Python提取HTML属性值的方法有多种,其中常用的方法包括使用BeautifulSoup、lxml库、Selenium等。本文将详细介绍这些方法的使用技巧和注意事项。 在实际操作中,我们经常使用BeautifulSoup库来解析HTML并提取属性值,因为它易于使用且功能强大。接下来,我们将详细介绍如何使用BeautifulSoup库提取HTML属性值。
一、使用BeautifulSoup解析HTML
BeautifulSoup是一个用于解析HTML和XML的Python库。它可以很方便地从网页中提取数据。首先,我们需要安装BeautifulSoup库和requests库,以便抓取网页内容并解析HTML。
pip install beautifulsoup4
pip install requests
1、导入必要的库
在开始解析HTML之前,我们需要导入必要的库。
from bs4 import BeautifulSoup
import requests
2、获取网页内容
我们使用requests库获取网页内容。
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
3、解析HTML内容
使用BeautifulSoup解析获取到的HTML内容。
soup = BeautifulSoup(html_content, 'html.parser')
4、提取HTML属性值
假设我们要提取所有a标签的href属性值,可以使用以下代码:
for link in soup.find_all('a'):
href = link.get('href')
print(href)
二、使用lxml库解析HTML
lxml是另一个用于解析HTML和XML的强大库。与BeautifulSoup相比,lxml解析速度更快,但需要安装C语言的依赖包。首先,我们需要安装lxml库。
pip install lxml
1、导入必要的库
from lxml import html
import requests
2、获取网页内容
同样,我们使用requests库获取网页内容。
url = 'https://example.com'
response = requests.get(url)
html_content = response.content
3、解析HTML内容
使用lxml解析获取到的HTML内容。
tree = html.fromstring(html_content)
4、提取HTML属性值
假设我们要提取所有a标签的href属性值,可以使用以下代码:
for link in tree.xpath('//a'):
href = link.get('href')
print(href)
三、使用Selenium解析HTML
Selenium是一个用于自动化网页操作的库,通常用于测试网页应用。它可以模拟用户操作浏览器,并获取动态加载的内容。首先,我们需要安装Selenium库和浏览器驱动。
pip install selenium
1、导入必要的库
from selenium import webdriver
2、设置浏览器驱动
我们使用Chrome浏览器驱动作为示例。
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
3、获取网页内容
url = 'https://example.com'
driver.get(url)
4、解析HTML内容
使用Selenium可以直接获取网页元素,并提取属性值。
links = driver.find_elements_by_tag_name('a')
for link in links:
href = link.get_attribute('href')
print(href)
5、关闭浏览器
操作完成后,记得关闭浏览器。
driver.quit()
四、不同方法的对比
1、BeautifulSoup:易于使用,适合解析静态HTML文档,但解析速度相对较慢。
2、lxml:解析速度快,适合处理大型HTML文档,但安装和使用复杂度较高。
3、Selenium:可以处理动态加载的内容,适合需要模拟用户操作的场景,但性能较低。
五、示例代码
以下是一个完整的示例代码,展示如何使用BeautifulSoup提取HTML属性值。
from bs4 import BeautifulSoup
import requests
获取网页内容
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
解析HTML内容
soup = BeautifulSoup(html_content, 'html.parser')
提取HTML属性值
for link in soup.find_all('a'):
href = link.get('href')
print(href)
六、总结
在使用Python提取HTML属性值时,选择合适的解析库非常重要。 BeautifulSoup、lxml和Selenium各有优缺点,应根据具体需求选择合适的工具。BeautifulSoup适合解析静态HTML,lxml适合处理大型文档,Selenium适合处理动态内容。 通过合理选择和使用这些工具,可以高效地提取所需的HTML属性值。
七、实践中的注意事项
1、处理异常情况:在实际操作中,可能会遇到各种异常情况,例如网络请求失败、HTML解析错误等。需要添加适当的异常处理机制,提高代码的健壮性。
2、优化性能:对于大规模数据提取任务,可以考虑使用多线程或异步编程技术,提高数据提取速度和效率。
3、合法合规:在进行网页数据提取时,应遵守相关法律法规和网站的robots.txt文件,避免对目标网站造成不必要的负担。
八、案例分析
1、提取商品信息
假设我们要提取某电商网站的商品信息,包括商品名称、价格和链接。可以使用以下代码:
from bs4 import BeautifulSoup
import requests
url = 'https://example-ecommerce.com/products'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
for product in soup.find_all('div', class_='product'):
name = product.find('h2', class_='product-name').text
price = product.find('span', class_='product-price').text
link = product.find('a', class_='product-link').get('href')
print(f'Name: {name}, Price: {price}, Link: {link}')
2、提取新闻标题和链接
假设我们要提取某新闻网站的新闻标题和链接。可以使用以下代码:
from bs4 import BeautifulSoup
import requests
url = 'https://example-news.com'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
for article in soup.find_all('article'):
title = article.find('h2').text
link = article.find('a').get('href')
print(f'Title: {title}, Link: {link}')
九、进阶技巧
1、使用正则表达式提取属性值
有时,我们可能需要使用正则表达式来提取特定格式的属性值。可以结合BeautifulSoup和re库实现:
import re
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
for script in soup.find_all('script', text=re.compile(r'var\s+data\s*=\s*\{')):
script_content = script.text
match = re.search(r'var\s+data\s*=\s*(\{.*?\});', script_content, re.DOTALL)
if match:
data = match.group(1)
print(data)
2、使用CSS选择器提取属性值
BeautifulSoup还支持使用CSS选择器来提取属性值,使用方法如下:
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
for link in soup.select('a[href]'):
href = link['href']
print(href)
十、总结与展望
通过本文的介绍,相信大家已经掌握了使用Python提取HTML属性值的基本方法和进阶技巧。在实际应用中,需要根据具体需求选择合适的解析库,并注意处理异常情况和优化性能。 随着技术的发展,新的解析工具和方法不断涌现,建议大家持续关注和学习相关领域的最新动态。
相关问答FAQs:
1. 如何使用Python从HTML中提取特定标签的属性值?
使用Python提取HTML中的属性值,通常可以使用Beautiful Soup库。首先,安装Beautiful Soup库和requests库。然后,使用requests获取网页内容,接着用Beautiful Soup解析HTML文档,最后通过选择器查找特定标签并获取其属性值。例如:
from bs4 import BeautifulSoup
import requests
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
attribute_value = soup.find('a')['href'] # 获取<a>标签的href属性值
2. 可以通过哪些库来提取HTML属性值?
提取HTML属性值的常用库包括Beautiful Soup、lxml和html.parser。Beautiful Soup易于使用,适合初学者;lxml速度快且功能强大,适合处理复杂的HTML文档;html.parser是Python内置的解析器,适合简单的解析任务。根据具体需求选择合适的库。
3. 提取HTML属性值时需要注意哪些问题?
在提取HTML属性值时,需要确保HTML文档是有效的,避免因标签不完整或格式错误导致解析失败。此外,网页内容可能会动态加载,这时需要使用Selenium等工具来处理JavaScript生成的内容。同时,注意遵守网站的爬虫协议(robots.txt),以免引发法律问题。
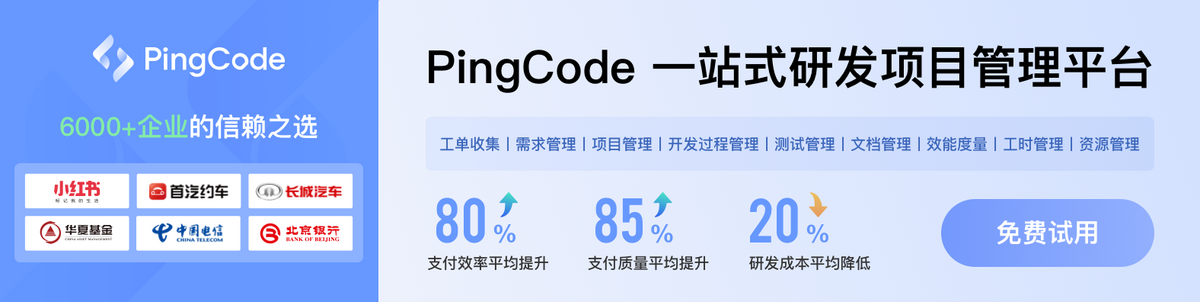