在Python中,提取字符串的最后一个字符可以通过多种方式实现,常见方法包括索引、切片、负索引等。其中,使用负索引是最为简单和直观的方式。负索引从字符串的末尾开始计数,因此字符串的最后一个字符的索引为-1。例如,假设字符串为str = "hello"
,那么str[-1]
就是它的最后一个字符'o'
。接下来,我们将详细讨论这些方法并提供示例代码。
一、索引提取
在Python中,字符串是一个字符序列,因此可以像列表一样使用索引来访问每个字符。索引从0开始计数,因此最后一个字符的索引为len(string) - 1
。
示例代码
string = "hello"
last_char = string[len(string) - 1]
print(last_char) # 输出: o
使用索引提取最后一个字符虽然可行,但代码显得繁琐,尤其是当字符串长度较长时,计算索引会变得复杂。
二、切片提取
字符串切片是提取字符串子序列的常用方法。切片的语法为string[start:end:step]
。若省略start
和end
,则默认从字符串的开头提取到结尾。通过切片,我们可以轻松提取字符串的最后一个字符。
示例代码
string = "hello"
last_char = string[-1:]
print(last_char) # 输出: o
虽然切片方法比索引方法简洁,但它返回的是一个包含单个字符的字符串,而不是单个字符本身。
三、负索引提取
负索引是Python中特有的功能,它使得从字符串末尾开始计数变得简单。负索引从-1开始,表示最后一个字符。
示例代码
string = "hello"
last_char = string[-1]
print(last_char) # 输出: o
使用负索引提取最后一个字符是最简单、最直观的方式,因此在实际编程中是最推荐的方法。
四、使用函数提取
为了提高代码的可读性和重用性,我们可以将提取最后一个字符的逻辑封装在一个函数中。这在需要多次提取字符串最后一个字符时尤为有用。
示例代码
def get_last_char(string):
return string[-1]
string = "hello"
last_char = get_last_char(string)
print(last_char) # 输出: o
通过函数封装,我们不仅提高了代码的可读性,还使得代码更易于维护和重用。
五、应用场景
提取字符串最后一个字符在许多实际应用中非常有用。例如,在处理文件路径时,我们可以根据最后一个字符判断文件类型或目录结构。
示例代码
def check_file_type(file_path):
if file_path[-1] == '/':
return "This is a directory"
elif file_path[-4:] == '.txt':
return "This is a text file"
else:
return "Unknown file type"
file_path = "/user/data/file.txt"
file_type = check_file_type(file_path)
print(file_type) # 输出: This is a text file
通过这种方式,我们可以根据文件路径的最后一个字符轻松判断文件类型或目录结构,从而进行相应的处理。
六、性能考虑
在处理长字符串时,提取最后一个字符的性能问题不容忽视。一般来说,索引和负索引方法的时间复杂度为O(1),即常数时间。而切片方法的时间复杂度为O(n),其中n为字符串长度。因此,对于长字符串,推荐使用索引或负索引方法。
性能比较
import time
def extract_last_char(string, method="index"):
if method == "index":
return string[len(string) - 1]
elif method == "slice":
return string[-1:]
elif method == "negative_index":
return string[-1]
long_string = "a" * 1000000 + "z"
start_time = time.time()
extract_last_char(long_string, method="index")
print("Index method took:", time.time() - start_time)
start_time = time.time()
extract_last_char(long_string, method="slice")
print("Slice method took:", time.time() - start_time)
start_time = time.time()
extract_last_char(long_string, method="negative_index")
print("Negative index method took:", time.time() - start_time)
通过性能比较,我们可以发现,索引和负索引方法在处理长字符串时具有显著的性能优势。
七、异常处理
在实际应用中,字符串可能为空或不存在,因此在提取最后一个字符时需要进行异常处理。我们可以使用try-except
语句来捕获和处理可能的异常。
示例代码
def safe_get_last_char(string):
try:
return string[-1]
except IndexError:
return "String is empty"
string = ""
last_char = safe_get_last_char(string)
print(last_char) # 输出: String is empty
通过异常处理,我们可以确保程序在遇到空字符串时不会崩溃,并返回友好的错误信息。
八、总结
提取字符串的最后一个字符在Python中有多种方法可供选择,包括索引、切片、负索引等。负索引方法最为简单和直观,推荐在实际编程中使用。此外,为了提高代码的可读性和重用性,可以将提取逻辑封装在函数中。在处理长字符串时,索引和负索引方法具有显著的性能优势。最后,考虑到实际应用中的异常情况,建议在提取最后一个字符时进行异常处理,以确保程序的鲁棒性。通过以上方法和技巧,我们可以灵活、高效地提取字符串的最后一个字符,从而解决实际编程中的问题。
相关问答FAQs:
如何在Python中提取字符串的最后一个字符?
在Python中,提取字符串的最后一个字符非常简单。可以使用负索引来实现,例如,string_variable[-1]
将返回字符串string_variable
的最后一个字符。确保在提取之前检查字符串的长度,以避免索引错误。
如果字符串为空,如何处理提取最后一个字符的情况?
当字符串为空时,尝试提取最后一个字符会导致IndexError。为了安全起见,可以在提取之前检查字符串的长度。如果长度为0,可以返回一个默认值或提示用户字符串为空。例如:
if len(string_variable) > 0:
last_char = string_variable[-1]
else:
last_char = "字符串为空"
是否可以使用其他方法来提取字符串的最后一个字符?
除了使用负索引,还可以使用字符串的切片功能来提取最后一个字符。例如,string_variable[len(string_variable)-1]
或string_variable[-1:]
也能实现相同的效果。后者会返回一个包含最后一个字符的子字符串,如果只需要字符本身,使用负索引更为方便。
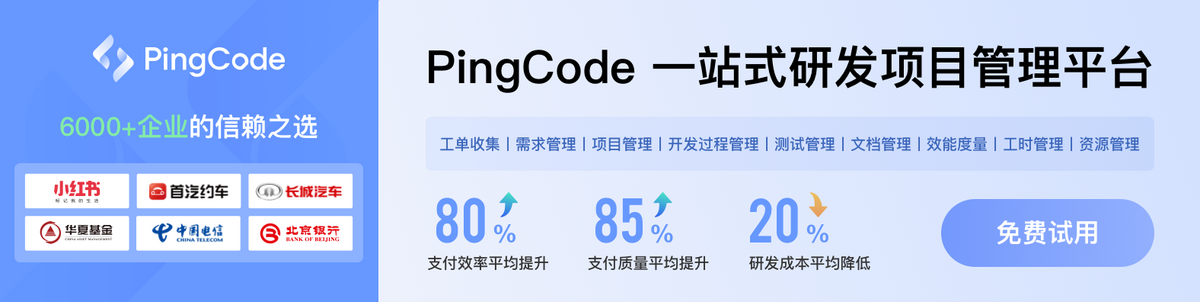