使用Python强力删除文件的方法包括:使用os
模块、使用shutil
模块、使用send2trash
库、使用Pathlib
模块。 其中,最常用的是os
模块和shutil
模块,特别是os
模块提供了直接删除文件和目录的功能。下面我们将详细介绍如何使用这些方法来强力删除文件。
一、使用os模块
os
模块是Python标准库中的一个模块,提供了与操作系统进行交互的接口。使用os
模块可以轻松地删除文件和目录。
删除单个文件
要删除一个文件,可以使用os.remove()
函数。这个函数接受一个文件路径作为参数,并将其删除。
import os
file_path = 'path_to_file.txt'
try:
os.remove(file_path)
print(f'{file_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{file_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {file_path}.')
except Exception as e:
print(f'Error occurred: {e}')
删除空目录
要删除一个空目录,可以使用os.rmdir()
函数。这个函数接受一个目录路径作为参数,并将其删除。
directory_path = 'path_to_directory'
try:
os.rmdir(directory_path)
print(f'{directory_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{directory_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {directory_path}.')
except OSError:
print(f'{directory_path} is not empty.')
except Exception as e:
print(f'Error occurred: {e}')
删除非空目录
要删除一个非空目录,可以使用os.walk()
结合os.remove()
和os.rmdir()
函数进行递归删除。
def delete_directory(directory_path):
for root, dirs, files in os.walk(directory_path, topdown=False):
for file in files:
os.remove(os.path.join(root, file))
for dir in dirs:
os.rmdir(os.path.join(root, dir))
os.rmdir(directory_path)
try:
delete_directory('path_to_directory')
print(f'Directory has been deleted successfully.')
except FileNotFoundError:
print(f'Directory does not exist.')
except PermissionError:
print(f'Permission denied to delete directory.')
except Exception as e:
print(f'Error occurred: {e}')
二、使用shutil模块
shutil
模块是Python标准库中的一个模块,提供了高级的文件操作功能。使用shutil
模块可以递归地删除目录及其内容。
删除非空目录
要删除一个非空目录,可以使用shutil.rmtree()
函数。这个函数接受一个目录路径作为参数,并将其递归删除。
import shutil
directory_path = 'path_to_directory'
try:
shutil.rmtree(directory_path)
print(f'{directory_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{directory_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {directory_path}.')
except Exception as e:
print(f'Error occurred: {e}')
三、使用send2trash库
send2trash
库是一个第三方库,提供了将文件和目录移动到回收站的功能,而不是直接删除。这样可以避免误删文件。
安装send2trash库
在使用send2trash
库之前,需要先安装它。可以使用以下命令安装:
pip install send2trash
将文件或目录移动到回收站
要将文件或目录移动到回收站,可以使用send2trash.send2trash()
函数。这个函数接受一个文件或目录路径作为参数,并将其移动到回收站。
from send2trash import send2trash
path = 'path_to_file_or_directory'
try:
send2trash(path)
print(f'{path} has been moved to the recycle bin successfully.')
except FileNotFoundError:
print(f'{path} does not exist.')
except PermissionError:
print(f'Permission denied to move {path} to the recycle bin.')
except Exception as e:
print(f'Error occurred: {e}')
四、使用Pathlib模块
Pathlib
模块是Python 3.4引入的一个模块,提供了面向对象的文件系统路径操作。使用Pathlib
模块可以更简洁地删除文件和目录。
删除单个文件
要删除一个文件,可以使用Path.unlink()
方法。这个方法可以删除文件或符号链接。
from pathlib import Path
file_path = Path('path_to_file.txt')
try:
file_path.unlink()
print(f'{file_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{file_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {file_path}.')
except Exception as e:
print(f'Error occurred: {e}')
删除空目录
要删除一个空目录,可以使用Path.rmdir()
方法。这个方法可以删除空目录。
directory_path = Path('path_to_directory')
try:
directory_path.rmdir()
print(f'{directory_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{directory_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {directory_path}.')
except OSError:
print(f'{directory_path} is not empty.')
except Exception as e:
print(f'Error occurred: {e}')
删除非空目录
要删除一个非空目录,可以使用shutil.rmtree()
函数,因为Pathlib
模块本身不提供递归删除目录的功能。
directory_path = Path('path_to_directory')
try:
shutil.rmtree(directory_path)
print(f'{directory_path} has been deleted successfully.')
except FileNotFoundError:
print(f'{directory_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {directory_path}.')
except Exception as e:
print(f'Error occurred: {e}')
五、确保文件被彻底删除
有时候,即使我们使用了上述方法删除文件或目录,文件仍然可能被恢复。这种情况下,我们可以使用一些额外的方法来确保文件被彻底删除。
使用安全删除工具
可以使用一些安全删除工具,例如shred
(适用于Linux)或sdelete
(适用于Windows),这些工具可以覆盖文件内容,使其难以恢复。
在Linux系统上,可以使用shred
命令:
shred -u path_to_file
在Windows系统上,可以使用sdelete
命令:
sdelete path_to_file
使用Python结合安全删除工具
可以通过Python脚本调用这些安全删除工具,确保文件被彻底删除。
import subprocess
def secure_delete(file_path):
try:
result = subprocess.run(['shred', '-u', file_path], check=True)
print(f'{file_path} has been securely deleted.')
except FileNotFoundError:
print(f'{file_path} does not exist.')
except PermissionError:
print(f'Permission denied to delete {file_path}.')
except subprocess.CalledProcessError as e:
print(f'Error occurred: {e}')
secure_delete('path_to_file')
六、删除大文件或大量文件
在删除大文件或大量文件时,可能会遇到性能问题。可以采取以下措施来提高删除效率。
分批删除
如果需要删除大量文件,可以将文件分成多批删除,避免一次性删除导致系统负载过高。
import os
def batch_delete(file_paths, batch_size=100):
for i in range(0, len(file_paths), batch_size):
batch = file_paths[i:i+batch_size]
for file_path in batch:
try:
os.remove(file_path)
print(f'{file_path} has been deleted.')
except Exception as e:
print(f'Error occurred: {e}')
file_paths = ['file1.txt', 'file2.txt', 'file3.txt'] # Add your file paths here
batch_delete(file_paths)
使用多线程或多进程
可以使用多线程或多进程来并行删除文件,提高删除效率。
import os
from concurrent.futures import ThreadPoolExecutor
def delete_file(file_path):
try:
os.remove(file_path)
print(f'{file_path} has been deleted.')
except Exception as e:
print(f'Error occurred: {e}')
file_paths = ['file1.txt', 'file2.txt', 'file3.txt'] # Add your file paths here
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(delete_file, file_paths)
七、处理权限问题
在删除文件和目录时,可能会遇到权限问题。可以采取以下措施来处理权限问题。
更改文件权限
可以使用os.chmod()
函数更改文件权限,使其可删除。
file_path = 'path_to_file.txt'
try:
os.chmod(file_path, 0o777)
os.remove(file_path)
print(f'{file_path} has been deleted.')
except Exception as e:
print(f'Error occurred: {e}')
提升权限
在Linux系统上,可以使用sudo
命令提升权限,删除系统文件。
sudo python3 -c "import os; os.remove('path_to_file')"
八、日志记录
在删除文件和目录时,记录日志可以帮助追踪删除操作,便于调试和审计。
使用logging模块记录日志
可以使用Python的logging
模块记录删除操作的日志。
import os
import logging
logging.basicConfig(filename='delete.log', level=logging.INFO)
def delete_file(file_path):
try:
os.remove(file_path)
logging.info(f'{file_path} has been deleted.')
except Exception as e:
logging.error(f'Error occurred: {e}')
delete_file('path_to_file.txt')
九、总结
通过上述方法,我们可以使用Python强力删除文件和目录,确保文件被彻底删除,避免误删文件,提高删除效率,并处理权限问题。无论是单个文件、空目录还是非空目录,os
模块、shutil
模块、send2trash
库和Pathlib
模块都提供了强大的删除功能。同时,结合安全删除工具、多线程或多进程以及日志记录,可以更好地管理删除操作,确保文件安全和系统性能。
相关问答FAQs:
如何确保使用Python删除文件时不发生错误?
在使用Python删除文件时,确保文件路径正确非常重要。可以使用os.path
模块来验证文件是否存在。代码示例:
import os
file_path = 'your_file.txt'
if os.path.exists(file_path):
os.remove(file_path)
else:
print("文件不存在,无法删除。")
这样做可以避免因路径错误而导致的程序崩溃。
使用Python删除文件后,如何确认文件已被删除?
可以通过再次检查文件是否存在来确认文件是否已被成功删除。使用os.path.exists()
函数可以实现这一点。如果返回值为False
,则表示文件已被删除。代码示例:
if not os.path.exists(file_path):
print("文件已成功删除。")
是否有方法可以在Python中实现批量删除文件?
当然可以。利用os
模块的os.listdir()
方法,可以遍历目录中的所有文件,并根据条件进行删除。例如,删除特定后缀名的文件:
import os
directory = 'your_directory/'
for filename in os.listdir(directory):
if filename.endswith('.txt'):
os.remove(os.path.join(directory, filename))
这种方法可以高效地处理多个文件,确保清理工作能够顺利完成。
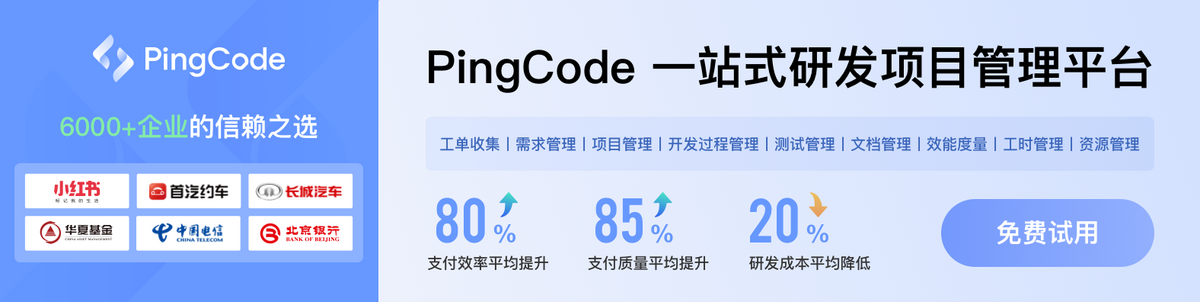