Python中可以通过多线程、多进程、异步编程、使用subprocess模块等方式实现多个命令同时执行。 其中,多线程 是一种常用的方法,适合I/O密集型任务;多进程 则适合CPU密集型任务;异步编程 则适合需要处理大量I/O操作的场景,如网络请求;而 subprocess模块 可以用来同时执行多个外部命令。以下将详细介绍如何使用这些方法中的一种:多线程。
一、多线程
1、多线程基础
多线程是一种在一个进程内实现多任务并行的技术。Python的threading
模块提供了创建和管理线程的功能。以下是一个简单的多线程示例:
import threading
def task(name):
print(f'Task {name} is starting...')
# 模拟任务执行时间
import time
time.sleep(2)
print(f'Task {name} is completed.')
创建多个线程
threads = []
for i in range(5):
thread = threading.Thread(target=task, args=(i,))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print('All tasks are completed.')
这个示例中,task
函数被多个线程同时执行,各自模拟了一个任务的执行时间。通过使用threading.Thread
创建线程,并使用start
方法启动线程,最后使用join
方法等待所有线程完成。
2、多线程应用场景
多线程适用于I/O密集型任务,例如文件读写、网络请求等。以下是一个实际应用的例子:
import threading
import requests
def download_image(url, filename):
print(f'Starting download {filename}...')
response = requests.get(url)
with open(filename, 'wb') as file:
file.write(response.content)
print(f'Completed download {filename}.')
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
]
threads = []
for i, url in enumerate(image_urls):
thread = threading.Thread(target=download_image, args=(url, f'image_{i}.jpg'))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print('All images are downloaded.')
在这个示例中,我们使用多线程同时下载多个图片,极大地提高了下载速度。
二、多进程
1、多进程基础
多进程是指在多个进程中同时执行任务。Python的multiprocessing
模块提供了创建和管理进程的功能。以下是一个简单的多进程示例:
from multiprocessing import Process
def task(name):
print(f'Task {name} is starting...')
import time
time.sleep(2)
print(f'Task {name} is completed.')
创建多个进程
processes = []
for i in range(5):
process = Process(target=task, args=(i,))
processes.append(process)
process.start()
等待所有进程完成
for process in processes:
process.join()
print('All tasks are completed.')
这个示例中,task
函数被多个进程同时执行,各自模拟了一个任务的执行时间。通过使用Process
创建进程,并使用start
方法启动进程,最后使用join
方法等待所有进程完成。
2、多进程应用场景
多进程适用于CPU密集型任务,例如计算密集型的图像处理、数据分析等。以下是一个实际应用的例子:
from multiprocessing import Process
import numpy as np
def matrix_multiplication(matrix1, matrix2, result, index):
result[index] = np.dot(matrix1, matrix2)
matrix1 = np.random.rand(1000, 1000)
matrix2 = np.random.rand(1000, 1000)
results = [None] * 4
processes = []
for i in range(4):
process = Process(target=matrix_multiplication, args=(matrix1, matrix2, results, i))
processes.append(process)
process.start()
for process in processes:
process.join()
print('All matrix multiplications are completed.')
在这个示例中,我们使用多进程同时进行多个矩阵乘法运算,极大地提高了计算速度。
三、异步编程
1、异步编程基础
异步编程是一种非阻塞的编程模型,通过事件循环实现任务的并发执行。Python的asyncio
模块提供了异步编程的功能。以下是一个简单的异步编程示例:
import asyncio
async def task(name):
print(f'Task {name} is starting...')
await asyncio.sleep(2)
print(f'Task {name} is completed.')
async def main():
tasks = [task(i) for i in range(5)]
await asyncio.gather(*tasks)
asyncio.run(main())
这个示例中,task
函数被多个异步任务同时执行,各自模拟了一个任务的执行时间。通过使用asyncio.run
运行主协程main
,并使用gather
方法等待所有异步任务完成。
2、异步编程应用场景
异步编程适用于需要处理大量I/O操作的场景,例如网络请求、数据库操作等。以下是一个实际应用的例子:
import asyncio
import aiohttp
async def download_image(url, session, filename):
print(f'Starting download {filename}...')
async with session.get(url) as response:
with open(filename, 'wb') as file:
file.write(await response.read())
print(f'Completed download {filename}.')
async def main():
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
]
async with aiohttp.ClientSession() as session:
tasks = [download_image(url, session, f'image_{i}.jpg') for i, url in enumerate(image_urls)]
await asyncio.gather(*tasks)
asyncio.run(main())
在这个示例中,我们使用异步编程同时下载多个图片,极大地提高了下载速度。
四、使用subprocess模块
1、subprocess模块基础
subprocess
模块允许你生成新的进程,连接它们的输入/输出/错误管道,并获得它们的返回码。以下是一个简单的示例:
import subprocess
def run_command(command):
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
return stdout, stderr
commands = [
'ls -l',
'echo "Hello, World!"',
'pwd'
]
for command in commands:
stdout, stderr = run_command(command)
print(f'Command: {command}')
print(f'Stdout: {stdout.decode()}')
print(f'Stderr: {stderr.decode()}')
这个示例中,run_command
函数执行传入的命令,并返回标准输出和标准错误。我们使用subprocess.Popen
创建新的进程,并使用communicate
方法获取输出。
2、subprocess模块应用场景
subprocess
模块适用于需要同时执行多个外部命令的场景,例如批处理脚本、系统管理任务等。以下是一个实际应用的例子:
import subprocess
import threading
def run_command(command):
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
return stdout, stderr
def task(command):
stdout, stderr = run_command(command)
print(f'Command: {command}')
print(f'Stdout: {stdout.decode()}')
print(f'Stderr: {stderr.decode()}')
commands = [
'ls -l',
'echo "Hello, World!"',
'pwd',
'sleep 2',
'date'
]
threads = []
for command in commands:
thread = threading.Thread(target=task, args=(command,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print('All commands are executed.')
在这个示例中,我们使用subprocess
模块和多线程同时执行多个外部命令,极大地提高了执行速度。
五、总结
多线程、多进程、异步编程、使用subprocess模块 是Python中实现多个命令同时执行的常用方法。多线程 适合I/O密集型任务,多进程 适合CPU密集型任务,异步编程 适合处理大量I/O操作的场景,subprocess模块 适合同时执行多个外部命令。在实际应用中,可以根据具体需求选择合适的方法,以提高程序的执行效率和性能。
相关问答FAQs:
如何在Python中实现多个命令并行执行?
在Python中,实现多个命令的并行执行可以使用多线程或多进程模块。threading
模块适合处理I/O密集型任务,而multiprocessing
模块则更适合CPU密集型任务。使用concurrent.futures
模块可以简化多线程和多进程的实现,提供了简单的接口来管理并行任务。您可以根据任务的性质选择合适的方式来实现并行执行。
Python中是否有库可以帮助管理并行命令的执行?
确实有多个库可以帮助管理并行命令的执行。比如subprocess
模块可以用于启动子进程并与其交互,适合执行外部命令。而asyncio
库则允许您以异步方式运行多个协程,使得在等待某个命令完成时,可以同时执行其他任务。选择合适的库取决于您的具体需求以及任务的性质。
在Python中如何处理并行执行的命令结果?
处理并行执行命令的结果通常可以通过回调函数或Future对象来实现。使用concurrent.futures
模块时,可以使用as_completed()
方法来获取每个任务的结果,并进行相应的处理。对于每个命令的执行结果,您可以将其存储在列表或字典中,方便后续使用和分析。这样可以确保您能有效管理和使用每个并行命令的返回数据。
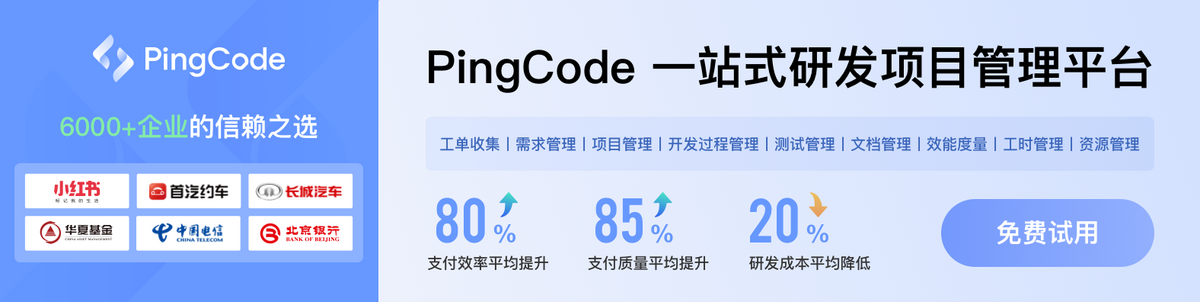