在Python中编写温度转换程序可以通过几个简单的步骤来实现。我们可以编写一个函数来转换温度单位,例如从摄氏度转换为华氏度,或从华氏度转换为摄氏度。以下是如何实现这些转换的详细步骤和代码示例。
摄氏度到华氏度转换公式:
摄氏温度(°C) 转换为 华氏温度(°F) 的公式是:°F = °C * 9/5 + 32
华氏度到摄氏度转换公式:
华氏温度(°F) 转换为 摄氏温度(°C) 的公式是:°C = (°F - 32) * 5/9
一、基本温度转换功能实现
1.1、摄氏度到华氏度
我们首先编写一个函数来将摄氏度转换为华氏度。
def celsius_to_fahrenheit(celsius):
"""Convert Celsius to Fahrenheit."""
return celsius * 9/5 + 32
1.2、华氏度到摄氏度
同样,我们也可以编写一个函数来将华氏度转换为摄氏度。
def fahrenheit_to_celsius(fahrenheit):
"""Convert Fahrenheit to Celsius."""
return (fahrenheit - 32) * 5/9
二、使用示例
2.1、调用转换函数
我们可以通过调用这些函数来进行温度转换。以下是一些示例代码:
# Convert 100 Celsius to Fahrenheit
celsius_temp = 100
fahrenheit_temp = celsius_to_fahrenheit(celsius_temp)
print(f"{celsius_temp}°C is equal to {fahrenheit_temp}°F")
Convert 212 Fahrenheit to Celsius
fahrenheit_temp = 212
celsius_temp = fahrenheit_to_celsius(fahrenheit_temp)
print(f"{fahrenheit_temp}°F is equal to {celsius_temp}°C")
三、扩展功能
3.1、增加输入验证
为了使我们的程序更健壮,我们可以增加输入验证,确保输入的温度值是数字。
def celsius_to_fahrenheit(celsius):
"""Convert Celsius to Fahrenheit with input validation."""
try:
celsius = float(celsius)
return celsius * 9/5 + 32
except ValueError:
return "Invalid input, please enter a numeric value."
def fahrenheit_to_celsius(fahrenheit):
"""Convert Fahrenheit to Celsius with input validation."""
try:
fahrenheit = float(fahrenheit)
return (fahrenheit - 32) * 5/9
except ValueError:
return "Invalid input, please enter a numeric value."
3.2、增加用户交互
我们可以通过增加用户交互来提高程序的可用性。以下是一个示例:
def main():
while True:
choice = input("Choose conversion type:\n1. Celsius to Fahrenheit\n2. Fahrenheit to Celsius\n3. Exit\nEnter choice (1/2/3): ")
if choice == '1':
celsius = input("Enter temperature in Celsius: ")
print(f"{celsius}°C is equal to {celsius_to_fahrenheit(celsius)}°F")
elif choice == '2':
fahrenheit = input("Enter temperature in Fahrenheit: ")
print(f"{fahrenheit}°F is equal to {fahrenheit_to_celsius(fahrenheit)}°C")
elif choice == '3':
break
else:
print("Invalid choice, please try again.")
if __name__ == "__main__":
main()
四、温度转换的应用场景
温度转换在许多领域中都有广泛的应用,包括但不限于:
4.1、气象学
在气象学中,温度数据可能以不同的单位记录和报告。科学家和气象学家需要在摄氏度和华氏度之间进行转换,以便进行数据分析和报告。
4.2、医学
在医学领域,温度测量对于诊断和治疗至关重要。不同国家和地区使用的温度单位不同,医生和护士需要在摄氏度和华氏度之间进行转换,以确保准确的体温测量和记录。
4.3、工业和工程
在工业和工程领域,温度控制和监测是关键因素。工程师和技术人员需要在摄氏度和华氏度之间进行转换,以确保设备和工艺的正常运行。
五、总结
通过本文,我们学习了如何使用Python编写一个简单的温度转换程序,包括摄氏度到华氏度和华氏度到摄氏度的转换。我们还探讨了如何通过增加输入验证和用户交互来提高程序的健壮性和可用性。最后,我们了解了温度转换在气象学、医学和工业工程等领域的应用场景。
希望这篇文章对您有所帮助,使您能够更好地理解和实现温度转换程序。如果您有任何问题或建议,欢迎在评论区留言讨论。
相关问答FAQs:
如何使用Python将华氏温度转换为摄氏温度?
要将华氏温度转换为摄氏温度,可以使用以下公式:C = (F – 32) * 5/9。在Python中,可以通过定义一个函数来实现这个转换。例如:
def fahrenheit_to_celsius(f):
return (f - 32) * 5/9
# 示例
fahrenheit = 100
celsius = fahrenheit_to_celsius(fahrenheit)
print(f"{fahrenheit} 华氏度等于 {celsius:.2f} 摄氏度")
运行这段代码后,你将得到华氏温度转换成的摄氏温度。
如何实现摄氏温度到华氏温度的转换?
摄氏温度转换为华氏温度的公式是 F = C * 9/5 + 32。在Python中同样可以通过定义一个函数来实现。示例代码如下:
def celsius_to_fahrenheit(c):
return c * 9/5 + 32
# 示例
celsius = 37
fahrenheit = celsius_to_fahrenheit(celsius)
print(f"{celsius} 摄氏度等于 {fahrenheit:.2f} 华氏度")
通过这段代码,可以轻松实现摄氏度到华氏度的转换。
如何在Python中处理用户输入的温度转换?
可以使用input()
函数接收用户输入的温度值,并根据用户选择的转换方式进行相应的转换。下面是一个简单的示例:
def temperature_converter():
choice = input("选择转换类型(1: 摄氏转华氏, 2: 华氏转摄氏): ")
temp = float(input("请输入温度: "))
if choice == '1':
print(f"{temp} 摄氏度等于 {celsius_to_fahrenheit(temp):.2f} 华氏度")
elif choice == '2':
print(f"{temp} 华氏度等于 {fahrenheit_to_celsius(temp):.2f} 摄氏度")
else:
print("无效选择,请选择 1 或 2")
temperature_converter()
运行后,用户可以选择转换类型并输入温度,程序将输出转换结果。
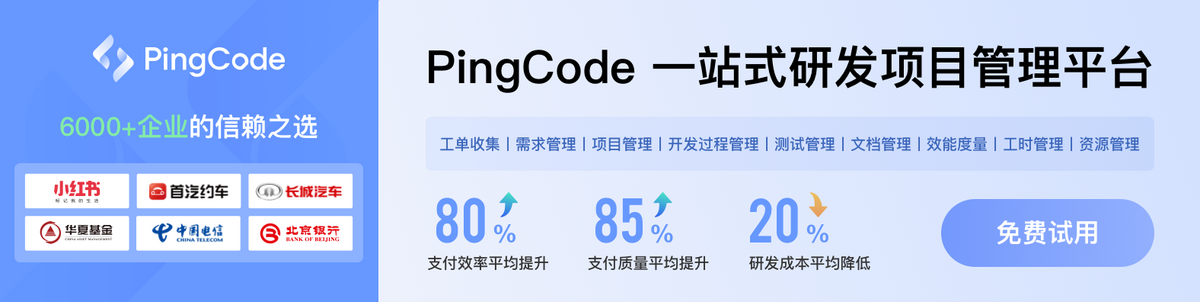