要在大圆上画小圆,可以使用Python中的Matplotlib库。通过计算小圆的坐标位置、利用Matplotlib的绘图功能、并通过循环实现多个小圆的绘制,可以实现这个效果。以下是具体步骤:
- 计算小圆的坐标位置:确定大圆的半径和小圆的半径,利用三角函数计算小圆的中心点在大圆上的位置。
- 使用Matplotlib绘制大圆和小圆:利用Matplotlib库中的
Circle
对象绘制圆形,并通过循环实现多个小圆的绘制。
下面详细介绍如何实现这些步骤。
一、计算小圆的坐标位置
首先,我们需要确定大圆和小圆的半径,并计算小圆的中心点位置。假设大圆的半径为R,小圆的半径为r。
1.1 确定大圆和小圆的半径
R = 10 # 大圆半径
r = 1 # 小圆半径
1.2 计算小圆的中心点位置
小圆的中心点在大圆的边缘上,可以通过极坐标系来计算。假设小圆的中心点与大圆圆心的夹角为theta,则小圆的中心点坐标为:
import numpy as np
theta = np.linspace(0, 2 * np.pi, num=12, endpoint=False) # 12个小圆的角度
x = R * np.cos(theta)
y = R * np.sin(theta)
二、使用Matplotlib绘制大圆和小圆
使用Matplotlib库中的Circle
对象绘制大圆和小圆,并通过循环实现多个小圆的绘制。
2.1 导入Matplotlib库
import matplotlib.pyplot as plt
2.2 绘制大圆和小圆
fig, ax = plt.subplots()
绘制大圆
big_circle = plt.Circle((0, 0), R, color='blue', fill=False)
ax.add_artist(big_circle)
绘制小圆
for (xi, yi) in zip(x, y):
small_circle = plt.Circle((xi, yi), r, color='red', fill=True)
ax.add_artist(small_circle)
设置轴的比例和范围
ax.set_aspect('equal')
ax.set_xlim(-R-r-1, R+r+1)
ax.set_ylim(-R-r-1, R+r+1)
plt.show()
这样就可以在大圆的边缘上绘制12个等间距的小圆。
三、进一步优化
可以根据需要对绘图进行进一步优化,例如添加标签、调整颜色、增加交互功能等。
3.1 添加标签
for i, (xi, yi) in enumerate(zip(x, y)):
ax.text(xi, yi, str(i+1), color='black', ha='center', va='center')
3.2 调整颜色
colors = plt.cm.viridis(np.linspace(0, 1, len(theta)))
for (xi, yi), color in zip(zip(x, y), colors):
small_circle = plt.Circle((xi, yi), r, color=color, fill=True)
ax.add_artist(small_circle)
3.3 增加交互功能
可以使用Matplotlib的ginput
函数来实现交互功能,例如点击图形获取坐标等。
coords = plt.ginput(n=3)
print(coords)
通过以上步骤,你可以在大圆上绘制小圆,并根据需要进行进一步的优化和定制。希望这些内容对你有所帮助!
四、实例应用
4.1 在实际应用中的情景
在实际应用中,绘制大圆和小圆的场景非常多。例如,在天文学中,可能需要绘制行星轨道和卫星轨道;在工业设计中,可能需要绘制齿轮和啮合齿轮等等。
4.2 绘制齿轮和啮合齿轮
假设我们需要绘制一个齿轮和其啮合的小齿轮,我们可以利用之前的代码进行修改:
import numpy as np
import matplotlib.pyplot as plt
齿轮参数
R = 10 # 大齿轮半径
r = 2 # 小齿轮半径
num_teeth = 12 # 齿轮齿数
计算小齿轮中心点位置
theta = np.linspace(0, 2 * np.pi, num=num_teeth, endpoint=False)
x = R * np.cos(theta)
y = R * np.sin(theta)
fig, ax = plt.subplots()
绘制大齿轮
big_gear = plt.Circle((0, 0), R, color='blue', fill=False)
ax.add_artist(big_gear)
绘制小齿轮
for (xi, yi) in zip(x, y):
small_gear = plt.Circle((xi, yi), r, color='red', fill=True)
ax.add_artist(small_gear)
设置轴的比例和范围
ax.set_aspect('equal')
ax.set_xlim(-R-r-1, R+r+1)
ax.set_ylim(-R-r-1, R+r+1)
plt.show()
通过以上代码,可以绘制一个大齿轮和其啮合的小齿轮。
五、其他绘图库的使用
除了Matplotlib,Python中还有其他绘图库也能实现类似的功能,例如Plotly和Bokeh。
5.1 使用Plotly绘图
Plotly是一个交互式绘图库,可以生成高质量的图形,并支持在浏览器中进行交互操作。
import plotly.graph_objects as go
import numpy as np
R = 10 # 大圆半径
r = 1 # 小圆半径
num_circles = 12 # 小圆数量
计算小圆中心点位置
theta = np.linspace(0, 2 * np.pi, num=num_circles, endpoint=False)
x = R * np.cos(theta)
y = R * np.sin(theta)
fig = go.Figure()
绘制大圆
fig.add_shape(type="circle", x0=-R, y0=-R, x1=R, y1=R, line=dict(color="blue"))
绘制小圆
for (xi, yi) in zip(x, y):
fig.add_shape(type="circle", x0=xi-r, y0=yi-r, x1=xi+r, y1=yi+r, fillcolor="red")
fig.update_layout(xaxis=dict(scaleanchor="y", scaleratio=1), yaxis=dict(scaleanchor="x", scaleratio=1))
fig.show()
5.2 使用Bokeh绘图
Bokeh是一个交互式可视化库,适用于Web应用程序。
from bokeh.plotting import figure, show
from bokeh.io import output_notebook
import numpy as np
output_notebook()
R = 10 # 大圆半径
r = 1 # 小圆半径
num_circles = 12 # 小圆数量
计算小圆中心点位置
theta = np.linspace(0, 2 * np.pi, num=num_circles, endpoint=False)
x = R * np.cos(theta)
y = R * np.sin(theta)
p = figure(match_aspect=True)
p.circle(0, 0, radius=R, fill_color=None, line_color="blue")
绘制小圆
for (xi, yi) in zip(x, y):
p.circle(xi, yi, radius=r, fill_color="red")
show(p)
通过使用不同的绘图库,可以根据具体需求选择合适的工具来实现大圆和小圆的绘制。
六、总结
通过计算小圆的坐标位置、利用Matplotlib的绘图功能、并通过循环实现多个小圆的绘制,我们可以在大圆上绘制小圆。这些步骤可以应用于各种实际场景,例如天文观测、工业设计等。此外,还可以使用其他绘图库如Plotly和Bokeh来实现类似的功能。通过不断优化和定制,可以生成高质量的图形,以满足不同的需求。希望这些内容能够帮助你更好地理解和应用Python进行图形绘制。
相关问答FAQs:
如何在大圆的周围均匀分布小圆?
可以通过计算小圆的中心坐标来实现均匀分布。首先,确定大圆的半径和小圆的半径,然后根据小圆的数量计算每个小圆的中心角度。利用三角函数(正弦和余弦)可以计算出每个小圆的坐标,从而在大圆周围均匀分布。
在Python中使用哪些库来绘制圆形?
常用的库包括Matplotlib和Pygame。Matplotlib提供了强大的绘图功能,可以轻松绘制圆形和其他形状。Pygame则更适合于开发游戏和图形应用程序,支持动态绘制和交互。
如何调整小圆的大小和颜色?
在使用Matplotlib绘制时,可以通过设置参数来调整小圆的大小和颜色。例如,plt.Circle()
函数的radius
参数可以用来改变圆的半径,而facecolor
参数则可以指定填充颜色。通过这些参数的调整,可以实现不同的视觉效果。
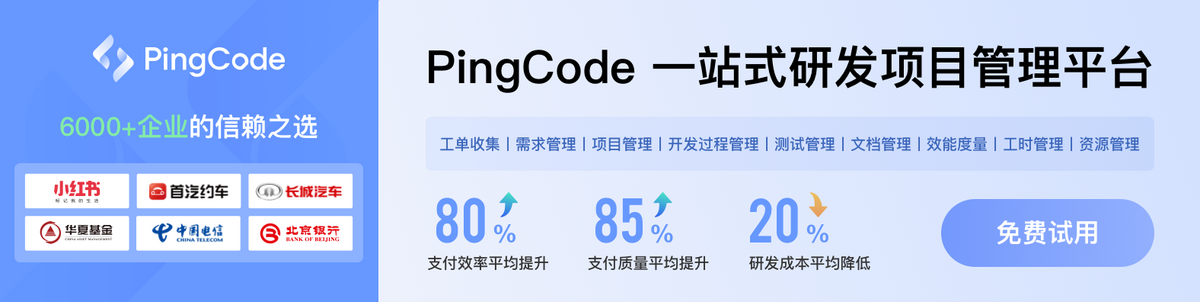