如何利用Python爬取今天天气
要利用Python爬取今天天气,我们可以使用以下几个步骤:选择合适的API、安装必要的库、编写爬虫代码、处理和展示数据。选择合适的API、安装必要的库、编写爬虫代码、处理和展示数据。首先,我们需要选择一个提供天气数据的API服务,例如OpenWeatherMap或WeatherAPI,它们提供了丰富的天气数据接口。接下来,我们需要安装Python的requests库来发送HTTP请求,并使用json库来解析数据。然后,我们编写代码来从API获取天气数据,最后处理并展示这些数据。下面是详细描述:
选择合适的API
选择一个好的API是成功爬取天气数据的关键。OpenWeatherMap和WeatherAPI是两个非常流行且功能强大的天气数据API。它们提供了当前天气、预报、历史数据等丰富的接口,并且有良好的文档支持。
- OpenWeatherMap:这是一个广泛使用的天气数据API,它提供了免费的和付费的服务。免费服务的限制是每分钟调用次数受限,并且数据有一定的延迟。
- WeatherAPI:这个API也提供了免费的和付费的服务。它的数据更加实时,接口更加简洁,适合快速开发。
安装必要的库
在开始编写代码之前,我们需要安装一些必要的库。requests库是用来发送HTTP请求的,json库是用来解析JSON数据的。我们可以使用pip来安装这些库:
pip install requests
编写爬虫代码
接下来,我们开始编写爬虫代码。以下是一个使用OpenWeatherMap API的示例代码:
import requests
import json
def get_weather(city):
api_key = "your_api_key_here"
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "q=" + city + "&appid=" + api_key
response = requests.get(complete_url)
data = response.json()
if data["cod"] != "404":
main = data["main"]
weather = data["weather"][0]
temp = main["temp"]
pressure = main["pressure"]
humidity = main["humidity"]
description = weather["description"]
weather_info = {
"Temperature": temp,
"Pressure": pressure,
"Humidity": humidity,
"Description": description
}
return weather_info
else:
return None
city = "London"
weather_info = get_weather(city)
if weather_info:
print("Temperature: " + str(weather_info["Temperature"]))
print("Pressure: " + str(weather_info["Pressure"]))
print("Humidity: " + str(weather_info["Humidity"]))
print("Description: " + weather_info["Description"])
else:
print("City Not Found!")
在这个示例代码中,我们定义了一个函数get_weather,它接受一个城市名称作为参数,并返回该城市的天气信息。我们使用requests库发送HTTP请求,并使用json库解析返回的数据。最后,我们将天气信息打印出来。
处理和展示数据
获取到天气数据后,我们需要对数据进行处理和展示。我们可以将数据存储到数据库中,或者通过网页、命令行界面等方式展示出来。以下是一个简单的展示示例:
def display_weather_info(weather_info):
print("Temperature: " + str(weather_info["Temperature"]) + " K")
print("Pressure: " + str(weather_info["Pressure"]) + " hPa")
print("Humidity: " + str(weather_info["Humidity"]) + " %")
print("Description: " + weather_info["Description"])
if weather_info:
display_weather_info(weather_info)
else:
print("City Not Found!")
这个示例代码定义了一个函数display_weather_info,它接受一个天气信息字典作为参数,并将信息打印出来。我们可以根据需要对数据进行进一步处理和展示。
小结
通过以上步骤,我们可以成功地利用Python爬取今天天气。选择合适的API、安装必要的库、编写爬虫代码、处理和展示数据是关键步骤。希望这篇文章对你有所帮助。
相关问答FAQs:
如何使用Python获取天气数据?
可以使用Python的requests库来发送HTTP请求,从天气API获取天气数据。常用的天气API包括OpenWeatherMap和Weather API等。获取到的数据通常是JSON格式,通过Python的json库进行解析后,就可以提取出所需的天气信息。
使用Python爬虫时需要注意哪些问题?
在爬取天气数据时,需遵循网站的robots.txt文件规定,以确保遵守网站的爬虫政策。此外,合理设置请求间隔时间,防止对服务器造成过大压力,避免被封IP。
可以用哪些Python库来辅助爬取天气信息?
除了requests库外,Beautiful Soup和lxml是解析HTML和XML文档的常用库,适合处理从网站上直接抓取的天气信息。如果使用API获取天气数据,则可以使用json库来处理响应数据。其他如pandas库也可以帮助处理和分析数据。
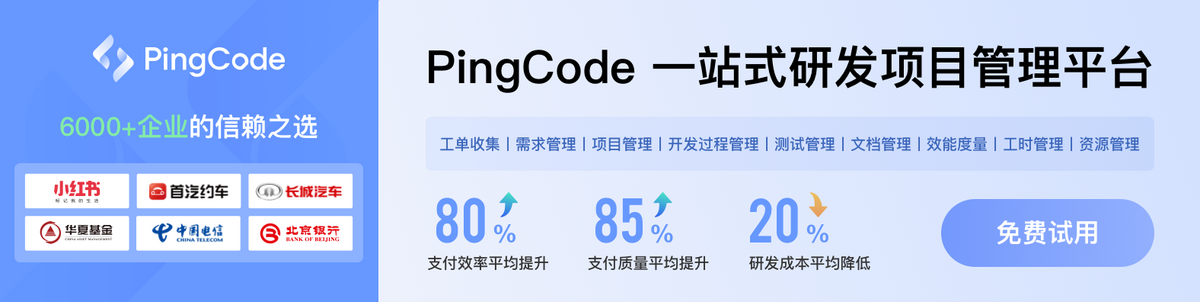