Python匹配英文数字单词的方法包括使用正则表达式、预定义的数字单词列表、自然语言处理库等。 其中,使用正则表达式是一种常见且高效的方法。正则表达式能够灵活地定义匹配模式,并且可以处理复杂的文本匹配任务。以下将详细介绍正则表达式的实现方法。
正则表达式(Regular Expression)是一种强大的文本匹配工具,通过定义字符模式,可以快速匹配、查找和替换文本中的特定内容。在Python中,正则表达式主要通过re
模块来实现。
一、正则表达式匹配英文数字单词
1、基础概念
正则表达式使用一种简洁的语法来定义文本匹配模式,其中:
\b
表示单词边界\d
表示任意一个数字字符+
表示前面的字符可以重复一次或多次
2、实现步骤
-
导入正则表达式模块:
import re
-
定义匹配模式:
例如,匹配单词边界内的数字:
pattern = r'\b\d+\b'
-
编译正则表达式:
regex = re.compile(pattern)
-
匹配文本:
例如,查找文本中的所有数字单词:
text = "There are 3 apples and 20 oranges."
matches = regex.findall(text)
print(matches) # 输出 ['3', '20']
二、预定义的数字单词列表匹配
1、基础概念
预定义的数字单词列表包含常用的英文数字单词(如 "one", "two", "three" 等),通过列表的方式进行匹配。
2、实现步骤
-
定义数字单词列表:
number_words = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten"]
-
匹配文本:
例如,查找文本中的所有数字单词:
text = "I have one apple and two oranges."
matches = [word for word in text.split() if word in number_words]
print(matches) # 输出 ['one', 'two']
三、使用自然语言处理库(如NLTK)
自然语言处理库(NLTK)提供了一些高级功能,可以用于更复杂的文本分析和处理。
1、基础概念
NLTK(Natural Language Toolkit)是一个用于处理自然语言文本的Python库,提供了丰富的工具和资源。
2、实现步骤
-
安装NLTK:
pip install nltk
-
导入NLTK并下载相关资源:
import nltk
nltk.download('punkt')
-
定义匹配函数:
from nltk.tokenize import word_tokenize
def find_number_words(text):
tokens = word_tokenize(text)
number_words = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten"]
matches = [word for word in tokens if word in number_words]
return matches
-
匹配文本:
text = "I have one apple and two oranges."
matches = find_number_words(text)
print(matches) # 输出 ['one', 'two']
四、结合多种方法的综合应用
在实际应用中,可以结合多种方法来提高匹配的准确性和效率。例如,先使用正则表达式匹配数字,再使用预定义的数字单词列表进行过滤。
1、综合实现步骤
-
导入所需模块:
import re
from nltk.tokenize import word_tokenize
-
定义匹配模式和数字单词列表:
pattern = r'\b\d+\b'
number_words = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten"]
-
编译正则表达式:
regex = re.compile(pattern)
-
定义综合匹配函数:
def find_numbers_and_words(text):
# 使用正则表达式匹配数字
number_matches = regex.findall(text)
# 使用NLTK匹配数字单词
tokens = word_tokenize(text)
word_matches = [word for word in tokens if word in number_words]
# 合并结果
return number_matches + word_matches
-
匹配文本:
text = "I have one apple, 2 bananas, and three oranges."
matches = find_numbers_and_words(text)
print(matches) # 输出 ['2', 'one', 'three']
五、错误处理和优化
在实际应用中,处理文本数据时可能会遇到一些特殊情况,需要进行错误处理和优化。例如,处理大小写敏感性、去除标点符号、处理复数形式等。
1、处理大小写敏感性
可以在匹配时将文本和数字单词列表转换为小写,以避免因大小写不同而导致的匹配失败。
def find_number_words_case_insensitive(text):
text = text.lower()
tokens = word_tokenize(text)
number_words = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten"]
matches = [word for word in tokens if word in number_words]
return matches
2、去除标点符号
可以使用正则表达式去除文本中的标点符号,以确保匹配的准确性。
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
text = "I have one apple, two bananas, and three oranges."
clean_text = remove_punctuation(text)
matches = find_number_words_case_insensitive(clean_text)
print(matches) # 输出 ['one', 'two', 'three']
3、处理复数形式
可以通过词形还原(Lemmatization)将复数形式的单词转换为单数形式,以提高匹配的覆盖率。
from nltk.stem import WordNetLemmatizer
nltk.download('wordnet')
def lemmatize_tokens(tokens):
lemmatizer = WordNetLemmatizer()
return [lemmatizer.lemmatize(token) for token in tokens]
tokens = word_tokenize(clean_text)
lemmatized_tokens = lemmatize_tokens(tokens)
matches = [word for word in lemmatized_tokens if word in number_words]
print(matches) # 输出 ['one', 'two', 'three']
六、应用场景
匹配英文数字单词在自然语言处理、文本分析和数据挖掘中有着广泛的应用。例如:
- 文本分类: 可以根据文本中的数字信息对文本进行分类,如识别包含特定数字的文档。
- 信息提取: 可以从文本中提取重要的数字信息,如提取价格、数量等。
- 数据清洗: 可以在数据预处理中识别和处理文本中的数字信息,提高数据的质量和一致性。
七、总结
通过上述方法,可以在Python中高效地匹配英文数字单词。正则表达式提供了灵活的匹配模式,预定义的数字单词列表适用于固定的数字单词匹配,而自然语言处理库则提供了更高级的文本分析功能。结合多种方法,可以提高匹配的准确性和效率,并应用于不同的场景。处理文本数据时,还需要考虑大小写敏感性、标点符号、复数形式等特殊情况,进行相应的优化和错误处理。
相关问答FAQs:
如何使用正则表达式在Python中匹配英文和数字单词?
在Python中,可以使用re
模块中的正则表达式来匹配英文和数字单词。可以使用[a-zA-Z0-9]+
作为模式,这将匹配所有由字母和数字组成的单词。示例代码如下:
import re
text = "Hello world 123 Python 456"
matches = re.findall(r'[a-zA-Z0-9]+', text)
print(matches)
这段代码将输出所有匹配的单词,包括字母和数字。
在Python中,如何提取包含特定字符的英文和数字单词?
如果你想提取包含特定字符的英文或数字单词,可以在正则表达式中添加条件。例如,如果你想匹配包含字母“a”的单词,可以使用[a-zA-Z0-9]*a[a-zA-Z0-9]*
。示例代码如下:
import re
text = "Apple 1234 Banana 5678"
matches = re.findall(r'[a-zA-Z0-9]*a[a-zA-Z0-9]*', text)
print(matches)
这将输出包含字母“a”的所有单词。
如何在Python中区分英文单词和数字单词?
使用正则表达式,您可以分别提取英文单词和数字单词。可以使用[a-zA-Z]+
匹配英文单词,使用\d+
匹配数字单词。示例代码如下:
import re
text = "Python 123 Java 456"
english_words = re.findall(r'[a-zA-Z]+', text)
numbers = re.findall(r'\d+', text)
print("英文单词:", english_words)
print("数字单词:", numbers)
这种方法可以让您清晰地区分文本中的英文单词和数字。
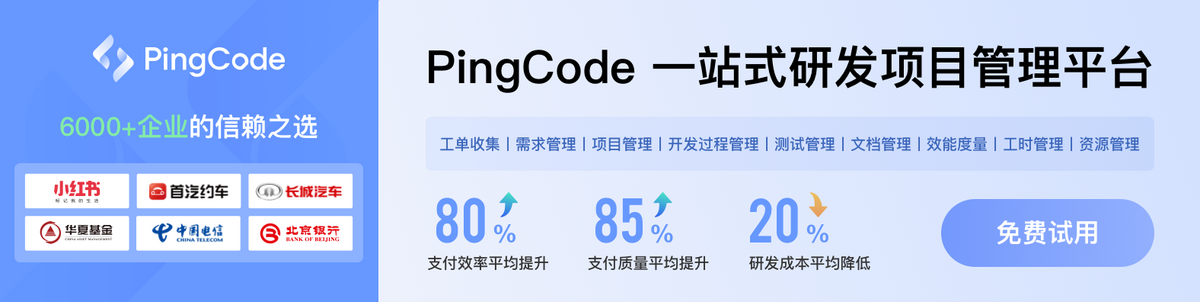