遗传算法(Genetic Algorithm, GA)是一种基于自然选择和遗传机制的优化算法,常用于解决复杂的优化和搜索问题。使用Python实现遗传算法优化样本的核心步骤包括初始化种群、选择、交叉、变异和适应度评估。本文将详细介绍如何使用Python实现遗传算法优化样本,并提供相关代码示例和解释。
一、初始化种群
在遗传算法中,种群是由多个个体组成的,每个个体代表一个可能的解决方案。种群的初始化是指创建一定数量的个体,并为每个个体随机生成一组基因(变量)。
import random
def initialize_population(pop_size, gene_length):
population = []
for _ in range(pop_size):
individual = [random.randint(0, 1) for _ in range(gene_length)]
population.append(individual)
return population
pop_size = 10 # 种群大小
gene_length = 5 # 基因长度
population = initialize_population(pop_size, gene_length)
print("初始种群:", population)
解释: 上述代码定义了initialize_population
函数,用于初始化种群。pop_size
表示种群大小,gene_length
表示每个个体的基因长度。每个个体的基因是一个由0和1组成的列表。
二、适应度评估
适应度函数用于评估每个个体的优劣程度。适应度值越高,个体的质量越好。在实际应用中,适应度函数通常与问题的目标函数相关。
def fitness_function(individual):
return sum(individual) # 简单的适应度函数,基因和越大越好
fitness_values = [fitness_function(ind) for ind in population]
print("适应度值:", fitness_values)
解释: 上述代码定义了fitness_function
函数,用于计算个体的适应度值。在这个示例中,适应度函数为个体基因的和,基因和越大,适应度值越高。
三、选择
选择操作用于从当前种群中选择出适应度较高的个体,作为下一代的父母。常用的选择方法包括轮盘赌选择、锦标赛选择等。
def selection(population, fitness_values):
selected = []
total_fitness = sum(fitness_values)
for _ in range(len(population)):
pick = random.uniform(0, total_fitness)
current = 0
for ind, fit in zip(population, fitness_values):
current += fit
if current > pick:
selected.append(ind)
break
return selected
selected_population = selection(population, fitness_values)
print("选择后的种群:", selected_population)
解释: 上述代码定义了selection
函数,采用轮盘赌选择方法从当前种群中选择个体。个体被选择的概率与其适应度值成正比。
四、交叉
交叉操作用于将两个父母个体的基因组合在一起,生成新的子代个体。常用的交叉方法包括单点交叉、两点交叉等。
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
new_population = []
for i in range(0, len(selected_population), 2):
parent1 = selected_population[i]
parent2 = selected_population[i + 1]
child1, child2 = crossover(parent1, parent2)
new_population.extend([child1, child2])
print("交叉后的种群:", new_population)
解释: 上述代码定义了crossover
函数,采用单点交叉方法生成新的子代个体。new_population
为交叉后的新种群。
五、变异
变异操作用于随机改变个体的基因值,以增加种群的多样性,防止算法陷入局部最优解。常用的变异方法包括单点变异、多点变异等。
def mutation(individual, mutation_rate):
for i in range(len(individual)):
if random.random() < mutation_rate:
individual[i] = 1 - individual[i]
return individual
mutation_rate = 0.01 # 变异率
mutated_population = [mutation(ind, mutation_rate) for ind in new_population]
print("变异后的种群:", mutated_population)
解释: 上述代码定义了mutation
函数,采用单点变异方法随机改变个体的基因值。mutation_rate
表示变异率,mutated_population
为变异后的新种群。
六、完整的遗传算法实现
将上述各个步骤整合在一起,形成完整的遗传算法实现。
import random
初始化种群
def initialize_population(pop_size, gene_length):
population = []
for _ in range(pop_size):
individual = [random.randint(0, 1) for _ in range(gene_length)]
population.append(individual)
return population
适应度函数
def fitness_function(individual):
return sum(individual)
选择操作
def selection(population, fitness_values):
selected = []
total_fitness = sum(fitness_values)
for _ in range(len(population)):
pick = random.uniform(0, total_fitness)
current = 0
for ind, fit in zip(population, fitness_values):
current += fit
if current > pick:
selected.append(ind)
break
return selected
交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
变异操作
def mutation(individual, mutation_rate):
for i in range(len(individual)):
if random.random() < mutation_rate:
individual[i] = 1 - individual[i]
return individual
遗传算法主程序
def genetic_algorithm(pop_size, gene_length, generations, mutation_rate):
population = initialize_population(pop_size, gene_length)
for generation in range(generations):
fitness_values = [fitness_function(ind) for ind in population]
selected_population = selection(population, fitness_values)
new_population = []
for i in range(0, len(selected_population), 2):
parent1 = selected_population[i]
parent2 = selected_population[i + 1]
child1, child2 = crossover(parent1, parent2)
new_population.extend([child1, child2])
population = [mutation(ind, mutation_rate) for ind in new_population]
best_individual = max(population, key=fitness_function)
print(f"第{generation+1}代最佳个体:{best_individual}, 适应度值:{fitness_function(best_individual)}")
return best_individual
参数设置
pop_size = 10 # 种群大小
gene_length = 5 # 基因长度
generations = 20 # 迭代次数
mutation_rate = 0.01 # 变异率
运行遗传算法
best_solution = genetic_algorithm(pop_size, gene_length, generations, mutation_rate)
print("最佳解决方案:", best_solution)
解释: 上述代码整合了初始化种群、适应度评估、选择、交叉和变异各个步骤,形成完整的遗传算法实现。genetic_algorithm
函数为遗传算法的主程序,输入参数包括种群大小pop_size
、基因长度gene_length
、迭代次数generations
和变异率mutation_rate
。
通过运行上述代码,可以观察到每一代的最佳个体及其适应度值,不断进化,最终找到一个最佳解决方案。
总结
本文详细介绍了如何使用Python实现遗传算法优化样本的核心步骤,包括初始化种群、适应度评估、选择、交叉和变异,并提供了完整的代码示例和解释。通过这些步骤,我们可以有效地使用遗传算法解决复杂的优化和搜索问题。遗传算法具有较强的鲁棒性和全局搜索能力,适用于多种实际应用场景,如函数优化、路径规划、机器学习等。希望本文对您理解和实现遗传算法有所帮助。
相关问答FAQs:
如何使用Python实现遗传算法来优化样本?
遗传算法是一种模拟自然选择的优化技术,通常用于解决复杂的优化问题。在Python中,可以使用库如DEAP(Distributed Evolutionary Algorithms in Python)或自定义实现遗传算法。首先,定义适应度函数,表示样本的优劣;接着,生成初始种群,并进行选择、交叉和变异操作。最后,迭代优化直到满足终止条件。
遗传算法在样本优化中的优势是什么?
遗传算法具有全局搜索能力,能够有效处理高维和复杂的优化问题。与其他算法相比,它不容易陷入局部最优解,能够探索更广泛的解空间。此外,它能够并行处理多个解,提高优化效率。通过选择合适的适应度函数和参数设置,遗传算法能够在许多实际应用中表现出色。
在实现遗传算法时,如何选择合适的参数?
选择遗传算法的参数如种群规模、交叉率、变异率等是影响算法性能的关键因素。一般来说,种群规模应足够大以保证多样性,但也需考虑计算资源。交叉率和变异率的设置通常依赖于具体问题的特性,可以通过实验来调整。进行多次实验和交叉验证,观察优化结果的稳定性,有助于找到最佳参数组合。
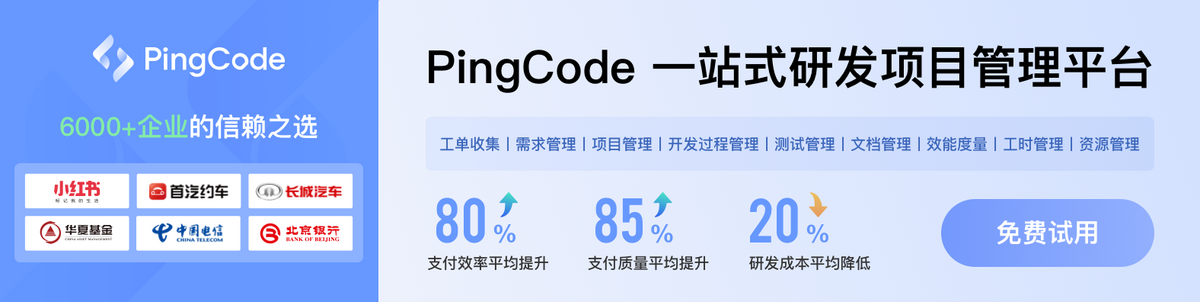