在Python中不区分大小写的方法有很多种,比如:使用lower()
方法、使用casefold()
方法、使用正则表达式等。常用的方法是将字符串转换为小写或大写、使用casefold()
方法处理Unicode字符串、使用正则表达式忽略大小写。其中,casefold()
方法是Python 3.3之后引入的,它专门用来处理Unicode字符串,能更彻底地忽略大小写。
一、使用lower()
或upper()
方法
Python提供了lower()
和upper()
两个方法来处理字符串的大小写。lower()
方法将字符串中的所有字符转换为小写,而upper()
方法则将所有字符转换为大写。以下是具体的使用方法:
string1 = "Hello World"
string2 = "hello world"
if string1.lower() == string2.lower():
print("The strings are equal, ignoring case.")
else:
print("The strings are not equal.")
在这个例子中,string1
和string2
在转换为小写后是相等的,所以输出会是“The strings are equal, ignoring case.
”。
二、使用casefold()
方法处理Unicode字符串
casefold()
方法类似于lower()
方法,但是它对某些特殊字符的处理更加彻底。特别是在处理德语等含有特殊字符的语言时,casefold()
表现更好。以下是使用casefold()
的方法:
string1 = "Straße"
string2 = "strasse"
if string1.casefold() == string2.casefold():
print("The strings are equal, ignoring case.")
else:
print("The strings are not equal.")
在这个例子中,string1
和string2
在转换为小写后是相等的,所以输出会是“The strings are equal, ignoring case.
”。
三、使用正则表达式忽略大小写
正则表达式提供了一个名为re.IGNORECASE
的标志,可以在匹配时忽略大小写。以下是具体的使用方法:
import re
string1 = "Hello World"
pattern = "hello world"
if re.match(pattern, string1, re.IGNORECASE):
print("The strings match, ignoring case.")
else:
print("The strings do not match.")
在这个例子中,string1
和pattern
在匹配时忽略了大小写,所以输出会是“The strings match, ignoring case.
”。
四、比较字典键和值时忽略大小写
在处理字典时,有时需要忽略键和值的大小写。可以通过将所有键和值转换为小写或大写来实现这一点:
dict1 = {'Key1': 'Value1', 'Key2': 'Value2'}
dict2 = {'key1': 'value1', 'key2': 'value2'}
def case_insensitive_dict_comparison(d1, d2):
d1_lower = {k.lower(): v.lower() for k, v in d1.items()}
d2_lower = {k.lower(): v.lower() for k, v in d2.items()}
return d1_lower == d2_lower
if case_insensitive_dict_comparison(dict1, dict2):
print("The dictionaries are equal, ignoring case.")
else:
print("The dictionaries are not equal.")
在这个例子中,dict1
和dict2
在转换为小写后是相等的,所以输出会是“The dictionaries are equal, ignoring case.
”。
五、处理文件内容时忽略大小写
在处理文件内容时,有时需要忽略大小写。可以通过读取文件内容并将其转换为小写或大写来实现这一点:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2:
content1 = file1.read().lower()
content2 = file2.read().lower()
if content1 == content2:
print("The file contents are equal, ignoring case.")
else:
print("The file contents are not equal.")
在这个例子中,file1.txt
和file2.txt
的内容在转换为小写后是相等的,所以输出会是“The file contents are equal, ignoring case.
”。
六、总结
在Python中,有多种方法可以实现不区分大小写的操作。常用的方法包括:将字符串转换为小写或大写、使用casefold()
方法处理Unicode字符串、使用正则表达式忽略大小写等。选择合适的方法取决于具体的应用场景和需求。通过合理使用这些方法,可以有效地处理字符串、字典、文件内容等不同类型的数据,忽略大小写。
相关问答FAQs:
如何在Python中实现不区分大小写的字符串比较?
在Python中,可以使用str.lower()
或str.upper()
方法将字符串转换为小写或大写,从而实现不区分大小写的比较。例如,可以将两个字符串都转换为小写后进行比较:if str1.lower() == str2.lower():
。这样,无论原始字符串的大小写如何,比较结果都将一致。
在处理字典时,如何确保键不区分大小写?
可以通过创建一个自定义的字典类,继承自dict
,并重写__setitem__
和__getitem__
方法,将所有的键转换为统一的大小写形式。这样在插入和检索键时均可实现不区分大小写。例如,键可以统一转换为小写:self.data[key.lower()] = value
。
有哪些内置方法可以帮助处理不区分大小写的字符串操作?
Python提供了一些内置字符串方法,如str.casefold()
、str.lower()
和str.upper()
,这些方法可以帮助进行不区分大小写的字符串处理。casefold()
方法比lower()
更加强大,适用于多语言文本的比较,因为它能处理一些特定语言的字符。使用这些方法,可以轻松地实现字符串的规范化操作。
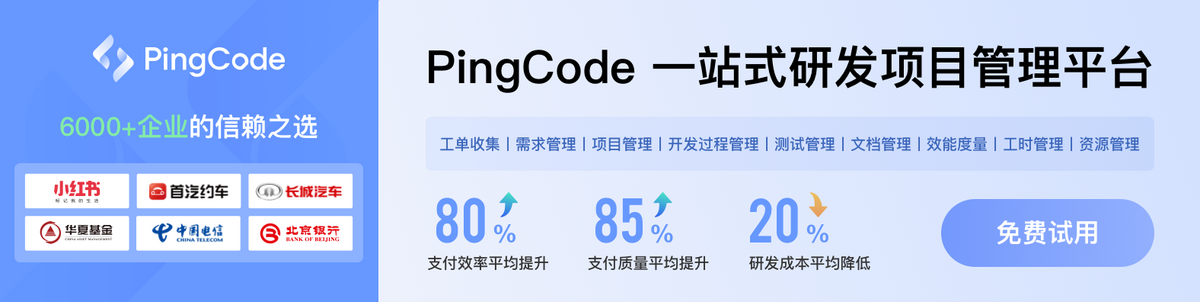