在Python中使用Qt执行鼠标按下事件的方法有:继承QWidget、重写mousePressEvent、使用QMouseEvent。 其中,最常用的方法是重写mousePressEvent
函数来捕捉和处理鼠标按下事件。下面将详细讲解如何实现这一点。
一、继承QWidget
在Qt中,所有的窗口部件都是从QWidget类继承而来的。为了捕捉和处理鼠标事件,我们通常需要创建一个自定义的窗口部件类,并从QWidget类继承。
from PyQt5.QtWidgets import QWidget, QApplication
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QMouseEvent
class MyWidget(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('Mouse Press Event Example')
self.setGeometry(100, 100, 400, 300)
def mousePressEvent(self, event: QMouseEvent):
if event.button() == Qt.LeftButton:
print('Left mouse button pressed at:', event.pos())
elif event.button() == Qt.RightButton:
print('Right mouse button pressed at:', event.pos())
elif event.button() == Qt.MiddleButton:
print('Middle mouse button pressed at:', event.pos())
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
widget = MyWidget()
widget.show()
sys.exit(app.exec_())
在这个示例中,我们创建了一个名为MyWidget
的自定义窗口部件类,并重写了mousePressEvent
方法。这个方法在鼠标按下时会被调用,并根据按下的鼠标按钮类型打印相应的信息。
二、重写mousePressEvent
重写mousePressEvent
方法是捕捉和处理鼠标按下事件的关键。在这个方法中,我们可以使用QMouseEvent
对象来获取关于鼠标事件的详细信息,例如按下的鼠标按钮、鼠标位置等。
def mousePressEvent(self, event: QMouseEvent):
if event.button() == Qt.LeftButton:
print('Left mouse button pressed at:', event.pos())
elif event.button() == Qt.RightButton:
print('Right mouse button pressed at:', event.pos())
elif event.button() == Qt.MiddleButton:
print('Middle mouse button pressed at:', event.pos())
在这个方法中,我们使用event.button()
来确定按下的鼠标按钮类型,并使用event.pos()
来获取鼠标按下时的位置。
三、使用QMouseEvent
QMouseEvent
类提供了关于鼠标事件的详细信息。在mousePressEvent
方法中,我们可以使用QMouseEvent
对象来获取鼠标事件的各种属性,例如按下的鼠标按钮、鼠标位置、修饰键等。
def mousePressEvent(self, event: QMouseEvent):
if event.button() == Qt.LeftButton:
print('Left mouse button pressed at:', event.pos())
print('Modifiers:', event.modifiers())
print('Global position:', event.globalPos())
elif event.button() == Qt.RightButton:
print('Right mouse button pressed at:', event.pos())
elif event.button() == Qt.MiddleButton:
print('Middle mouse button pressed at:', event.pos())
在这个方法中,我们使用event.modifiers()
来获取修饰键(例如Ctrl、Shift、Alt等),使用event.globalPos()
来获取鼠标按下时的全局位置。
四、捕捉更多鼠标事件
除了mousePressEvent
方法外,Qt还提供了其他与鼠标事件相关的方法,例如mouseReleaseEvent
、mouseDoubleClickEvent
、mouseMoveEvent
等。我们可以重写这些方法来捕捉和处理更多的鼠标事件。
def mouseReleaseEvent(self, event: QMouseEvent):
print('Mouse button released at:', event.pos())
def mouseDoubleClickEvent(self, event: QMouseEvent):
print('Mouse button double clicked at:', event.pos())
def mouseMoveEvent(self, event: QMouseEvent):
print('Mouse moved to:', event.pos())
在这些方法中,我们可以使用QMouseEvent
对象来获取鼠标事件的详细信息,并根据需要进行处理。
五、总结
在Python中使用Qt执行鼠标按下事件的方法包括继承QWidget、重写mousePressEvent、使用QMouseEvent等。通过重写mousePressEvent
方法,我们可以捕捉和处理鼠标按下事件,并使用QMouseEvent
对象获取关于鼠标事件的详细信息。此外,Qt还提供了其他与鼠标事件相关的方法,例如mouseReleaseEvent
、mouseDoubleClickEvent
、mouseMoveEvent
等,我们可以重写这些方法来捕捉和处理更多的鼠标事件。在实际开发中,我们可以根据需要选择合适的方法来处理鼠标事件。
相关问答FAQs:
如何在Python中使用Qt捕捉鼠标按下事件?
在Python中使用Qt库时,可以通过重写mousePressEvent
方法来捕捉鼠标按下事件。您可以在自定义的QWidget类中实现该方法,获取鼠标按下的位置及其他相关信息,从而执行相应的操作。例如:
class MyWidget(QtWidgets.QWidget):
def mousePressEvent(self, event):
if event.button() == QtCore.Qt.LeftButton:
print(f"Mouse pressed at: {event.pos()}")
Qt支持的鼠标事件有哪些?
Qt提供了多种鼠标事件,包括mousePressEvent
、mouseReleaseEvent
和mouseMoveEvent
。这些事件允许开发者处理鼠标按下、释放和移动的情况。通过对这些事件的重写,您可以自定义应用程序的交互方式。
在Qt中如何区分不同的鼠标按键?
在Qt中,您可以通过QMouseEvent
对象的button()
方法来判断哪个鼠标按键被按下。常见的鼠标按键包括左键、右键和中间滚轮键。使用这些方法可以帮助您实现复杂的鼠标交互逻辑。例如:
if event.button() == QtCore.Qt.RightButton:
print("Right mouse button pressed.")
如何在Qt中实现鼠标按下事件的延迟响应?
如果希望在鼠标按下后延迟执行某个操作,可以使用QTimer
。在mousePressEvent
中启动一个定时器,并在定时器到期后执行所需的操作。这种方法可以避免在快速点击时触发多个事件。例如:
self.timer = QtCore.QTimer()
self.timer.timeout.connect(self.performAction)
self.timer.setSingleShot(True)
def mousePressEvent(self, event):
if event.button() == QtCore.Qt.LeftButton:
self.timer.start(1000) # 延迟1秒
通过以上方式,您可以灵活地处理Qt中的鼠标按下事件,增强用户体验。
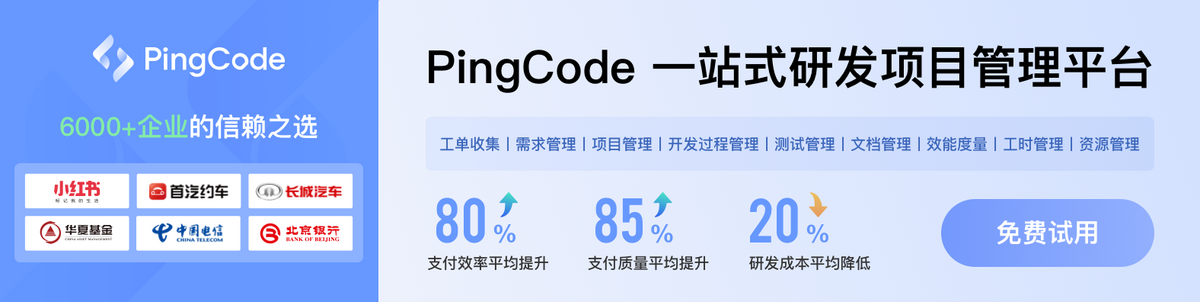