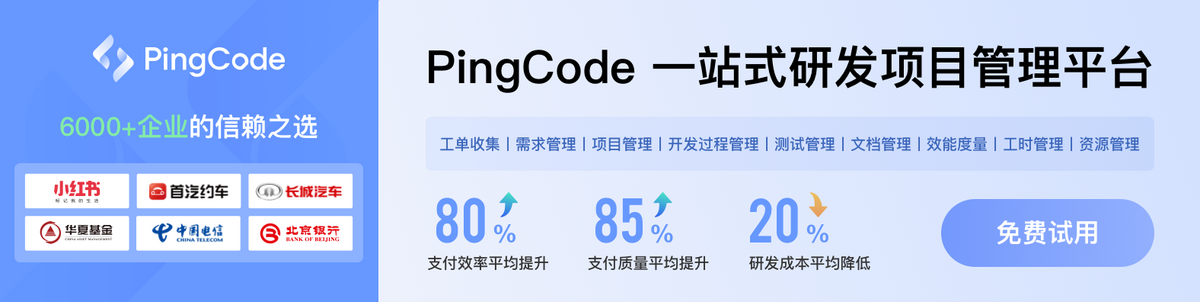