在Python中拼接字符串有多种方法,其中包括使用加号(+)、join()方法、格式化字符串和f-strings。常用的字符串拼接方法有:加号(+)、join()方法、格式化字符串、f-strings。其中,f-strings 是Python 3.6及之后版本引入的一种新的字符串格式化方法,它提供了一种简洁、易读的方式来嵌入变量和表达式到字符串中。
下面我们详细描述一下使用f-strings的方法:
f-strings(格式化字符串字面量):f-strings 是在字符串前加上字母“f”或“F”,并在字符串中使用大括号 {} 包裹变量或表达式。f-strings 提供了一种简洁且强大的方式来嵌入变量和表达式到字符串中。
name = "Alice"
age = 30
greeting = f"Hello, my name is {name} and I am {age} years old."
print(greeting)
在上面的例子中,变量 name
和 age
被嵌入到字符串中,使得 greeting
变量的值为 "Hello, my name is Alice and I am 30 years old."
。
接下来,我们将详细介绍Python中其他字符串拼接方法,并列出一些使用场景和注意事项。
一、使用加号(+)拼接字符串
使用加号(+)是最基本的字符串拼接方法。它简单直观,但在拼接大量字符串时效率较低,因为每次拼接都会创建新的字符串对象。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # 输出: Hello World
注意事项
- 效率低下:在循环中使用加号拼接大量字符串时,效率较低。因为每次拼接都会创建一个新的字符串对象。
- 可读性:对于简单的字符串拼接,使用加号非常直观,但对于复杂的拼接,代码的可读性可能会降低。
二、使用 join() 方法拼接字符串
join() 方法是推荐的字符串拼接方式之一,尤其是在需要拼接大量字符串时。它不仅效率高,而且代码简洁。
words = ["Hello", "World", "from", "Python"]
sentence = " ".join(words)
print(sentence) # 输出: Hello World from Python
注意事项
- 高效:join() 方法在拼接大量字符串时效率更高,因为它只会创建一个新的字符串对象。
- 易读:代码简洁易读,适用于拼接列表或元组中的字符串。
三、使用格式化字符串
格式化字符串是拼接字符串的另一种常用方法,它通过占位符来嵌入变量值。Python提供了多种格式化字符串的方法,包括 %
操作符、str.format() 方法和f-strings。
1. 使用 % 操作符
这种方法类似于C语言中的printf函数,通过 %
操作符来嵌入变量值。
name = "Alice"
age = 30
greeting = "Hello, my name is %s and I am %d years old." % (name, age)
print(greeting)
2. 使用 str.format() 方法
str.format() 方法提供了一种更灵活的字符串格式化方式,通过 {}
占位符来嵌入变量值。
name = "Alice"
age = 30
greeting = "Hello, my name is {} and I am {} years old.".format(name, age)
print(greeting)
四、使用 f-strings(格式化字符串字面量)
f-strings 是 Python 3.6 引入的一种新的字符串格式化方法,它提供了一种简洁、易读的方式来嵌入变量和表达式到字符串中。
name = "Alice"
age = 30
greeting = f"Hello, my name is {name} and I am {age} years old."
print(greeting)
注意事项
- 简洁:f-strings 语法简洁,代码更易读。
- 强大:支持嵌入任意表达式,可以进行复杂的字符串拼接。
- 版本限制:仅适用于 Python 3.6 及之后的版本。
五、其他字符串拼接方法
1. 使用列表推导式和 join()
在处理大量数据时,可以结合列表推导式和 join() 方法来提高效率。
words = ["word" + str(i) for i in range(1000)]
sentence = " ".join(words)
print(sentence)
2. 使用生成器表达式和 join()
生成器表达式与列表推导式类似,但不会一次性创建所有元素,可以节省内存。
words = ("word" + str(i) for i in range(1000))
sentence = " ".join(words)
print(sentence)
六、字符串拼接的应用场景
1. 动态生成SQL语句
在数据库操作中,动态生成SQL语句是常见的需求。例如,拼接查询条件。
table_name = "users"
conditions = ["age > 30", "country = 'USA'"]
query = f"SELECT * FROM {table_name} WHERE {' AND '.join(conditions)}"
print(query)
2. 动态生成HTML内容
在Web开发中,动态生成HTML内容也是常见的需求。例如,生成表格行。
rows = [
{"name": "Alice", "age": 30},
{"name": "Bob", "age": 25}
]
html_rows = "".join([f"<tr><td>{row['name']}</td><td>{row['age']}</td></tr>" for row in rows])
html_table = f"<table>{html_rows}</table>"
print(html_table)
七、性能比较
不同的字符串拼接方法在性能上有所差异。在拼接少量字符串时,性能差异不明显;但在拼接大量字符串时,性能差异显著。
1. 小规模字符串拼接
在拼接少量字符串时,加号(+)、join() 方法、格式化字符串和 f-strings 的性能差异较小。
import time
str1 = "Hello"
str2 = "World"
num_iterations = 100000
使用加号拼接
start_time = time.time()
for _ in range(num_iterations):
result = str1 + " " + str2
end_time = time.time()
print("加号拼接耗时:", end_time - start_time)
使用 join() 方法
start_time = time.time()
for _ in range(num_iterations):
result = " ".join([str1, str2])
end_time = time.time()
print("join() 方法耗时:", end_time - start_time)
使用格式化字符串
start_time = time.time()
for _ in range(num_iterations):
result = "Hello, my name is {} and I am {} years old.".format(str1, str2)
end_time = time.time()
print("格式化字符串耗时:", end_time - start_time)
使用 f-strings
start_time = time.time()
for _ in range(num_iterations):
result = f"{str1} {str2}"
end_time = time.time()
print("f-strings 耗时:", end_time - start_time)
2. 大规模字符串拼接
在拼接大量字符串时,join() 方法和生成器表达式的性能更优。
import time
words = ["word" + str(i) for i in range(10000)]
使用加号拼接
start_time = time.time()
result = ""
for word in words:
result += word + " "
end_time = time.time()
print("加号拼接耗时:", end_time - start_time)
使用 join() 方法
start_time = time.time()
result = " ".join(words)
end_time = time.time()
print("join() 方法耗时:", end_time - start_time)
使用生成器表达式和 join()
start_time = time.time()
result = " ".join("word" + str(i) for i in range(10000))
end_time = time.time()
print("生成器表达式和 join() 耗时:", end_time - start_time)
八、总结
在Python中,字符串拼接有多种方法可供选择。常用的字符串拼接方法有:加号(+)、join()方法、格式化字符串、f-strings。其中,f-strings 提供了一种简洁且强大的方式来嵌入变量和表达式到字符串中,适用于Python 3.6及之后的版本。对于拼接大量字符串,推荐使用join()方法或生成器表达式,这两种方法在性能上更具优势。在实际应用中,可以根据具体需求选择合适的字符串拼接方法,以提高代码的可读性和执行效率。
相关问答FAQs:
如何在Python中拼接多个字符串?
在Python中,可以通过多种方式来拼接字符串。最常用的方法是使用加号(+)运算符,将多个字符串直接相加。此外,使用join()
方法也是一个高效的选择,尤其是在需要拼接大量字符串时。示例代码如下:
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2 # 使用加号拼接
print(result) # 输出: Hello World
# 使用join方法
words = ["Hello", "World"]
result_join = " ".join(words) # 使用join拼接
print(result_join) # 输出: Hello World
在Python中拼接字符串时会遇到哪些常见问题?
在进行字符串拼接时,用户可能会遇到一些常见问题,比如类型错误。尝试拼接一个字符串和一个非字符串类型(如整数或列表)会导致错误。解决这一问题的方法是使用str()
函数将非字符串类型转换为字符串。例如:
num = 10
result = "The number is " + str(num) # 正确拼接
print(result) # 输出: The number is 10
此外,确保拼接的字符串之间有适当的分隔符,以便生成的字符串易于阅读。
是否有推荐的字符串拼接性能优化方法?
对于需要频繁拼接字符串的情况,使用加号拼接可能导致性能下降。考虑使用str.join()
方法或者StringIO
模块来提高效率。StringIO
允许在内存中高效地构建字符串,适合处理大量字符串拼接的任务。示例代码如下:
from io import StringIO
output = StringIO()
output.write("Hello")
output.write(" ")
output.write("World")
result = output.getvalue() # 获取拼接结果
print(result) # 输出: Hello World
这种方法在处理大数据量时能够显著提高性能。
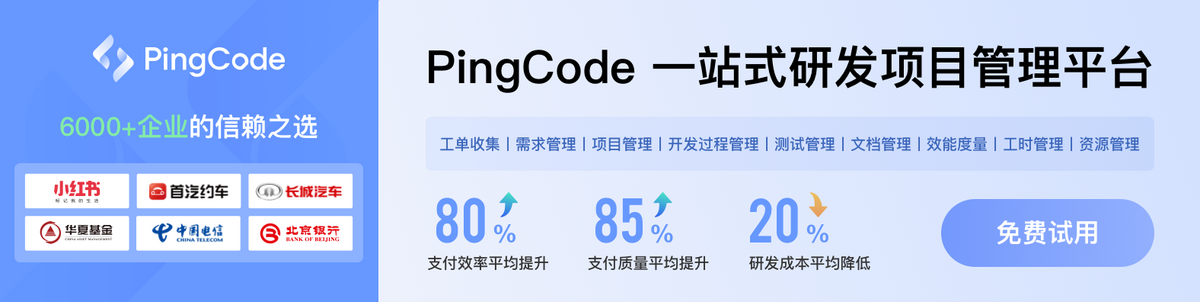