使用Python爬取文件中的数据,可以通过以下几种方式:使用requests库进行HTTP请求、使用BeautifulSoup解析HTML、使用Selenium自动化浏览器操作。这些方法各有优劣,可以根据具体场景选择合适的工具。以下将详细介绍一种常用的方式:使用requests和BeautifulSoup库进行网页爬取。
一、使用requests库进行HTTP请求
- 安装requests库
首先,确保已经安装了requests库。如果没有安装,可以使用pip进行安装:
pip install requests
- 发送HTTP请求并获取响应内容
使用requests库发送HTTP请求,并获取网页的HTML内容:
import requests
url = 'http://example.com'
response = requests.get(url)
html_content = response.content
- 处理响应状态码
在发送请求后,需要检查响应的状态码,以确保请求成功:
if response.status_code == 200:
print('请求成功')
else:
print(f'请求失败,状态码:{response.status_code}')
二、使用BeautifulSoup解析HTML
- 安装BeautifulSoup库
如果没有安装BeautifulSoup库,可以使用pip进行安装:
pip install beautifulsoup4
- 解析HTML内容
使用BeautifulSoup库解析HTML内容:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
- 查找并提取数据
使用BeautifulSoup提供的查找方法,提取所需的数据:
data = soup.find_all('tag_name', class_='class_name')
for item in data:
print(item.text)
以爬取文件中的数据为例,假设要从一个网页中爬取所有的PDF文件链接:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
pdf_links = soup.find_all('a', href=True)
for link in pdf_links:
if link['href'].endswith('.pdf'):
print(link['href'])
else:
print(f'请求失败,状态码:{response.status_code}')
三、使用Selenium自动化浏览器操作
有些网页内容是通过JavaScript动态加载的,requests和BeautifulSoup无法直接获取到,这时可以使用Selenium进行爬取。
- 安装Selenium库和浏览器驱动
pip install selenium
下载浏览器驱动(如ChromeDriver),并将其路径添加到系统环境变量中。
- 使用Selenium打开网页并获取页面内容
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get('http://example.com')
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
- 查找并提取数据
data = soup.find_all('tag_name', class_='class_name')
for item in data:
print(item.text)
driver.quit()
四、解析和存储数据
在爬取到数据后,需要进行解析和存储。可以将数据存储到CSV、Excel或数据库中,具体方法如下:
- 存储到CSV文件
import csv
data = [['name1', 'value1'], ['name2', 'value2']]
with open('data.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Value'])
writer.writerows(data)
- 存储到Excel文件
import pandas as pd
data = {'Name': ['name1', 'name2'], 'Value': ['value1', 'value2']}
df = pd.DataFrame(data)
df.to_excel('data.xlsx', index=False)
- 存储到数据库
import sqlite3
conn = sqlite3.connect('data.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS data
(Name TEXT, Value TEXT)''')
data = [('name1', 'value1'), ('name2', 'value2')]
cursor.executemany('INSERT INTO data VALUES (?, ?)', data)
conn.commit()
conn.close()
五、处理复杂网页结构
有些网页结构复杂,数据嵌套在多个层级内,或者使用JavaScript动态生成内容。针对这种情况,可以结合使用requests、BeautifulSoup和Selenium,以及其他解析库,如lxml等。
- 解析嵌套结构
nested_data = soup.find('div', class_='parent_class')
child_data = nested_data.find_all('span', class_='child_class')
for item in child_data:
print(item.text)
- 处理动态内容
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('http://example.com')
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, 'dynamic_class'))
)
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
六、应对反爬虫机制
有些网站具有反爬虫机制,限制频繁访问或检测爬虫行为。为了应对这些机制,可以采取以下措施:
- 设置请求头
通过设置User-Agent等请求头,模拟真实浏览器访问:
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url, headers=headers)
- 使用代理IP
通过使用代理IP,避免被网站屏蔽:
proxies = {
'http': 'http://proxy_ip:port',
'https': 'http://proxy_ip:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
- 设置请求间隔
通过设置合理的请求间隔,避免频繁访问导致被封禁:
import time
for url in url_list:
response = requests.get(url, headers=headers)
time.sleep(5) # 每次请求间隔5秒
- 使用验证码识别
有些网站会使用验证码来防止爬虫,可以使用第三方验证码识别服务,如打码平台,进行验证码识别:
七、处理异常情况
在爬取数据时,可能会遇到各种异常情况,如网络错误、页面不存在等。需要添加异常处理机制,保证程序的健壮性:
- 捕获网络错误
import requests
from requests.exceptions import RequestException
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
except RequestException as e:
print(f'网络错误:{e}')
- 处理页面不存在
if response.status_code == 404:
print('页面不存在')
- 捕获其他异常
try:
# 解析和处理数据
except Exception as e:
print(f'其他异常:{e}')
八、总结与实践
使用Python爬取文件中的数据,是一个系统工程,需要结合使用requests、BeautifulSoup、Selenium等工具,处理各种复杂的网页结构和反爬虫机制。在实际操作中,需要不断调整和优化爬取策略,确保数据的准确性和完整性。
通过实践,熟练掌握这些工具和方法,可以高效地完成数据爬取任务,并为后续的数据分析和应用提供可靠的数据支持。
相关问答FAQs:
如何用Python从文本文件中提取特定数据?
在Python中,可以使用内置的文件操作功能来读取文本文件。通过逐行读取文件内容,结合字符串处理方法,如split()
、find()
等,可以提取特定的数据。如果数据以结构化格式存储(如CSV、JSON),可以使用相应的库(如csv
、json
)来简化提取过程。
在爬取数据时,如何处理文件的编码问题?
文件的编码方式可能会影响数据的读取。常见的编码格式包括UTF-8和ISO-8859-1。在打开文件时,可以指定encoding
参数,例如open('file.txt', 'r', encoding='utf-8')
。确保使用与文件实际编码相符的编码格式,以避免乱码和读取错误。
有哪些常用的Python库可以帮助爬取和解析数据?
Python提供了多种库来帮助爬取和解析数据。例如,requests
库用于发送HTTP请求获取网页内容,BeautifulSoup
和lxml
则用于解析HTML和XML文档。此外,pandas
库在处理结构化数据时非常强大,它能够方便地读取CSV文件和Excel文件,帮助分析和处理数据。
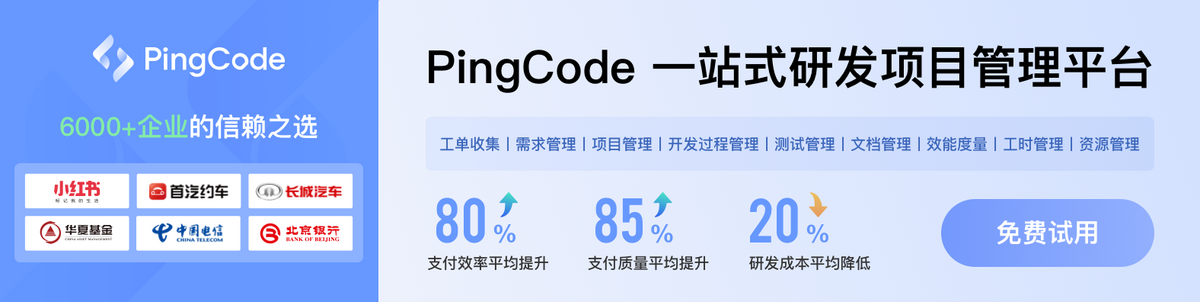