Python进行发邮件的方法包括使用smtplib模块、使用第三方库如yagmail、使用Django等框架自带的邮件发送功能。 其中,最常用的方法是使用Python的内置模块smtplib, 该方法可以直接通过SMTP协议发送邮件,具体步骤包括:设置SMTP服务器、登录邮箱、构建邮件内容以及发送邮件。以下将详细介绍如何使用smtplib发送邮件。
一、SMTP服务器的设置和登录
1、设置SMTP服务器
要发送邮件,首先需要知道所用邮箱的SMTP服务器地址和端口号。以下是一些常用邮箱的SMTP服务器地址和端口号:
- Gmail: smtp.gmail.com, 端口号587(TLS)或465(SSL)
- Outlook: smtp-mail.outlook.com, 端口号587(TLS)
- Yahoo: smtp.mail.yahoo.com, 端口号587(TLS)
2、登录邮箱
使用smtplib模块时,首先需要创建SMTP对象,然后使用邮箱账户和密码登录到SMTP服务器。代码如下:
import smtplib
创建SMTP对象
smtp_obj = smtplib.SMTP('smtp.gmail.com', 587)
smtp_obj.starttls() # 启动TLS加密模式
smtp_obj.login('your_email@gmail.com', 'your_password') # 登录邮箱
二、构建邮件内容
1、邮件头和邮件体
邮件的内容包括邮件头(如发件人、收件人、主题等)和邮件体(实际的邮件内容)。可以使用email模块来构建邮件内容。以下是一个简单的文本邮件的构建示例:
from email.mime.text import MIMEText
构建邮件内容
msg = MIMEText('This is the body of the email.', 'plain', 'utf-8')
msg['From'] = 'your_email@gmail.com'
msg['To'] = 'recipient_email@gmail.com'
msg['Subject'] = 'Test Email'
2、发送HTML格式的邮件
如果需要发送HTML格式的邮件,可以将邮件类型从plain改为html:
msg = MIMEText('<html><body><h1>Hello</h1><p>This is an HTML email.</p></body></html>', 'html', 'utf-8')
三、发送邮件
1、发送邮件
使用SMTP对象的sendmail方法发送邮件,代码如下:
smtp_obj.sendmail('your_email@gmail.com', 'recipient_email@gmail.com', msg.as_string())
2、关闭SMTP连接
完成邮件发送后,需要关闭SMTP连接:
smtp_obj.quit()
总结上述内容,完整的代码如下:
import smtplib
from email.mime.text import MIMEText
设置SMTP服务器地址和端口号
smtp_server = 'smtp.gmail.com'
smtp_port = 587
登录邮箱账户和密码
email_account = 'your_email@gmail.com'
email_password = 'your_password'
创建SMTP对象并登录
smtp_obj = smtplib.SMTP(smtp_server, smtp_port)
smtp_obj.starttls()
smtp_obj.login(email_account, email_password)
构建邮件内容
msg = MIMEText('This is the body of the email.', 'plain', 'utf-8')
msg['From'] = email_account
msg['To'] = 'recipient_email@gmail.com'
msg['Subject'] = 'Test Email'
发送邮件
smtp_obj.sendmail(email_account, 'recipient_email@gmail.com', msg.as_string())
关闭SMTP连接
smtp_obj.quit()
四、使用第三方库yagmail发送邮件
1、安装yagmail
可以使用pip来安装yagmail:
pip install yagmail
2、使用yagmail发送邮件
yagmail简化了邮件的发送过程,以下是一个示例:
import yagmail
登录邮箱
yag = yagmail.SMTP('your_email@gmail.com', 'your_password')
发送邮件
yag.send(
to='recipient_email@gmail.com',
subject='Test Email',
contents='This is the body of the email.'
)
五、使用Django框架发送邮件
1、Django邮件设置
在Django项目的settings.py文件中,配置邮件设置:
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
EMAIL_HOST = 'smtp.gmail.com'
EMAIL_PORT = 587
EMAIL_USE_TLS = True
EMAIL_HOST_USER = 'your_email@gmail.com'
EMAIL_HOST_PASSWORD = 'your_password'
DEFAULT_FROM_EMAIL = EMAIL_HOST_USER
2、发送邮件
在Django视图或其他地方使用send_mail函数发送邮件:
from django.core.mail import send_mail
send_mail(
'Subject here',
'Here is the message.',
'your_email@gmail.com',
['recipient_email@gmail.com'],
fail_silently=False,
)
六、异常处理和安全性
1、异常处理
在实际应用中,邮件发送过程中可能会出现各种异常情况,如网络问题、身份验证失败等。可以使用try-except语句进行异常处理:
try:
smtp_obj = smtplib.SMTP('smtp.gmail.com', 587)
smtp_obj.starttls()
smtp_obj.login('your_email@gmail.com', 'your_password')
smtp_obj.sendmail('your_email@gmail.com', 'recipient_email@gmail.com', msg.as_string())
smtp_obj.quit()
except smtplib.SMTPException as e:
print(f'Error: {e}')
2、保护账户信息
为了保护邮箱账户和密码,可以使用环境变量或配置文件来存储敏感信息,而不是在代码中明文写出:
import os
email_account = os.getenv('EMAIL_ACCOUNT')
email_password = os.getenv('EMAIL_PASSWORD')
七、发送带附件的邮件
1、构建带附件的邮件
可以使用email.mime.multipart模块来创建带附件的邮件:
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
构建邮件内容
msg = MIMEMultipart()
msg['From'] = 'your_email@gmail.com'
msg['To'] = 'recipient_email@gmail.com'
msg['Subject'] = 'Test Email with Attachment'
邮件正文
body = 'This is the body of the email.'
msg.attach(MIMEText(body, 'plain', 'utf-8'))
附件
filename = 'path/to/attachment.txt'
attachment = open(filename, 'rb')
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={filename}')
msg.attach(part)
发送邮件
smtp_obj = smtplib.SMTP('smtp.gmail.com', 587)
smtp_obj.starttls()
smtp_obj.login('your_email@gmail.com', 'your_password')
smtp_obj.sendmail('your_email@gmail.com', 'recipient_email@gmail.com', msg.as_string())
smtp_obj.quit()
attachment.close()
八、发送多种格式的邮件
1、发送HTML和纯文本格式的邮件
为了确保邮件能在各种邮件客户端中正常显示,可以同时发送HTML和纯文本格式的邮件:
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
构建邮件内容
msg = MIMEMultipart('alternative')
msg['From'] = 'your_email@gmail.com'
msg['To'] = 'recipient_email@gmail.com'
msg['Subject'] = 'Test Email with HTML and Plain Text'
纯文本格式
text = 'This is the plain text version of the email.'
part1 = MIMEText(text, 'plain', 'utf-8')
HTML格式
html = '<html><body><h1>Hello</h1><p>This is the HTML version of the email.</p></body></html>'
part2 = MIMEText(html, 'html', 'utf-8')
将两种格式的内容添加到邮件中
msg.attach(part1)
msg.attach(part2)
发送邮件
smtp_obj = smtplib.SMTP('smtp.gmail.com', 587)
smtp_obj.starttls()
smtp_obj.login('your_email@gmail.com', 'your_password')
smtp_obj.sendmail('your_email@gmail.com', 'recipient_email@gmail.com', msg.as_string())
smtp_obj.quit()
九、批量发送邮件
1、批量发送邮件
在需要向多个收件人发送邮件时,可以使用for循环来批量发送邮件:
# 收件人列表
recipients = ['recipient1@gmail.com', 'recipient2@gmail.com', 'recipient3@gmail.com']
循环发送邮件
for recipient in recipients:
msg['To'] = recipient
smtp_obj.sendmail('your_email@gmail.com', recipient, msg.as_string())
2、使用send_message方法
smtplib提供了send_message方法,可以直接传递MIME对象:
smtp_obj.send_message(msg, from_addr='your_email@gmail.com', to_addrs=recipients)
通过上述内容的详细介绍,我们已经了解了如何使用Python进行发邮件的多种方法和技巧,包括使用smtplib模块、第三方库yagmail、Django框架自带功能,以及发送带附件和多种格式的邮件等。希望这些内容能为你在实际应用中提供帮助。
相关问答FAQs:
如何在Python中发送电子邮件?
在Python中发送电子邮件通常可以通过使用内置的smtplib
库来实现。您需要提供SMTP服务器的地址和端口,以及您的电子邮件账户和密码。以下是一个简单的示例代码:
import smtplib
from email.mime.text import MIMEText
# 设置邮件内容
msg = MIMEText("这是一封测试邮件")
msg['Subject'] = '测试主题'
msg['From'] = 'your_email@example.com'
msg['To'] = 'recipient@example.com'
# 连接SMTP服务器并发送邮件
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls() # 启用TLS加密
server.login('your_email@example.com', 'your_password')
server.send_message(msg)
确保将your_email@example.com
和your_password
替换为您自己的电子邮件信息。
使用Python发送邮件需要哪些库?
发送邮件的基本库是smtplib
,此外,email
库中的MIMEText
用于构建邮件内容。对于更复杂的邮件内容,如附件或HTML格式邮件,可以使用email.mime
模块中的其他类,如MIMEBase
和MIMEImage
。
如何处理SMTP服务器的连接问题?
连接SMTP服务器时,可能会遇到一些常见问题。例如,确保SMTP服务器地址和端口号正确,检查您的网络连接是否正常。如果需要,您还可以在代码中添加异常处理机制,以便在连接失败时提供更友好的错误提示。例如:
try:
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login('your_email@example.com', 'your_password')
server.send_message(msg)
except Exception as e:
print(f"发送邮件失败: {e}")
通过这种方式,您可以更好地了解连接问题的原因,并进行相应的调整。
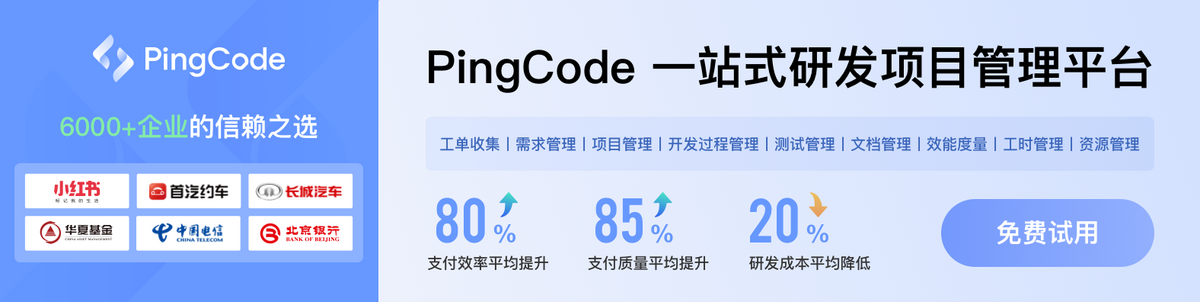