要使用Python爬取页面标题,可以使用几种常见的库,如requests、BeautifulSoup和Selenium。以下是几个步骤的简要概述:首先使用requests获取页面内容,然后使用BeautifulSoup解析HTML并提取标题。你也可以使用Selenium来处理动态加载的页面。以下是详细的介绍。
一、使用requests和BeautifulSoup
requests和BeautifulSoup是Python中非常流行的库,常用于网页抓取任务。requests库用于发送HTTP请求,获取网页的HTML内容,BeautifulSoup则用于解析HTML,并提取所需的信息。以下是使用这两个库爬取页面标题的步骤:
安装requests和BeautifulSoup
在开始之前,需要确保已安装了requests和BeautifulSoup库。可以使用pip安装:
pip install requests
pip install beautifulsoup4
发送HTTP请求并获取页面内容
首先,使用requests库发送HTTP请求,获取网页的HTML内容:
import requests
url = 'https://example.com'
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
html_content = response.text
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
使用BeautifulSoup解析HTML并提取标题
接下来,使用BeautifulSoup库解析HTML内容,并提取页面标题:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
提取页面标题
title = soup.title.string
print(f"Page Title: {title}")
这种方法适用于大多数静态网页,但对于需要动态加载内容的网页,requests和BeautifulSoup可能无法获取所需的信息,这时可以考虑使用Selenium。
二、使用Selenium
Selenium是一个用于自动化Web浏览的工具,支持处理动态加载的网页。它通过控制浏览器执行JavaScript,从而获取动态内容。以下是使用Selenium爬取页面标题的步骤:
安装Selenium和浏览器驱动
首先,安装Selenium库,并下载与浏览器匹配的驱动程序(如ChromeDriver):
pip install selenium
下载ChromeDriver,并将其添加到系统路径中。
使用Selenium控制浏览器并获取页面内容
使用Selenium启动浏览器,加载网页,并获取页面标题:
from selenium import webdriver
启动Chrome浏览器
driver = webdriver.Chrome()
加载网页
url = 'https://example.com'
driver.get(url)
获取页面标题
title = driver.title
print(f"Page Title: {title}")
关闭浏览器
driver.quit()
Selenium适用于处理复杂的网页抓取任务,尤其是那些包含动态内容的页面。
三、综合使用requests、BeautifulSoup和Selenium
在实际应用中,可能需要综合使用requests、BeautifulSoup和Selenium以实现更复杂的网页抓取任务。例如,可以先使用requests获取静态内容,再用BeautifulSoup解析,最后使用Selenium处理动态内容。
结合使用示例
以下是一个结合使用requests、BeautifulSoup和Selenium的示例:
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
url = 'https://example.com'
使用requests获取静态内容
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.title.string
print(f"Static Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
使用Selenium处理动态内容
driver = webdriver.Chrome()
driver.get(url)
dynamic_title = driver.title
print(f"Dynamic Page Title: {dynamic_title}")
driver.quit()
四、处理不同类型的网页
在实际操作中,网页的结构和内容可能会有所不同,需要灵活运用不同的方法来爬取页面标题。要处理不同类型的网页,需要了解网页的结构和加载方式。
处理简单静态网页
对于大多数简单的静态网页,requests和BeautifulSoup已经足够使用。可以通过查看网页的HTML源代码来确定标题的位置,并使用BeautifulSoup提取。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
处理动态加载的网页
对于需要动态加载内容的网页,Selenium是一个很好的选择。可以使用Selenium模拟用户操作,等待页面完全加载后再提取标题。
from selenium import webdriver
driver = webdriver.Chrome()
url = 'https://example.com'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10) # 等待10秒
title = driver.title
print(f"Page Title: {title}")
driver.quit()
处理需要登录的网页
对于需要登录才能访问的网页,可能需要先模拟登录过程,然后再抓取页面内容。可以使用requests库来处理登录,并保存会话信息。
import requests
from bs4 import BeautifulSoup
login_url = 'https://example.com/login'
target_url = 'https://example.com/target-page'
创建一个会话对象
session = requests.Session()
模拟登录
login_data = {
'username': 'your_username',
'password': 'your_password'
}
session.post(login_url, data=login_data)
访问目标页面
response = session.get(target_url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
五、处理反爬虫机制
有些网站会采取反爬虫机制,限制频繁的网页抓取请求。为了避免被封禁IP,可以使用一些技术手段,如设置请求头、使用代理IP和限速。
设置请求头
设置请求头可以模拟浏览器请求,减少被识别为爬虫的风险。
import requests
from bs4 import BeautifulSoup
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
url = 'https://example.com'
response = requests.get(url, headers=headers)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
使用代理IP
使用代理IP可以避免被封禁IP,但需要注意代理IP的质量和稳定性。
import requests
from bs4 import BeautifulSoup
proxies = {
'http': 'http://your_proxy_ip:your_proxy_port',
'https': 'https://your_proxy_ip:your_proxy_port'
}
url = 'https://example.com'
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
控制请求频率
控制请求频率可以避免频繁访问导致的封禁。可以使用time.sleep()函数来实现。
import requests
from bs4 import BeautifulSoup
import time
url = 'https://example.com'
控制请求频率
time.sleep(5) # 等待5秒
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Page Title: {title}")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
六、处理复杂网页结构
有些网页的结构非常复杂,可能包含多个嵌套的标签和动态加载的内容。在这种情况下,需要深入分析网页的结构,并使用适当的方法提取标题。
分析网页结构
可以使用浏览器的开发者工具(如Chrome DevTools)来分析网页的结构,找到标题所在的标签。
提取嵌套的标签
有些网页的标题可能嵌套在多个标签中,可以使用BeautifulSoup的find_all()方法来提取嵌套的标签。
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
title_tag = soup.find_all('title')
if title_tag:
title = title_tag[0].string
print(f"Page Title: {title}")
else:
print("Title tag not found.")
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
处理动态加载的内容
对于动态加载的内容,可以使用Selenium等待页面完全加载后再提取标题。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = webdriver.Chrome()
url = 'https://example.com'
driver.get(url)
等待标题元素出现
try:
title_element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.TAG_NAME, 'title'))
)
title = title_element.get_attribute('innerHTML')
print(f"Page Title: {title}")
finally:
driver.quit()
七、总结
爬取页面标题是网页抓取中的一个基本任务,可以使用requests、BeautifulSoup和Selenium等库来实现。requests和BeautifulSoup适用于静态网页,Selenium适用于动态加载的网页。在实际应用中,可能需要综合使用这些工具,并根据网页的结构和反爬虫机制采取适当的技术手段。
在进行网页抓取时,还需要注意合法合规性,尊重网站的robots.txt文件和使用条款。合理控制抓取频率,避免对目标网站造成负担。
相关问答FAQs:
如何使用Python获取网页的标题?
要使用Python获取网页标题,可以使用requests库下载网页内容,并结合BeautifulSoup库解析HTML。具体步骤包括发送HTTP请求获取网页源码,然后解析该源码,提取
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(title)
在爬取网页标题时需要注意哪些事项?
在爬取网页标题时,需遵循网站的robots.txt协议,以确保不违反其爬虫政策。此外,要注意网站的反爬虫机制,过于频繁的请求可能会导致IP被封禁。因此,建议在爬取时加入适当的延迟和随机化请求头。
如果页面没有
如果页面没有
标签。使用BeautifulSoup可以轻松查找这些标签。例如:
h1 = soup.find('h1')
if h1:
print(h1.string)
else:
print('没有找到标题')
h1 = soup.find('h1')
if h1:
print(h1.string)
else:
print('没有找到标题')
通过这种方式,可以确保获得网页的主要信息,即使页面结构有所不同。
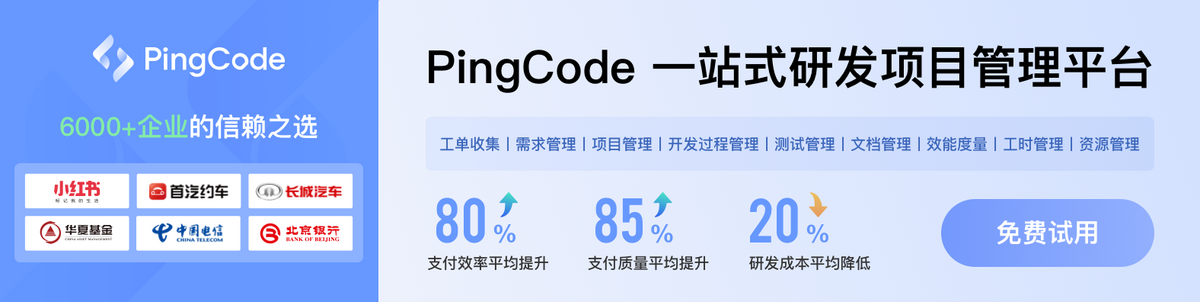