在 Python 中,实现重新输入密码的方法有多种,可以通过循环、条件判断和输入函数来实现。 比如,使用 while
循环和 input
函数,结合密码匹配的逻辑,确保用户输入正确的密码。详细描述如下:
可以使用 getpass
模块来隐藏输入的密码,这样在终端中输入密码时不会显示在屏幕上。通过 while
循环不断检查用户输入的密码是否正确,如果不正确,则提示用户重新输入。
一、使用 while 循环和 getpass 模块
- 首先导入
getpass
模块。 - 设置正确的密码。
- 使用
while
循环让用户输入密码,并检查输入是否正确。 - 如果输入正确,则跳出循环;否则,提示用户重新输入。
import getpass
correct_password = "your_password"
while True:
entered_password = getpass.getpass("Please enter your password: ")
if entered_password == correct_password:
print("Password is correct!")
break
else:
print("Incorrect password, please try again.")
二、提高用户体验的进一步改进
- 增加输入错误次数限制:为了防止用户无限次尝试,可以设置一个最大错误次数。
- 提示用户剩余尝试次数:每次输入错误后,提示用户剩余的尝试次数。
- 提供重置密码功能:在用户多次输入错误后,可以提供一个选项让用户重置密码。
增加输入错误次数限制
通过设置一个计数器来跟踪用户输入错误的次数,超过一定次数则终止程序。
import getpass
correct_password = "your_password"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
entered_password = getpass.getpass("Please enter your password: ")
if entered_password == correct_password:
print("Password is correct!")
break
else:
attempts += 1
print(f"Incorrect password, you have {max_attempts - attempts} attempts left.")
else:
print("Too many failed attempts. Access denied.")
提供重置密码功能
在用户多次输入错误后,可以提供一个选项让用户重置密码。
import getpass
correct_password = "your_password"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
entered_password = getpass.getpass("Please enter your password: ")
if entered_password == correct_password:
print("Password is correct!")
break
else:
attempts += 1
print(f"Incorrect password, you have {max_attempts - attempts} attempts left.")
if attempts == max_attempts:
reset_option = input("Would you like to reset your password? (yes/no): ")
if reset_option.lower() == 'yes':
new_password = getpass.getpass("Enter new password: ")
confirm_password = getpass.getpass("Confirm new password: ")
if new_password == confirm_password:
correct_password = new_password
attempts = 0
print("Password has been reset. Please enter your new password.")
else:
print("Passwords do not match. Access denied.")
else:
print("Too many failed attempts. Access denied.")
三、总结
通过使用 getpass
模块、while
循环和条件判断,可以轻松实现用户密码重新输入的功能。增加输入错误次数限制和重置密码功能可以进一步提高用户体验和安全性。整个过程不仅体现了 Python 编程的灵活性,还展示了如何通过简单的逻辑控制实现复杂的需求。
四、进一步扩展
为了使密码管理更加安全和灵活,可以考虑以下几个方面的改进:
- 密码强度检查:在用户设置新密码时,检查密码的强度,确保密码足够复杂。
- 密码加密存储:将密码加密存储在文件或数据库中,而不是在代码中明文存储。
- 多因素认证:结合其他认证方式(如短信验证码、电子邮件验证)提高安全性。
密码强度检查
密码强度检查可以确保用户设置的密码包含数字、字母和特殊字符,并且长度足够。
import re
import getpass
def check_password_strength(password):
if len(password) < 8:
return "Password must be at least 8 characters long."
if not re.search("[a-z]", password):
return "Password must contain at least one lowercase letter."
if not re.search("[A-Z]", password):
return "Password must contain at least one uppercase letter."
if not re.search("[0-9]", password):
return "Password must contain at least one digit."
if not re.search("[@#$%^&+=]", password):
return "Password must contain at least one special character (@#$%^&+=)."
return None
correct_password = "your_password"
max_attempts = 3
attempts = 0
while attempts < max_attempts:
entered_password = getpass.getpass("Please enter your password: ")
if entered_password == correct_password:
print("Password is correct!")
break
else:
attempts += 1
print(f"Incorrect password, you have {max_attempts - attempts} attempts left.")
if attempts == max_attempts:
reset_option = input("Would you like to reset your password? (yes/no): ")
if reset_option.lower() == 'yes':
while True:
new_password = getpass.getpass("Enter new password: ")
confirm_password = getpass.getpass("Confirm new password: ")
if new_password != confirm_password:
print("Passwords do not match.")
continue
strength_message = check_password_strength(new_password)
if strength_message:
print(strength_message)
else:
correct_password = new_password
attempts = 0
print("Password has been reset. Please enter your new password.")
break
else:
print("Too many failed attempts. Access denied.")
密码加密存储
使用 hashlib
模块将密码加密存储在文件中。
import hashlib
import getpass
def hash_password(password):
return hashlib.sha256(password.encode()).hexdigest()
correct_password_hash = hash_password("your_password")
max_attempts = 3
attempts = 0
while attempts < max_attempts:
entered_password = getpass.getpass("Please enter your password: ")
if hash_password(entered_password) == correct_password_hash:
print("Password is correct!")
break
else:
attempts += 1
print(f"Incorrect password, you have {max_attempts - attempts} attempts left.")
if attempts == max_attempts:
reset_option = input("Would you like to reset your password? (yes/no): ")
if reset_option.lower() == 'yes':
while True:
new_password = getpass.getpass("Enter new password: ")
confirm_password = getpass.getpass("Confirm new password: ")
if new_password != confirm_password:
print("Passwords do not match.")
continue
strength_message = check_password_strength(new_password)
if strength_message:
print(strength_message)
else:
correct_password_hash = hash_password(new_password)
attempts = 0
print("Password has been reset. Please enter your new password.")
break
else:
print("Too many failed attempts. Access denied.")
通过这些扩展,可以进一步提高密码管理的安全性和用户体验。此实现可以应用于各种需要密码认证的场景,如登录系统、敏感操作确认等。
相关问答FAQs:
如何在Python中实现密码输入的安全性?
在Python中,可以通过使用getpass
模块来安全地输入密码。这个模块可以隐藏用户输入的密码,避免在终端中显示明文。这不仅提升了安全性,还能防止他人窥视用户输入的密码。示例代码如下:
import getpass
password = getpass.getpass("请输入密码: ")
print("密码输入成功!")
如何在Python中验证用户重新输入的密码?
为了确保用户记住的密码一致,可以要求用户输入密码两次,并进行比较。下面是一个简单的示例:
import getpass
password1 = getpass.getpass("请输入密码: ")
password2 = getpass.getpass("请再次输入密码: ")
if password1 == password2:
print("密码设置成功!")
else:
print("两次输入的密码不一致,请重新尝试。")
在Python中如何处理密码输入的异常情况?
在处理密码输入时,可能会遇到不同的异常情况,比如用户输入为空或格式不符合要求。可以通过添加条件语句来检查用户输入,并给予相应的提示。例如:
import getpass
while True:
password = getpass.getpass("请输入密码: ")
if len(password) < 6:
print("密码长度必须至少为6个字符,请重新输入。")
else:
print("密码输入成功!")
break
通过以上方法,可以有效地实现密码的重新输入和验证,为用户提供更安全的体验。
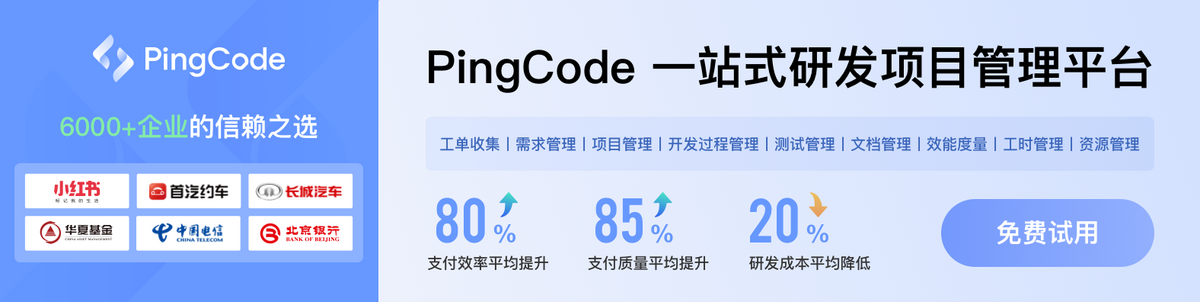