在Python类中,方法调用方法是一个常见的编程场景。方法调用方法的方式包括:直接调用、通过self关键字调用、通过类名调用、通过super()调用,其中最常见和最推荐的方式是通过self关键字调用。这种调用方式不仅直观,而且便于代码的维护和扩展。直接调用意味着在同一个方法中直接调用另一个方法,而不需要任何前缀。这种方式适用于静态方法和类方法。在类方法中,我们通常使用类名来调用方法。在继承和多态的场景下,super()调用是一个强有力的工具。
一、直接调用
直接调用是最简单的方法调用方式。你可以在一个方法中直接调用另一个方法,而无需使用任何前缀。这种方式通常适用于静态方法和类方法。它可以让代码更加简洁和易读。
class MyClass:
def method1(self):
print("Method 1 called")
self.method2()
def method2(self):
print("Method 2 called")
obj = MyClass()
obj.method1()
在上面的例子中,method1
直接调用了method2
,并且由于它们都在同一个类中,调用是直接的。
二、通过self关键字调用
在类的实例方法中,self关键字是必须的。self代表类的实例,使用self可以访问类的属性和方法。这使得代码的可读性和维护性都得到了极大的提升。
class MyClass:
def method1(self):
print("Method 1 called")
self.method2()
def method2(self):
print("Method 2 called")
obj = MyClass()
obj.method1()
在这个例子中,method1
使用self.method2()
来调用method2
。这种方式不仅可以调用方法,还可以访问属性。
三、通过类名调用
在一些特殊情况下,特别是当涉及到继承和多态时,你可能需要通过类名来调用方法。这种方式通常用于类方法和静态方法。
class MyClass:
@classmethod
def method1(cls):
print("Class Method 1 called")
cls.method2()
@classmethod
def method2(cls):
print("Class Method 2 called")
MyClass.method1()
在这个例子中,method1
使用cls.method2()
来调用method2
,其中cls
是类本身。这种方式在类方法中非常常见。
四、通过super()调用
在继承和多态的场景下,super()调用是一个强有力的工具。它允许你调用父类的方法,这对于代码的重用性和扩展性非常有帮助。
class ParentClass:
def method1(self):
print("Parent Method 1 called")
class ChildClass(ParentClass):
def method1(self):
print("Child Method 1 called")
super().method1()
obj = ChildClass()
obj.method1()
在这个例子中,ChildClass
的method1
调用了ParentClass
的method1
,这使得子类可以扩展父类的功能。
五、使用装饰器
装饰器是一种非常强大的工具,允许你在不修改函数定义的情况下扩展其功能。装饰器可以用于方法调用,特别是在需要在调用前后执行某些操作时。
def decorator(func):
def wrapper(*args, kwargs):
print("Before calling the method")
result = func(*args, kwargs)
print("After calling the method")
return result
return wrapper
class MyClass:
@decorator
def method1(self):
print("Method 1 called")
obj = MyClass()
obj.method1()
在这个例子中,decorator
在method1
调用前后打印了一些信息,这使得方法调用更加灵活和可扩展。
六、使用闭包
闭包是一种特殊的函数,它可以捕获并记住其创建时的上下文环境。在类方法调用中,闭包可以用于创建动态方法或属性。
class MyClass:
def __init__(self, value):
self.value = value
def method1(self):
def inner_method():
print(f"Inner method called with value {self.value}")
return inner_method
obj = MyClass(10)
inner = obj.method1()
inner()
在这个例子中,method1
返回了一个闭包inner_method
,这个闭包记住了self.value
的值。
七、使用反射
反射是指在运行时动态地获取类的属性和方法。Python提供了内置的getattr
函数来实现反射,这在某些动态场景下非常有用。
class MyClass:
def method1(self):
print("Method 1 called")
obj = MyClass()
method_name = 'method1'
method = getattr(obj, method_name)
method()
在这个例子中,getattr
动态地获取了method1
方法,并且成功地调用了它。这使得代码在处理未知或动态方法时更加灵活。
八、使用多线程和多进程
在一些高性能或并发场景下,你可能需要在多线程或多进程中调用方法。Python的threading
和multiprocessing
库可以帮助你实现这一点。
import threading
class MyClass:
def method1(self):
print("Method 1 called")
obj = MyClass()
thread = threading.Thread(target=obj.method1)
thread.start()
thread.join()
在这个例子中,method1
在一个单独的线程中被调用,这使得方法调用更加并发和高效。
九、使用异步编程
异步编程是一种高效的I/O操作方式,特别适用于网络请求和文件操作。Python的asyncio
库提供了强大的异步编程支持。
import asyncio
class MyClass:
async def method1(self):
print("Method 1 called")
await self.method2()
async def method2(self):
print("Method 2 called")
obj = MyClass()
asyncio.run(obj.method1())
在这个例子中,method1
和method2
都是异步方法,通过await
关键字实现异步调用。
十、使用元类
元类是创建类的类,它们允许你在类创建时动态地修改类的行为。元类可以用于方法调用,特别是在需要在类创建时注入或修改方法时。
class Meta(type):
def __new__(cls, name, bases, dct):
def method(self):
print("Injected method called")
dct['injected_method'] = method
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
pass
obj = MyClass()
obj.injected_method()
在这个例子中,Meta
元类在创建MyClass
时注入了一个方法injected_method
,并且成功调用了它。
通过上述多种方式,你可以根据具体的场景和需求选择合适的方法调用方式。每种方式都有其优缺点和适用场景,合理使用可以使代码更加高效、可读和易维护。
相关问答FAQs:
在Python类中如何嵌套调用方法?
在Python中,类的一个方法可以直接调用同一类中的另一个方法。只需在方法内部使用self.方法名()
的形式即可。例如,定义一个类时,可以在一个方法中调用另一个方法来实现更复杂的逻辑。
如何在类的构造函数中调用其他方法?
构造函数__init__
可以用来初始化对象的属性,同时也可以在其中调用其他方法以执行额外的设置或计算。在构造函数中使用self.方法名()
来实现,确保在对象创建时就能完成必要的初始化工作。
如果方法需要传递参数,如何在调用时实现?
当调用一个需要参数的方法时,可以在调用时传入相应的参数。在类内的方法调用中,依然使用self.方法名(参数)
的格式来完成。例如,可以创建一个方法接收参数并返回结果,然后在另一个方法中调用此方法并传入所需的参数。
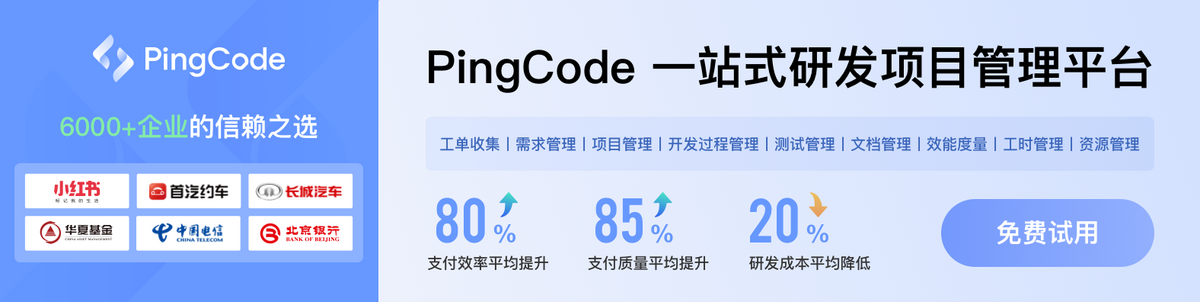