Python程序读取多张图片的方法有多种,常用的方法包括:使用os模块遍历文件夹、使用glob模块匹配文件模式、使用Pillow库加载图片。 其中,使用os模块遍历文件夹 是一种常见且高效的方法。通过os.listdir()函数,我们可以获取指定文件夹中的所有文件和文件夹名,然后通过循环读取图片文件,并使用Pillow库的Image.open()函数打开和处理图片。接下来,我们将详细介绍使用os模块遍历文件夹读取多张图片的方法。
一、使用os模块遍历文件夹读取多张图片
os模块是Python标准库中的一个模块,提供了非常丰富的方法来处理文件和目录。我们可以使用os.listdir()函数获取指定目录中的所有文件和目录名,再通过循环读取图片文件。以下是一个示例代码:
import os
from PIL import Image
def read_images_from_folder(folder_path):
images = []
for filename in os.listdir(folder_path):
if filename.endswith(".jpg") or filename.endswith(".png"):
img_path = os.path.join(folder_path, filename)
img = Image.open(img_path)
images.append(img)
return images
示例使用
folder_path = "path/to/your/image/folder"
images = read_images_from_folder(folder_path)
print(f"Total {len(images)} images read from folder.")
在上述代码中,我们首先导入了os和PIL(Python Imaging Library)模块。然后定义了一个函数read_images_from_folder
,该函数接受一个文件夹路径作为参数。接着,我们使用os.listdir()函数获取文件夹中的所有文件和目录名,并通过循环判断文件名是否以“.jpg”或“.png”结尾,如果是,则使用Image.open()函数打开图片并添加到images列表中。最后,返回包含所有图片的images列表。
二、使用glob模块匹配文件模式读取多张图片
glob模块提供了一个在目录中查找符合特定模式的文件的功能。我们可以使用glob模块来匹配图片文件的模式,从而读取多张图片。以下是一个示例代码:
import glob
from PIL import Image
def read_images_with_glob(folder_path):
images = []
image_files = glob.glob(f"{folder_path}/*.jpg") + glob.glob(f"{folder_path}/*.png")
for img_path in image_files:
img = Image.open(img_path)
images.append(img)
return images
示例使用
folder_path = "path/to/your/image/folder"
images = read_images_with_glob(folder_path)
print(f"Total {len(images)} images read from folder.")
在上述代码中,我们首先导入了glob和PIL模块。然后定义了一个函数read_images_with_glob
,该函数接受一个文件夹路径作为参数。接着,我们使用glob.glob()函数匹配文件夹中所有以“.jpg”和“.png”结尾的文件路径,并将它们合并到一个列表中。最后,通过循环读取每个图片文件,并使用Image.open()函数打开图片并添加到images列表中,返回包含所有图片的images列表。
三、使用Pillow库加载图片
Pillow(PIL Fork)是Python图像处理库,可以用来打开、操作和保存许多不同格式的图像文件。Pillow库的Image模块提供了打开图像文件的方法。以下是一个示例代码:
from PIL import Image
def read_images_with_pillow(image_paths):
images = []
for img_path in image_paths:
img = Image.open(img_path)
images.append(img)
return images
示例使用
image_paths = ["path/to/your/image1.jpg", "path/to/your/image2.png", "path/to/your/image3.jpg"]
images = read_images_with_pillow(image_paths)
print(f"Total {len(images)} images read.")
在上述代码中,我们首先导入了PIL模块。然后定义了一个函数read_images_with_pillow
,该函数接受一个图片文件路径列表作为参数。接着,通过循环读取每个图片文件,并使用Image.open()函数打开图片并添加到images列表中,返回包含所有图片的images列表。
四、结合多个方法的综合应用
在实际应用中,我们可以结合多个方法来提高代码的灵活性和可读性。例如,我们可以先使用os模块遍历文件夹获取所有图片文件路径,然后使用Pillow库加载图片。以下是一个综合示例代码:
import os
from PIL import Image
def get_image_files(folder_path, extensions=[".jpg", ".png"]):
image_files = []
for filename in os.listdir(folder_path):
if any(filename.endswith(ext) for ext in extensions):
img_path = os.path.join(folder_path, filename)
image_files.append(img_path)
return image_files
def read_images(image_files):
images = []
for img_path in image_files:
img = Image.open(img_path)
images.append(img)
return images
示例使用
folder_path = "path/to/your/image/folder"
image_files = get_image_files(folder_path)
images = read_images(image_files)
print(f"Total {len(images)} images read from folder.")
在上述代码中,我们首先定义了一个函数get_image_files
,该函数接受一个文件夹路径和一个文件扩展名列表作为参数,通过os.listdir()函数获取文件夹中的所有文件名,并判断文件名是否以指定的扩展名结尾,如果是,则将文件路径添加到image_files列表中。接着,定义了一个函数read_images
,该函数接受一个图片文件路径列表作为参数,通过循环读取每个图片文件,并使用Image.open()函数打开图片并添加到images列表中。最后,结合两个函数实现从文件夹中读取多张图片。
五、处理读取图片时的异常
在读取图片时,可能会遇到各种异常情况,例如文件不存在、文件格式不正确等。为了提高代码的健壮性,我们需要对这些异常进行处理。以下是一个示例代码:
import os
from PIL import Image
def get_image_files(folder_path, extensions=[".jpg", ".png"]):
image_files = []
for filename in os.listdir(folder_path):
if any(filename.endswith(ext) for ext in extensions):
img_path = os.path.join(folder_path, filename)
image_files.append(img_path)
return image_files
def read_images(image_files):
images = []
for img_path in image_files:
try:
img = Image.open(img_path)
images.append(img)
except (FileNotFoundError, IOError) as e:
print(f"Error reading image {img_path}: {e}")
return images
示例使用
folder_path = "path/to/your/image/folder"
image_files = get_image_files(folder_path)
images = read_images(image_files)
print(f"Total {len(images)} images read from folder.")
在上述代码中,我们在read_images
函数中使用try-except语句捕获并处理可能的异常情况。如果读取图片时发生FileNotFoundError或IOError异常,则打印错误信息并继续处理下一个图片文件。
六、在数据处理和机器学习中的应用
读取多张图片在数据处理和机器学习中非常常见。例如,在图像分类、目标检测、图像分割等任务中,我们通常需要读取大量的图片进行训练和测试。以下是一个使用TensorFlow和Keras读取多张图片进行图像分类的示例代码:
import os
import numpy as np
from PIL import Image
import tensorflow as tf
from tensorflow.keras.preprocessing.image import img_to_array
def get_image_files(folder_path, extensions=[".jpg", ".png"]):
image_files = []
for filename in os.listdir(folder_path):
if any(filename.endswith(ext) for ext in extensions):
img_path = os.path.join(folder_path, filename)
image_files.append(img_path)
return image_files
def load_and_preprocess_images(image_files, target_size=(224, 224)):
images = []
for img_path in image_files:
try:
img = Image.open(img_path)
img = img.resize(target_size)
img_array = img_to_array(img)
img_array = np.expand_dims(img_array, axis=0)
img_array = tf.keras.applications.mobilenet_v2.preprocess_input(img_array)
images.append(img_array)
except (FileNotFoundError, IOError) as e:
print(f"Error reading image {img_path}: {e}")
return np.vstack(images)
示例使用
folder_path = "path/to/your/image/folder"
image_files = get_image_files(folder_path)
images = load_and_preprocess_images(image_files)
print(f"Total {images.shape[0]} images read and preprocessed.")
创建和训练模型
model = tf.keras.applications.MobileNetV2(weights="imagenet", include_top=False, input_shape=(224, 224, 3))
model.summary()
在上述代码中,我们使用了TensorFlow和Keras库。首先定义了一个函数load_and_preprocess_images
,该函数接受一个图片文件路径列表和目标图像大小作为参数,通过循环读取每个图片文件,调整图像大小,并将图像转换为NumPy数组。然后,使用MobileNetV2模型进行图像分类。
七、使用多线程或多进程加速读取图片
在读取大量图片时,单线程处理可能会比较慢。我们可以使用多线程或多进程来加速读取图片。以下是一个使用多线程读取图片的示例代码:
import os
import threading
from PIL import Image
def get_image_files(folder_path, extensions=[".jpg", ".png"]):
image_files = []
for filename in os.listdir(folder_path):
if any(filename.endswith(ext) for ext in extensions):
img_path = os.path.join(folder_path, filename)
image_files.append(img_path)
return image_files
def read_image(img_path, images):
try:
img = Image.open(img_path)
images.append(img)
except (FileNotFoundError, IOError) as e:
print(f"Error reading image {img_path}: {e}")
def read_images_multithreaded(image_files):
images = []
threads = []
for img_path in image_files:
thread = threading.Thread(target=read_image, args=(img_path, images))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return images
示例使用
folder_path = "path/to/your/image/folder"
image_files = get_image_files(folder_path)
images = read_images_multithreaded(image_files)
print(f"Total {len(images)} images read from folder.")
在上述代码中,我们首先定义了一个函数read_image
,该函数接受一个图片文件路径和一个列表作为参数,尝试读取图片并添加到列表中。接着,定义了一个函数read_images_multithreaded
,该函数接受一个图片文件路径列表,通过多线程读取图片。在循环中,我们为每个图片文件创建一个线程,并启动线程。最后,等待所有线程完成后,返回包含所有图片的images列表。
八、总结
通过本文的介绍,我们了解了Python读取多张图片的多种方法,包括使用os模块遍历文件夹、使用glob模块匹配文件模式、使用Pillow库加载图片等。同时,我们还介绍了处理读取图片时的异常、在数据处理和机器学习中的应用、以及使用多线程或多进程加速读取图片的方法。希望这些内容对你有所帮助。
相关问答FAQs:
如何在Python中使用库读取多张图片?
在Python中,可以使用多种库来读取多张图片。常用的库包括PIL(Pillow)、OpenCV和Matplotlib等。以PIL为例,可以通过循环遍历图像文件的路径列表,使用Image.open()
方法逐一读取每张图片。确保在读取之前已安装相应的库,并导入必要的模块。
读取多张图片时需要注意哪些文件格式?
在读取多张图片时,常见的文件格式包括JPEG、PNG、BMP和GIF等。确保所使用的库支持这些格式。Pillow库支持大多数常见格式,而OpenCV则更适合处理视频和图像流。根据需求选择合适的格式和库,可以确保图片读取的顺利进行。
如何处理读取多张图片后的数据?
读取多张图片后,通常需要对数据进行处理。可以将图像数据存储在列表或数组中,以便后续操作,例如图像增强、特征提取或模型训练。如果使用NumPy数组,可以更方便地进行数学运算和图像处理。此外,确保在处理过程中关注内存管理,以防止大规模图像处理时出现性能问题。
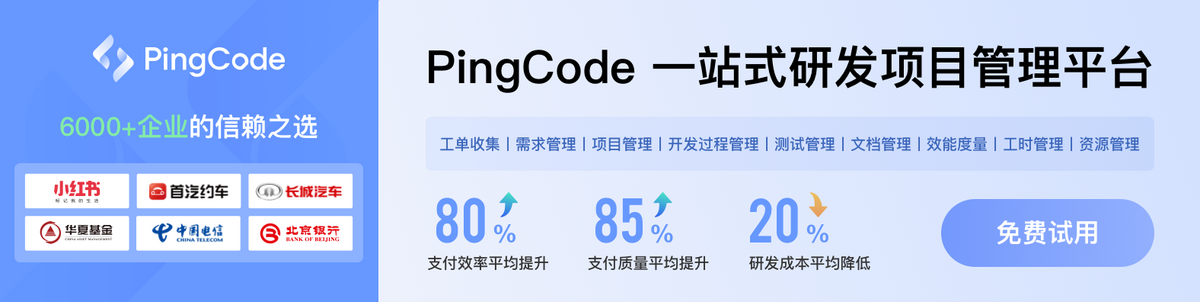