在Python中屏蔽一段代码的方法包括使用注释、条件语句和装饰器。 最常见的方法是使用注释符号将代码段注释掉,Python使用井号(#)作为单行注释符号。另一种方法是使用条件语句,如if False:
,将代码段放在条件语句块内,使其不会被执行。还可以使用装饰器来屏蔽函数的执行。以下是详细描述其中一种方法:
使用注释符号:
在Python中,注释符号是最常用来屏蔽代码的一种方法。你可以在你不希望执行的代码前加上井号(#),这样Python解释器在执行代码时会忽略这些被注释掉的行。例如:
# print("This line will not be executed")
print("This line will be executed")
通过注释符号,可以方便地屏蔽掉单行或多行代码,而不需要修改代码的结构。对于多行注释,可以在每一行前添加井号,或者使用三重引号将代码段包围起来。
"""
print("This line will not be executed")
print("This line will not be executed either")
"""
print("This line will be executed")
接下来我们将详细探讨Python中屏蔽代码的不同方法及其应用。
一、使用注释符号
注释符号是屏蔽代码最直观和常用的方法。通过在代码前加上井号(#),可以将整行代码注释掉,使其不被执行。
单行注释
单行注释适用于需要临时屏蔽单行代码的情况。只需在代码行前加上井号即可。
# print("This line is commented out and will not be executed")
print("This line will be executed")
多行注释
虽然Python没有多行注释的专用语法,但可以通过在每一行前添加井号,或者使用三重引号将代码段包围起来,达到类似的效果。
# print("This line is commented out and will not be executed")
print("This line is also commented out")
print("This line will be executed")
使用三重引号
"""
print("This code block is commented out")
print("This code block is also commented out")
"""
print("This line will be executed")
二、使用条件语句
条件语句可以用于屏蔽某段代码,而无需删除代码或修改其结构。使用if False:
语句可以将代码放在条件块内,使其不会被执行。
使用if False:
将代码段放在if False:
条件块内,这样代码永远不会被执行。
if False:
print("This code will not be executed")
print("This code will be executed")
使用变量控制
可以使用布尔变量控制代码的执行。通过改变变量的值,可以方便地启用或禁用某段代码。
execute_code = False
if execute_code:
print("This code will not be executed")
print("This code will be executed")
三、使用装饰器
装饰器是一种高级的Python特性,通常用于修改函数的行为。可以创建一个装饰器来屏蔽函数的执行。
创建装饰器
首先,创建一个装饰器函数,该函数接受一个函数作为参数,并返回一个新的函数。新的函数在调用时不执行原函数。
def block_execution(func):
def wrapper(*args, kwargs):
print(f"Function {func.__name__} is blocked and will not be executed")
return None
return wrapper
使用装饰器
在需要屏蔽的函数前加上装饰器。
@block_execution
def some_function():
print("This function will not be executed")
some_function()
print("This line will be executed")
通过使用装饰器,可以方便地屏蔽函数的执行,同时保留其定义和调用。
四、使用上下文管理器
上下文管理器是一种用于管理资源的高级特性。可以创建一个上下文管理器来屏蔽代码块的执行。
创建上下文管理器
使用contextlib
模块可以方便地创建上下文管理器。
import contextlib
@contextlib.contextmanager
def block_code():
print("Entering blocked code section")
yield
print("Exiting blocked code section")
使用上下文管理器
在需要屏蔽的代码段前加上上下文管理器。
with block_code():
print("This code will be executed but can be blocked")
print("This line will be executed")
上下文管理器提供了一种优雅的方法来管理代码块的执行,可以根据需要屏蔽或启用代码段。
五、使用异常处理
异常处理可以用于屏蔽潜在的错误代码段。通过捕获和忽略异常,可以防止代码的执行中断。
使用try-except
块
将可能引发异常的代码放在try
块内,并在except
块中处理异常。
try:
print("This code will be executed")
raise Exception("An error occurred")
print("This code will not be executed")
except Exception as e:
print(f"Exception caught: {e}")
print("This line will be executed")
通过异常处理,可以屏蔽有风险的代码,同时保证程序的正常运行。
六、使用函数封装
将需要屏蔽的代码封装在函数内,通过控制函数的调用来管理代码的执行。
定义函数
将代码段封装在一个函数内。
def some_function():
print("This function contains the code to be executed or blocked")
控制函数调用
通过条件语句或变量控制函数的调用。
execute_code = False
if execute_code:
some_function()
print("This line will be executed")
函数封装提供了一种结构化的方法来管理代码的执行,可以灵活地启用或禁用特定代码段。
七、使用模块管理
将需要屏蔽的代码放在单独的模块中,通过控制模块的导入来管理代码的执行。
创建模块
将代码段保存到一个单独的Python文件中,例如module.py
。
# module.py
def some_function():
print("This function contains the code to be executed or blocked")
控制模块导入
通过条件语句或变量控制模块的导入。
execute_code = False
if execute_code:
import module
module.some_function()
print("This line will be executed")
模块管理提供了一种模块化的方法来管理代码的执行,可以方便地启用或禁用整个模块的代码。
八、使用环境变量
通过环境变量控制代码的执行,可以在运行时动态配置代码的启用或禁用。
设置环境变量
在操作系统中设置环境变量。例如,在Linux或macOS中,可以使用export
命令。
export EXECUTE_CODE=True
读取环境变量
在代码中读取环境变量的值,并根据其值控制代码的执行。
import os
execute_code = os.getenv('EXECUTE_CODE') == 'True'
if execute_code:
print("This code will be executed based on environment variable")
else:
print("This code will not be executed based on environment variable")
环境变量提供了一种灵活的方法来控制代码的执行,适用于需要在不同运行环境下动态配置的情况。
九、使用配置文件
通过配置文件控制代码的执行,可以在运行时动态配置代码的启用或禁用。
创建配置文件
将配置信息保存到一个文件中,例如config.ini
。
[settings]
execute_code = True
读取配置文件
在代码中读取配置文件的值,并根据其值控制代码的执行。
import configparser
config = configparser.ConfigParser()
config.read('config.ini')
execute_code = config.getboolean('settings', 'execute_code')
if execute_code:
print("This code will be executed based on configuration file")
else:
print("This code will not be executed based on configuration file")
配置文件提供了一种外部化的方法来管理代码的执行,可以方便地在不同运行环境下动态配置。
十、使用日志记录
通过日志记录控制代码的执行,可以在运行时动态配置代码的启用或禁用,并记录代码的执行情况。
配置日志记录
使用logging
模块配置日志记录。
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
logger.info("This code will be executed based on logging configuration")
控制日志记录
通过日志级别控制代码的执行。
if logger.isEnabledFor(logging.INFO):
print("This code will be executed based on logging configuration")
else:
print("This code will not be executed based on logging configuration")
日志记录提供了一种灵活的方法来控制代码的执行,并记录代码的执行情况,适用于需要在不同运行环境下动态配置的情况。
总结
在Python中屏蔽代码的方法有很多种,包括使用注释符号、条件语句、装饰器、上下文管理器、异常处理、函数封装、模块管理、环境变量、配置文件和日志记录。每种方法都有其优点和适用场景,可以根据具体需求选择合适的方法。通过合理使用这些方法,可以灵活地管理代码的执行,提高代码的可维护性和可读性。
相关问答FAQs:
如何在Python中注释掉一段代码?
在Python中,可以使用井号(#)来注释单行代码。如果想要注释多行代码,可以在每行前加上#。另一种常见的方法是使用三重引号('''或""")包裹代码块,这样Python解释器就会忽略这些内容。
使用IDE或文本编辑器屏蔽代码的最佳方法是什么?
很多集成开发环境(IDE)和文本编辑器提供了快捷键来快速注释或取消注释代码。例如,在VS Code中,可以选中代码块后使用Ctrl+/(Windows)或Cmd+/(Mac)来快速添加或删除注释。
屏蔽代码是否会影响程序的性能?
注释掉的代码不会被执行,因此不会对程序的性能产生影响。注释仅用于代码的可读性和维护性,帮助开发人员理解代码的功能和逻辑。适当的注释可以提高团队协作的效率。
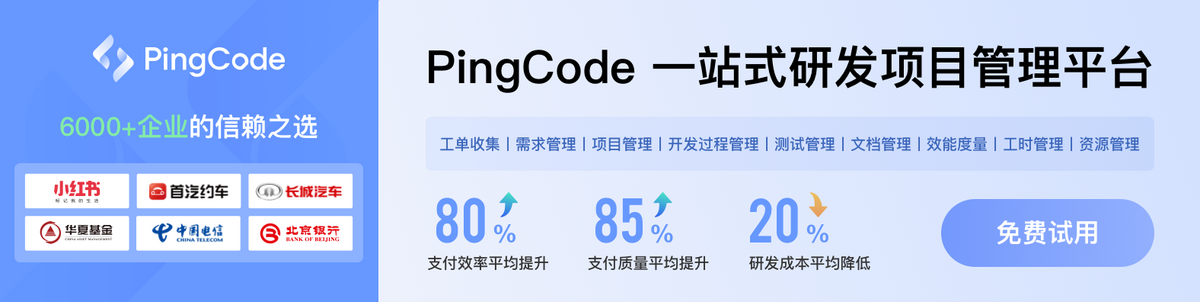