在Vue中实现组件通信传值主要依赖于以下几种方式:父子组件通信、兄弟组件通信、任意组件间通信、通过事件总线(Event Bus)、使用 Vuex。其中,父子组件通信是最常用的方法之一,分为父向子传值和子向父传值。父组件可以通过props向子组件传递数据,而子组件通过$emit向父组件发送消息,从而实现双向通信。
一、父子组件通信
在Vue.js中,实现父子组件通信的核心方法是使用props和$emit。这种方式的通信是单向下行的,即父组件可以向子组件传递数据,但子组件不可以直接修改父组件传递的数据。
父向子传值
父组件通过在子组件标签上绑定属性的方式,传递数据给子组件。子组件通过props选项接收数据。
// 父组件
<template>
<div>
<child-component :parent-msg="parentMsg" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
data() {
return {
parentMsg: 'Hello, child!',
}
}
}
</script>
// 子组件
<template>
<div>{{ parentMsg }}</div>
</template>
<script>
export default {
props: ['parentMsg'],
}
</script>
子向父传值
子组件使用$emit
方法触发事件,并将数据发送给父组件。父组件监听这个事件,并在事件处理函数中接收数据。
// 子组件
<template>
<button @click="sendMsgToParent">Send Message to Parent</button>
</template>
<script>
export default {
methods: {
sendMsgToParent() {
this.$emit('child-msg', 'Hi, parent!');
}
}
}
</script>
// 父组件
<template>
<child-component @child-msg="handleChildMsg" />
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
handleChildMsg(msg) {
console.log(msg); // "Hi, parent!"
}
}
}
</script>
二、兄弟组件通信
实现兄弟组件之间的通信通常需要借助一个共同的父组件作为桥梁,兄弟组件通过父组件来相互传递数据。
数据向上传递
使用$emit
方法将数据从一个兄弟组件传到父组件,然后父组件再通过props将数据传递给另一个兄弟组件。
数据向下传递
父组件可以直接通过props向任何一个子组件传递数据。
三、任意组件间通信
对于非父子关系的组件通信,除了可以通过共同的父组件传递数据外,Vue还提供了事件总线(Event Bus)来实现跨组件的通信。
// event-bus.js
import Vue from 'vue';
export const EventBus = new Vue();
发送事件
// 发送方组件
import { EventBus } from './event-bus.js';
export default {
methods: {
sendMessage() {
EventBus.$emit('message', 'Hello from Component A!');
}
}
}
接收事件
// 接收方组件
import { EventBus } from './event-bus.js';
export default {
mounted() {
EventBus.$on('message', (msg) => {
console.log(msg); // "Hello from Component A!"
});
}
}
四、使用 Vuex
当应用的组件结构变得复杂时,Vuex可以作为一个统一的状态管理库来管理组件间的状态。通过将数据存储在全局单一状态树(store)中,任何组件可以通过提交(mutation)或者派发(action)的方式,来更新全局状态或读取全局状态。
// 在 Vuex store中定义状态和修改状态的方法
const store = new Vuex.Store({
state: {
message: ''
},
mutations: {
updateMessage (state, message) {
state.message = message;
}
}
});
组件中读取全局状态
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
computed: {
message() {
return this.$store.state.message;
}
}
}
</script>
组件中更新全局状态
<template>
<button @click="updateMessage">Update Message</button>
</template>
<script>
export default {
methods: {
updateMessage() {
this.$store.commit('updateMessage', 'New message');
}
}
}
</script>
使用Vuex来管理组件间的通信和状态传递能够有效管理大型项目中各个组件的状态,避免了复杂的组件嵌套或数据传递过程,并使得状态的管理变得清晰和中心化。
相关问答FAQs:
1. 在 Vue 中,你可以使用 props 和 emit 来实现组件之间的通信传值。在父组件中通过 props 将数据传递给子组件,在子组件中通过 emit 发送事件来通知父组件更新数据。这样父子组件之间就可以进行双向的数据传递了。
例如,在父组件中定义一个数据属性 message
,并在子组件中使用 props 来接收该数据:
<!-- 父组件 -->
<template>
<div>
<child-component :message="message" @update-message="updateMessage"></child-component>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello from parent',
};
},
methods: {
updateMessage(newMessage) {
this.message = newMessage;
},
},
};
</script>
<!-- 子组件 -->
<template>
<div>
<p>{{ message }}</p>
<button @click="sendMessage">Send Message to Parent</button>
</div>
</template>
<script>
export default {
props: {
message: String,
},
methods: {
sendMessage() {
this.$emit('update-message', 'Hello from child');
},
},
};
</script>
2. 另一种实现组件通信传值的方式是使用 Vuex,它是 Vue.js 的官方状态管理库。Vuex 允许你在应用的任何地方使用一个共享的状态,并通过在不同组件之间共享该状态来实现组件之间的通信传值。你可以通过在 Store 中定义一个状态 state,并在组件中使用 mapState 函数将该状态映射到组件的计算属性中。这样,当该状态发生变化时,所有使用到该状态的组件都会自动更新。
例如,在定义一个 Vuex Store,设置一个 name 状态,并在两个组件中使用该状态:
// 创建 Store
import Vuex from 'vuex';
import Vue from 'vue';
Vue.use(Vuex);
const store = new Vuex.Store({
state: {
name: 'John Doe',
},
mutations: {
updateName(state, newName) {
state.name = newName;
},
},
});
export default store;
<!-- 组件一 -->
<template>
<div>
<p>{{ name }}</p>
<button @click="changeName">Change Name</button>
</div>
</template>
<script>
import { mapState, mapMutations } from 'vuex';
export default {
computed: {
...mapState(['name']),
},
methods: {
...mapMutations(['updateName']),
changeName() {
this.updateName('Jane Smith');
},
},
};
</script>
<!-- 组件二 -->
<template>
<div>
<p>{{ name }}</p>
</div>
</template>
<script>
import { mapState } from 'vuex';
export default {
computed: {
...mapState(['name']),
},
};
</script>
3. 如果组件之间的通信较为复杂,可以考虑使用事件总线。Vue 提供了一个公共的事件总线对象,它可以用于组件之间的通信。你可以在任何地方通过 $emit
发送一个事件,然后在其他地方通过 $on
监听该事件,并执行相应的操作。这样你就可以轻松地实现组件之间的通信传值。
例如,在创建一个事件总线的实例,然后在两个组件中使用该事件总线对象进行通信:
// 创建事件总线
const bus = new Vue();
// 组件一
export default {
methods: {
changeData() {
bus.$emit('dataChanged', 'New data');
},
},
};
// 组件二
export default {
data() {
return {
receivedData: '',
};
},
created() {
bus.$on('dataChanged', (data) => {
this.receivedData = data;
});
},
};
上面是三种实现 Vue 组件通信传值的方式,根据具体情况选择合适的方式来进行组件间的数据传递。
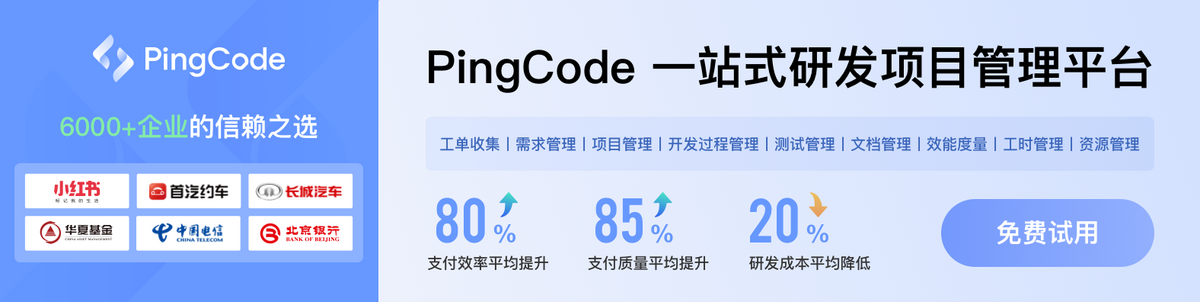