在Python中,重定向可以通过使用标准库中的sys
模块来实现,包括将输出重定向到文件、将错误信息重定向到文件、以及将输入重定向为来自文件的内容。 其中,最常用的重定向是将标准输出从控制台重定向到文件。这可以通过修改sys.stdout
的值实现,从而使print
函数的输出被写入指定的文件中。通过这种方式,可以轻松地将程序的输出结果保存下来,以便后续查看或分析。以下将详细介绍Python中的各种重定向方法。
一、重定向标准输出
在Python中,标准输出通常是指将输出显示到控制台。然而,有时我们希望将输出重定向到文件中,以便保存结果。可以通过以下步骤实现:
-
使用
sys.stdout
重定向输出可以通过修改
sys.stdout
的值来实现输出重定向,将其指向一个文件对象。例如:import sys
打开一个文件用于写入
with open('output.txt', 'w') as file:
# 将标准输出重定向到文件
sys.stdout = file
print("This will be written to the file instead of the console.")
恢复标准输出
sys.stdout = sys.__stdout__
在这段代码中,所有的
print
输出都会被写入到output.txt
文件中,而不是显示在控制台上。完成后,可以将sys.stdout
恢复为默认的标准输出。 -
使用上下文管理器
在Python中使用上下文管理器可以更优雅地处理文件操作和异常情况。可以创建一个自定义的上下文管理器来重定向标准输出:
import sys
from contextlib import contextmanager
@contextmanager
def redirect_stdout(new_target):
old_target = sys.stdout
sys.stdout = new_target
try:
yield
finally:
sys.stdout = old_target
with open('output.txt', 'w') as file:
with redirect_stdout(file):
print("This line is redirected to the file.")
这段代码定义了一个上下文管理器
redirect_stdout
,用于将标准输出重定向到指定的目标。在上下文管理器的范围内,所有的输出都会被重定向,离开该范围后将自动恢复。
二、重定向标准错误
标准错误输出通常用于显示错误信息或警告信息。在某些情况下,我们可能希望将这些信息记录到文件中以便分析。可以通过以下方式实现:
-
使用
sys.stderr
重定向错误输出类似于标准输出,可以通过修改
sys.stderr
的值来重定向错误输出:import sys
打开一个文件用于写入错误信息
with open('error_log.txt', 'w') as file:
# 将标准错误重定向到文件
sys.stderr = file
raise Exception("This error will be logged to the file.")
恢复标准错误
sys.stderr = sys.__stderr__
在这里,
raise Exception
产生的错误信息将被写入到error_log.txt
文件中。 -
使用上下文管理器
同样可以使用上下文管理器来重定向标准错误:
import sys
from contextlib import contextmanager
@contextmanager
def redirect_stderr(new_target):
old_target = sys.stderr
sys.stderr = new_target
try:
yield
finally:
sys.stderr = old_target
with open('error_log.txt', 'w') as file:
with redirect_stderr(file):
raise Exception("This error is redirected to the file.")
这个自定义的上下文管理器
redirect_stderr
可以在上下文中自动重定向标准错误,并在离开上下文时恢复默认设置。
三、重定向标准输入
有时候,我们希望从文件中读取输入数据,而不是从控制台获取。这可以通过重定向标准输入来实现:
-
使用
sys.stdin
重定向输入可以通过修改
sys.stdin
的值,将其指向一个文件对象,以便从文件中读取输入:import sys
打开一个文件用于读取输入
with open('input.txt', 'r') as file:
# 将标准输入重定向到文件
sys.stdin = file
user_input = input("Read from file: ")
print(user_input)
恢复标准输入
sys.stdin = sys.__stdin__
在这段代码中,
input
函数将从input.txt
文件中读取数据,而不是等待用户从控制台输入。 -
使用上下文管理器
同样可以创建一个上下文管理器来重定向标准输入:
import sys
from contextlib import contextmanager
@contextmanager
def redirect_stdin(new_source):
old_source = sys.stdin
sys.stdin = new_source
try:
yield
finally:
sys.stdin = old_source
with open('input.txt', 'r') as file:
with redirect_stdin(file):
user_input = input("Read from file: ")
print(user_input)
这个上下文管理器
redirect_stdin
在上下文内自动重定向标准输入,离开时恢复默认设置。
四、使用subprocess
模块进行重定向
subprocess
模块提供了更强大的功能,用于创建子进程并与其交互。可以使用subprocess
模块将子进程的输入、输出和错误输出重定向。
-
重定向子进程的输出
可以使用
subprocess.run
或subprocess.Popen
来执行命令,并将输出重定向:import subprocess
使用subprocess.run重定向输出
result = subprocess.run(['echo', 'Hello, World!'], stdout=subprocess.PIPE)
print(result.stdout.decode())
使用subprocess.Popen重定向输出
process = subprocess.Popen(['echo', 'Hello, World!'], stdout=subprocess.PIPE)
stdout, stderr = process.communicate()
print(stdout.decode())
在这两个示例中,
stdout
被重定向到管道中,可以通过result.stdout
或process.communicate()
来获取输出。 -
重定向子进程的输入
可以通过
subprocess.Popen
将输入重定向到子进程:import subprocess
使用subprocess.Popen重定向输入
process = subprocess.Popen(['python', 'script.py'], stdin=subprocess.PIPE)
process.communicate(input=b'Input data for script\n')
在这里,
input
数据被发送到子进程的标准输入。 -
重定向子进程的错误输出
可以通过
subprocess.run
或subprocess.Popen
将错误输出重定向:import subprocess
使用subprocess.run重定向错误输出
result = subprocess.run(['python', '-c', 'import sys; sys.stderr.write("Error message\n")'], stderr=subprocess.PIPE)
print(result.stderr.decode())
使用subprocess.Popen重定向错误输出
process = subprocess.Popen(['python', '-c', 'import sys; sys.stderr.write("Error message\n")'], stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
print(stderr.decode())
在这两个示例中,
stderr
被重定向到管道中,可以通过result.stderr
或process.communicate()
来获取错误输出。
通过上述方法,可以在Python中实现标准输入、输出和错误输出的重定向,从而更灵活地处理数据流。这种能力在编写复杂程序、调试代码和处理大量数据时非常有用。
相关问答FAQs:
如何在Python中实现标准输出的重定向?
在Python中,可以通过sys
模块来实现标准输出的重定向。使用sys.stdout
可以将输出流重定向到文件或其他对象。例如,打开一个文件并将其赋值给sys.stdout
,之后所有的打印操作将会输出到该文件中。代码示例:
import sys
# 重定向标准输出到文件
with open('output.txt', 'w') as f:
sys.stdout = f
print("这行将被写入文件中")
# 恢复标准输出
sys.stdout = sys.__stdout__
print("这行将显示在控制台中")
在Python中如何重定向错误输出?
Python同样允许你重定向标准错误输出(stderr)。使用sys.stderr
可以实现这一点,方法与重定向标准输出相似。通过将sys.stderr
指向一个文件,你可以捕捉并记录错误信息。示例代码如下:
import sys
# 重定向标准错误到文件
with open('error_log.txt', 'w') as error_file:
sys.stderr = error_file
print("发生错误", file=sys.stderr)
# 恢复标准错误输出
sys.stderr = sys.__stderr__
print("这条消息将显示在控制台")
如何在Python中使用上下文管理器进行输出重定向?
上下文管理器提供了一种优雅的方式来处理资源的管理,包括输出的重定向。通过自定义上下文管理器,可以轻松地在特定代码块中进行输出的重定向。以下是一个简单的示例:
import sys
class RedirectOutput:
def __init__(self, new_stdout):
self.new_stdout = new_stdout
self.old_stdout = sys.stdout
def __enter__(self):
sys.stdout = self.new_stdout
def __exit__(self, exc_type, exc_value, traceback):
sys.stdout = self.old_stdout
with open('redirected_output.txt', 'w') as f:
with RedirectOutput(f):
print("这个输出会被重定向到文件中")
print("这个输出会显示在控制台")
这种方式让代码更加整洁,并且能够在需要的时候方便地控制输出的流向。
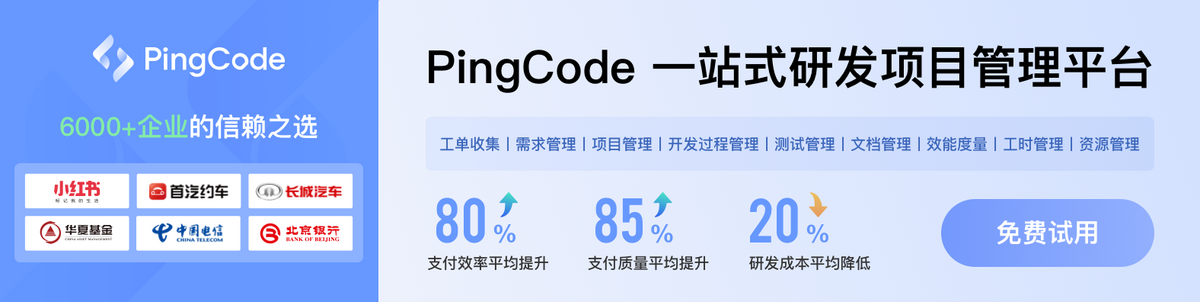