在Python中搜索内容的方法有多种,包括使用字符串方法、正则表达式、以及一些库如BeautifulSoup和requests等。常用的方法包括使用find、index、in运算符、正则表达式、BeautifulSoup库进行网页解析和requests库进行网络请求。
在Python中进行内容搜索时,最基本的方法是利用字符串自带的方法,例如find和index,可以快速查找子字符串在主字符串中的位置。正则表达式(通过re模块)则提供了更复杂的模式匹配功能,适用于需要进行复杂字符串匹配的场景。BeautifulSoup库和requests库常用于网页数据的抓取和解析,结合使用可以实现从网页中提取特定信息的功能。
一、字符串方法
1、find方法
Python的字符串对象有一个find方法,可以用于查找子字符串在主字符串中的位置。如果找到子字符串,则返回其起始位置的索引;如果找不到,则返回-1。
main_string = "Hello, welcome to the world of Python"
sub_string = "welcome"
position = main_string.find(sub_string)
print(f"The position of '{sub_string}' is: {position}")
2、index方法
index方法与find方法类似,不同的是如果找不到子字符串,index方法会引发ValueError异常。
try:
position = main_string.index(sub_string)
print(f"The position of '{sub_string}' is: {position}")
except ValueError:
print(f"'{sub_string}' not found in the main string")
3、in运算符
in运算符可以用于检查子字符串是否存在于主字符串中,返回True或False。
if sub_string in main_string:
print(f"'{sub_string}' found in the main string")
else:
print(f"'{sub_string}' not found in the main string")
二、正则表达式
正则表达式提供了更强大的字符串匹配功能,通过re模块可以实现复杂模式的查找和替换。
1、re.search()
re.search()方法用于在字符串中查找匹配正则表达式的第一个位置,如果找到则返回一个Match对象,否则返回None。
import re
pattern = r"welcome"
match = re.search(pattern, main_string)
if match:
print(f"Match found: {match.group()}")
else:
print("No match found")
2、re.findall()
re.findall()方法返回所有与正则表达式匹配的子字符串的列表。
pattern = r"\b\w{5}\b" # 匹配所有长度为5的单词
matches = re.findall(pattern, main_string)
print(f"Matches found: {matches}")
3、re.finditer()
re.finditer()方法返回一个迭代器,其中包含所有匹配正则表达式的Match对象。
for match in re.finditer(pattern, main_string):
print(f"Match found: {match.group()} at position {match.start()}")
三、BeautifulSoup和requests
1、BeautifulSoup
BeautifulSoup是一个用于解析HTML和XML文档的库,可以轻松提取网页中的数据。
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title.string)
2、requests
requests库用于发送HTTP请求,并获取网页内容。
import requests
url = "http://example.com"
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.title.string)
四、综合运用
将requests和BeautifulSoup结合使用,可以实现从网页中提取特定信息的功能。
url = "http://example.com"
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
links = soup.find_all('a', class_='sister')
for link in links:
print(f"Link text: {link.string}, URL: {link['href']}")
五、其他库
1、scrapy
Scrapy是一个强大的网页爬虫框架,可以用于大规模抓取网页数据。
import scrapy
class ExampleSpider(scrapy.Spider):
name = "example"
start_urls = ["http://example.com"]
def parse(self, response):
for href in response.css('a::attr(href)'):
yield response.follow(href, self.parse)
2、Selenium
Selenium是一个用于自动化测试的工具,也可以用于抓取动态加载的网页数据。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("http://example.com")
title = driver.title
print(f"Title: {title}")
driver.quit()
六、总结
在Python中搜索内容的方法多种多样,根据具体需求选择合适的方法和库,可以高效地完成各种字符串查找和网页数据提取任务。字符串方法适合简单查找,正则表达式适合复杂模式匹配,BeautifulSoup和requests适合网页数据抓取,Scrapy和Selenium则适合大规模和动态网页数据抓取。
无论使用哪种方法,理解其原理和适用场景是关键,这样才能在实际应用中灵活运用,解决实际问题。通过不断学习和实践,相信每一位Python开发者都能熟练掌握这些技巧,提高工作效率。
相关问答FAQs:
在Python中有哪些常用的搜索模块或库?
在Python中,有多个模块和库可以实现内容搜索功能。最常用的包括re
模块,用于正则表达式搜索,适合处理复杂的字符串匹配。fnmatch
模块则可以用于文件名匹配,适合处理文件搜索任务。如果需要处理更高级的文本搜索,可以使用Whoosh
或Whoosh
等全文搜索库,提供了更为强大的索引和查询功能。
如何使用正则表达式在Python中进行内容搜索?
使用正则表达式进行内容搜索非常简单。首先需要导入re
模块,然后使用re.search()
、re.match()
或re.findall()
等方法来查找特定模式的字符串。例如,可以使用re.search(r'pattern', string)
来查找string
中是否包含pattern
,返回匹配的对象或None
。正则表达式提供了强大的模式匹配能力,可以用来匹配字母、数字、空白字符等多种模式。
在Python中如何搜索文件中的特定内容?
要在文件中搜索特定内容,首先需要打开文件并读取其内容。可以使用open()
函数读取文件,然后结合in
运算符或者正则表达式来查找特定字符串。例如,可以使用以下代码来查找文件中是否包含某个字符串:
with open('filename.txt', 'r') as file:
content = file.read()
if 'search_term' in content:
print("Found!")
如果需要更复杂的搜索功能,可以考虑使用re
模块进行模式匹配,或使用grep
命令在大文件中快速搜索。
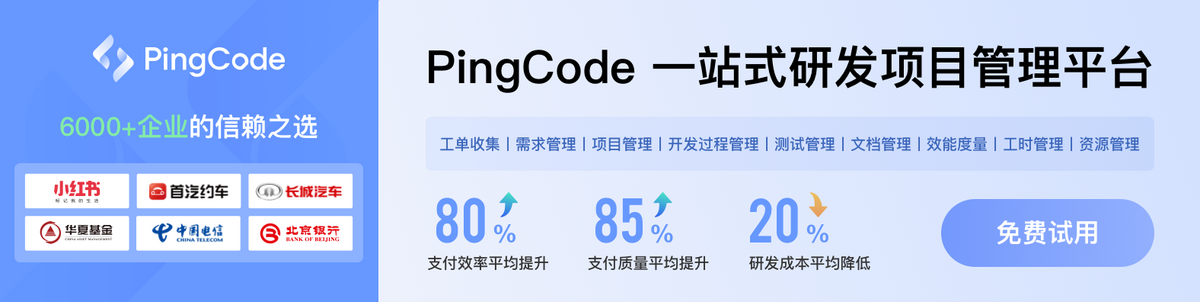