在Python中,将函数作为参数传递的方法包括:使用函数名、lambda表达式、装饰器等。 其中,最常用的方法是直接使用函数名将函数传递给其他函数。这种方法不仅简洁明了,而且非常灵活,可以满足大多数编程需求。
具体来说,假设我们有一个函数 apply_function
,它接受一个函数 f
和一个参数 x
,并返回 f(x)
的结果。我们可以通过将 f
作为参数传递给 apply_function
来实现这一功能。
一、使用函数名
1、直接传递函数名
直接传递函数名是最常见的方法之一。你只需将函数名作为参数传递给另一个函数即可。例如:
def add_one(x):
return x + 1
def apply_function(f, x):
return f(x)
result = apply_function(add_one, 5)
print(result) # 输出: 6
2、使用内置函数
Python 提供了许多内置函数,可以作为参数传递给其他函数。例如:
numbers = [1, 2, 3, 4, 5]
使用内置函数sum
total = sum(numbers)
print(total) # 输出: 15
使用内置函数len
length = len(numbers)
print(length) # 输出: 5
二、使用lambda表达式
1、定义匿名函数
lambda 表达式是一种创建匿名函数的方法,非常适合用于需要简短函数的场景。例如:
def apply_function(f, x):
return f(x)
result = apply_function(lambda x: x * 2, 5)
print(result) # 输出: 10
2、结合内置函数使用
lambda 表达式可以与内置函数结合使用,增强代码的灵活性。例如:
numbers = [1, 2, 3, 4, 5]
使用lambda表达式求平方
squared_numbers = list(map(lambda x: x2, numbers))
print(squared_numbers) # 输出: [1, 4, 9, 16, 25]
三、使用装饰器
1、定义装饰器函数
装饰器是一种特殊的函数,它接受一个函数作为参数,并返回一个新的函数。装饰器可以用于修改或扩展被装饰函数的行为。例如:
def my_decorator(func):
def wrapper(*args, kwargs):
print("Something is happening before the function is called.")
result = func(*args, kwargs)
print("Something is happening after the function is called.")
return result
return wrapper
@my_decorator
def say_hello(name):
print(f"Hello, {name}!")
say_hello("Alice")
2、组合多个装饰器
你可以将多个装饰器组合在一起,以实现更复杂的功能。例如:
def decorator1(func):
def wrapper(*args, kwargs):
print("Decorator 1")
return func(*args, kwargs)
return wrapper
def decorator2(func):
def wrapper(*args, kwargs):
print("Decorator 2")
return func(*args, kwargs)
return wrapper
@decorator1
@decorator2
def say_goodbye(name):
print(f"Goodbye, {name}!")
say_goodbye("Bob")
四、使用函数对象
1、将函数存储在数据结构中
你可以将函数存储在列表或字典等数据结构中,以实现更加灵活的函数调用。例如:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
operations = {
"add": add,
"subtract": subtract
}
result = operations["add"](5, 3)
print(result) # 输出: 8
result = operations["subtract"](5, 3)
print(result) # 输出: 2
2、动态选择函数
通过将函数存储在数据结构中,你可以根据条件动态选择要调用的函数。例如:
def multiply(x, y):
return x * y
def divide(x, y):
if y != 0:
return x / y
else:
raise ValueError("Cannot divide by zero")
operations = {
"multiply": multiply,
"divide": divide
}
operation_name = "divide"
result = operations[operation_name](10, 2)
print(result) # 输出: 5.0
五、应用场景
1、高阶函数
高阶函数是指接受一个或多个函数作为参数,或者返回一个函数作为结果的函数。例如:
def higher_order_function(f, x, y):
return f(x, y)
result = higher_order_function(add, 2, 3)
print(result) # 输出: 5
2、回调函数
回调函数是一种在特定事件发生时调用的函数。例如:
def on_click(callback):
print("Button clicked!")
callback()
def button_clicked():
print("Button click event handled")
on_click(button_clicked)
3、函数式编程
函数式编程是一种编程范式,它强调使用函数来处理数据和逻辑。例如:
def filter_even_numbers(numbers):
return list(filter(lambda x: x % 2 == 0, numbers))
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = filter_even_numbers(numbers)
print(even_numbers) # 输出: [2, 4, 6]
4、事件驱动编程
在事件驱动编程中,函数通常作为事件处理程序传递。例如:
def on_event(event_handler):
event = {"type": "click", "target": "button"}
event_handler(event)
def handle_event(event):
print(f"Event type: {event['type']}, Event target: {event['target']}")
on_event(handle_event)
通过将函数作为参数传递,你可以编写更加灵活和可重用的代码。这种技术在Python编程中非常常见,可以帮助你解决许多复杂的问题。
相关问答FAQs:
如何在Python中将函数作为参数传递给另一个函数?
在Python中,函数是一等公民,意味着它们可以像其他任何对象一样被传递。要将一个函数作为参数传递,只需在定义另一个函数时将其作为参数添加,并在函数体内调用它。例如:
def greet(name):
return f"Hello, {name}!"
def process_greeting(func, name):
return func(name)
result = process_greeting(greet, "Alice")
print(result) # 输出: Hello, Alice!
通过这种方式,您可以轻松地在不同的上下文中重用函数。
使用lambda表达式作为参数传递的优势是什么?
使用lambda表达式可以快速定义简单的匿名函数,这在将函数作为参数传递时非常方便。例如,您可以直接传递一个简单的计算逻辑,而不必事先定义一个完整的函数。如下所示:
result = process_greeting(lambda x: x.upper(), "hello")
print(result) # 输出: HELLO
这种方式在处理简单的逻辑时非常高效,代码也更加简洁。
在传递函数参数时,有哪些常见的错误需要避免?
在将函数作为参数传递时,一些常见的错误包括:忘记在函数调用时添加括号、传递不兼容的参数类型、以及在使用闭包时未正确引用外部变量。确保函数的参数与预期一致,并仔细检查任何闭包中使用的变量,能够有效减少错误发生的几率。例如:
def add(a, b):
return a + b
def calculate(func, x, y):
return func(x, y)
result = calculate(add, 5, 3) # 正确使用
print(result) # 输出: 8
检查函数签名和参数类型,可以确保代码的可靠性和可读性。
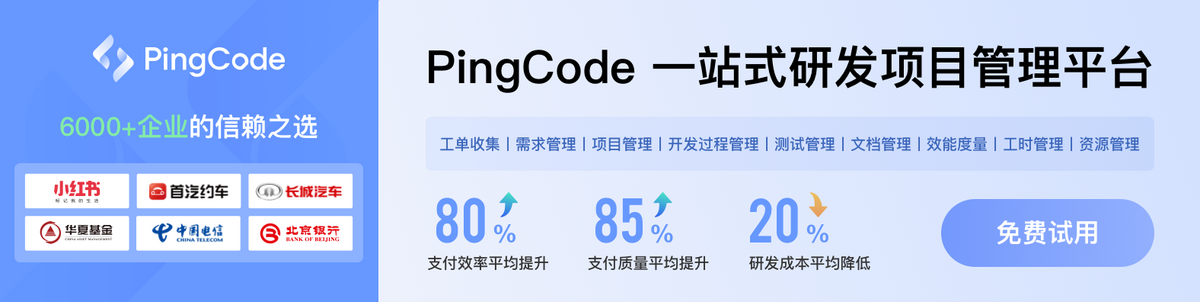