用Python定时发送消息的主要方法包括使用time
模块中的sleep
函数、使用threading
模块定时器、使用schedule
库、以及使用APScheduler
库。其中,使用schedule
库是一种相对简单且灵活的方式,它可以轻松地设置定时任务。以下是对这种方法的详细描述:
使用schedule
库,可以通过简单的代码设置定时任务并发送消息。schedule
库允许你以分钟、小时、天、周为单位设置定时任务,并且可以轻松地与其他Python库整合来发送消息。例如,可以结合smtplib
库发送邮件,或者结合requests
库向Web服务器发送请求。
一、使用schedule
库定时发送消息
1、安装schedule
库
首先,需要安装schedule
库,可以使用pip进行安装:
pip install schedule
2、编写定时任务代码
下面是一个使用schedule
库定时发送消息的示例代码。这个示例代码每一分钟发送一次消息。
import schedule
import time
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = "Scheduled Message"
body = "This is a scheduled message sent every minute."
message.attach(MIMEText(body, "plain"))
try:
server = smtplib.SMTP("smtp.example.com", 587)
server.starttls()
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully.")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit()
Schedule the job every minute
schedule.every(1).minutes.do(send_email)
while True:
schedule.run_pending()
time.sleep(1)
二、使用time
模块中的sleep
函数定时发送消息
1、基本概念
使用time.sleep()
函数可以使程序暂停一段时间,然后继续执行。这种方式简单但不够灵活,因为它无法很好地处理任务调度的复杂需求。
2、示例代码
下面是一个每隔一分钟发送一次消息的示例代码。
import time
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = "Scheduled Message"
body = "This is a scheduled message sent every minute."
message.attach(MIMEText(body, "plain"))
try:
server = smtplib.SMTP("smtp.example.com", 587)
server.starttls()
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully.")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit()
while True:
send_email()
time.sleep(60)
三、使用threading
模块定时器
1、基本概念
threading
模块中的Timer
类可以用于创建一个定时器,在指定的时间间隔之后执行一个函数。它比time.sleep()
更灵活,可以在后台运行定时任务。
2、示例代码
下面是一个使用threading.Timer
每隔一分钟发送一次消息的示例代码。
import threading
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = "Scheduled Message"
body = "This is a scheduled message sent every minute."
message.attach(MIMEText(body, "plain"))
try:
server = smtplib.SMTP("smtp.example.com", 587)
server.starttls()
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully.")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit()
# Schedule the next call
threading.Timer(60, send_email).start()
Start the first call
send_email()
四、使用APScheduler
库定时发送消息
1、安装APScheduler
库
首先,需要安装APScheduler
库,可以使用pip进行安装:
pip install APScheduler
2、编写定时任务代码
下面是一个使用APScheduler
库定时发送消息的示例代码。这个示例代码每一分钟发送一次消息。
from apscheduler.schedulers.blocking import BlockingScheduler
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email():
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = "Scheduled Message"
body = "This is a scheduled message sent every minute."
message.attach(MIMEText(body, "plain"))
try:
server = smtplib.SMTP("smtp.example.com", 587)
server.starttls()
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully.")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit()
scheduler = BlockingScheduler()
scheduler.add_job(send_email, 'interval', minutes=1)
scheduler.start()
总结
定时发送消息在许多自动化任务中非常有用。Python提供了多种方式来实现这一功能,包括使用schedule
库、time.sleep()
函数、threading.Timer
类以及APScheduler
库。根据具体需求选择合适的方法,可以实现高效的定时任务调度和消息发送。
相关问答FAQs:
如何使用Python定时发送消息?
要在Python中定时发送消息,可以使用schedule
库或time
模块结合threading
。你可以先安装schedule
库,并使用它来设置定时任务,例如每隔一段时间发送消息。具体代码示例可以参考以下内容:
import schedule
import time
def send_message():
print("消息已发送")
# 每隔10秒发送一次消息
schedule.every(10).seconds.do(send_message)
while True:
schedule.run_pending()
time.sleep(1)
可以通过哪些方式发送消息?
Python可以通过多种方式发送消息,包括但不限于使用邮件发送(通过smtplib
模块)、发送即时消息(通过API,如Slack、Telegram等)或通过短信服务(如Twilio)。选择适合你需求的方法即可。
定时发送消息时,如何处理异常情况?
在定时发送消息时,处理异常情况非常重要。可以使用try-except
结构来捕获可能的错误,例如网络连接失败或API调用失败,确保程序在遇到错误时不会崩溃。例如:
def send_message():
try:
# 发送消息的代码
print("消息已发送")
except Exception as e:
print(f"发送消息时发生错误: {e}")
如何选择合适的定时发送频率?
选择定时发送频率应考虑消息的类型和接收者的需求。例如,重要通知可能需要即时发送,而定期报告或提醒可以选择更长的间隔。根据具体应用场景,合理安排发送频率,确保信息的有效传达。
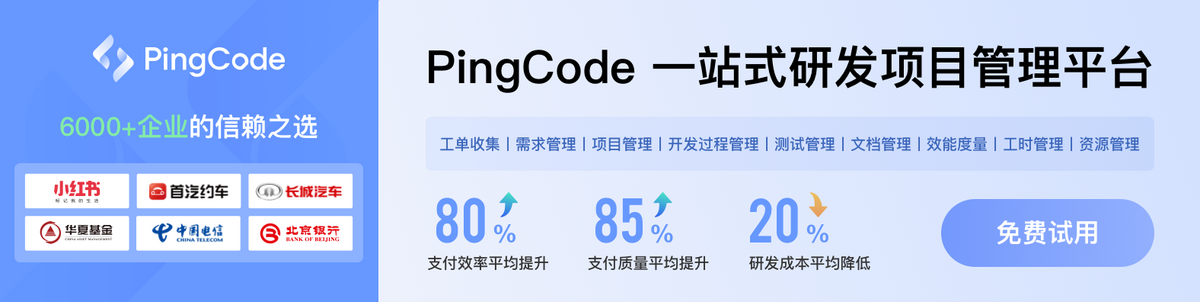