开头段落:
使用Python打开Juniper设备可以通过多种方法实现,包括使用Netmiko库、Paramiko库、以及通过REST API进行交互。Netmiko是一个专为网络设备设计的Python库,能够简化与设备的SSH连接和操作。使用Netmiko,您可以通过SSH协议直接连接到Juniper设备,并执行命令来获取信息或配置设备。此外,Paramiko是一个低级别的SSH库,可以提供更细粒度的控制和自定义功能。REST API则允许您通过HTTP请求与Juniper设备进行交互,这种方法尤其适用于自动化和大规模设备管理。
一、NETMIKO库的使用
Netmiko是一个专门用于网络设备的Python库,基于Paramiko构建,提供了更高级别的接口,便于与网络设备进行交互。
1. 安装Netmiko
要使用Netmiko库,首先需要安装它。可以通过Python的包管理工具pip进行安装:
pip install netmiko
2. 基本连接
使用Netmiko连接到Juniper设备非常简单。以下是一个基本的连接示例:
from netmiko import ConnectHandler
juniper_device = {
'device_type': 'juniper',
'ip': '192.168.1.1',
'username': 'admin',
'password': 'password',
}
connection = ConnectHandler(juniper_device)
output = connection.send_command('show version')
print(output)
connection.disconnect()
以上代码展示了如何通过Netmiko连接到Juniper设备,并执行show version
命令获取设备版本信息。
3. 批量命令执行
Netmiko还支持批量执行命令,这对于需要执行多个命令的场景非常有用:
commands = ['show version', 'show interfaces terse']
for command in commands:
output = connection.send_command(command)
print(output)
使用Netmiko的批量执行功能,可以提高配置和管理效率。
二、PARAMIKO库的使用
Paramiko是一个底层的SSH库,适用于需要更细粒度控制的场景。它提供了对SSH协议的全面支持。
1. 安装Paramiko
同样需要先安装Paramiko库:
pip install paramiko
2. 基本连接
使用Paramiko进行SSH连接稍微复杂一些,但也提供了更大的灵活性:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('192.168.1.1', username='admin', password='password')
stdin, stdout, stderr = ssh.exec_command('show version')
print(stdout.read().decode())
ssh.close()
Paramiko允许直接执行SSH命令,并获取输出结果。
3. 使用SCP传输文件
Paramiko还支持使用SCP协议进行文件传输:
from paramiko import SSHClient
from scp import SCPClient
ssh = SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('192.168.1.1', username='admin', password='password')
with SCPClient(ssh.get_transport()) as scp:
scp.put('local_file.txt', 'remote_file.txt')
scp.get('remote_file.txt', 'local_file.txt')
ssh.close()
通过SCP,您可以轻松地在本地与远程设备之间传输文件。
三、REST API的使用
Juniper设备支持REST API,通过HTTP请求实现设备管理和配置。
1. REST API简介
REST API是一种基于HTTP协议的接口标准,允许使用HTTP方法(GET、POST、PUT、DELETE)与设备进行交互。
2. 安装请求库
要使用REST API,通常需要Python的requests库:
pip install requests
3. 发送GET请求
以下是如何通过REST API获取设备信息的示例:
import requests
from requests.auth import HTTPBasicAuth
url = 'https://192.168.1.1/rest/api/v1/system/version'
response = requests.get(url, auth=HTTPBasicAuth('admin', 'password'), verify=False)
print(response.json())
通过GET请求可以获取设备的版本信息,这种方法尤其适合自动化任务。
4. 发送POST请求
POST请求通常用于更改设备配置:
url = 'https://192.168.1.1/rest/api/v1/configuration'
headers = {'Content-Type': 'application/json'}
data = {
"configuration": {
"interfaces": {
"ge-0/0/0": {
"unit": "0",
"family": "inet",
"address": "192.168.1.2/24"
}
}
}
}
response = requests.post(url, json=data, headers=headers, auth=HTTPBasicAuth('admin', 'password'), verify=False)
print(response.status_code)
使用POST请求可以向设备发送配置数据,从而实现配置更新。
四、自动化脚本的设计
将以上方法结合,可以设计更复杂的自动化脚本,提高管理效率。
1. 自动化设备配置
可以编写一个自动化脚本,定期检查设备配置,并根据需要进行更新:
def update_configuration(device_ip, username, password):
try:
connection = ConnectHandler(device_type='juniper', ip=device_ip, username=username, password=password)
connection.send_command('set system services ssh')
connection.commit()
connection.disconnect()
print(f'Configuration updated for {device_ip}')
except Exception as e:
print(f'Failed to update configuration for {device_ip}: {e}')
2. 批量设备管理
对于多台设备,可以批量执行配置更新:
devices = [
{'ip': '192.168.1.1', 'username': 'admin', 'password': 'password'},
{'ip': '192.168.1.2', 'username': 'admin', 'password': 'password'},
]
for device in devices:
update_configuration(device['ip'], device['username'], device['password'])
这种批量管理方式在大规模网络环境中尤为有效。
五、安全性和最佳实践
在使用Python与Juniper设备交互时,安全性是一个重要的考虑因素。
1. 使用加密连接
无论是使用SSH还是REST API,确保连接是加密的,以防止中间人攻击和数据泄露。
2. 管理凭据
不要在代码中硬编码用户名和密码,应该使用环境变量或配置文件来管理凭据。
3. 日志记录
记录所有操作日志,以便在出现问题时进行审计和故障排除。
通过遵循这些最佳实践,可以提高与Juniper设备交互的安全性和可靠性。
相关问答FAQs:
如何在Python中连接Juniper设备?
要在Python中连接Juniper设备,可以使用Netmiko或Paramiko等库。Netmiko是一个专门为网络设备设计的库,支持多种设备,包括Juniper。在使用Netmiko时,需要提供设备的IP地址、用户名、密码和设备类型。示例代码如下:
from netmiko import ConnectHandler
juniper_device = {
'device_type': 'juniper',
'host': '192.168.1.1',
'username': 'your_username',
'password': 'your_password',
}
connection = ConnectHandler(**juniper_device)
output = connection.send_command('show version')
print(output)
connection.disconnect()
这样就可以成功连接并执行命令。
在Python中如何处理Juniper设备的配置?
使用Python处理Juniper设备的配置可以通过Netmiko或Nornir等库来实现。通过这些库,可以发送配置命令并获取设备的反馈。确保在发送配置命令时使用send_config_set
方法,它可以将一系列配置命令批量发送到设备。示例代码如下:
commands = [
'set system host-name new-hostname',
'set interfaces ge-0/0/0 description "Uplink to Router"',
]
output = connection.send_config_set(commands)
print(output)
这样就能对Juniper设备进行相应的配置。
使用Python与Juniper设备进行故障排除的最佳实践是什么?
在进行故障排除时,建议使用Python脚本自动化收集设备信息。可以使用send_command
方法获取接口状态、路由表等关键信息。将这些信息记录到日志文件中,以便后续分析。此外,考虑使用异常处理来捕获连接或命令执行中的错误,以提高脚本的稳定性。示例代码如下:
try:
connection = ConnectHandler(**juniper_device)
output = connection.send_command('show interfaces terse')
with open('interface_status.log', 'w') as log_file:
log_file.write(output)
except Exception as e:
print(f"An error occurred: {e}")
finally:
connection.disconnect()
此方法有助于快速识别和解决网络问题。
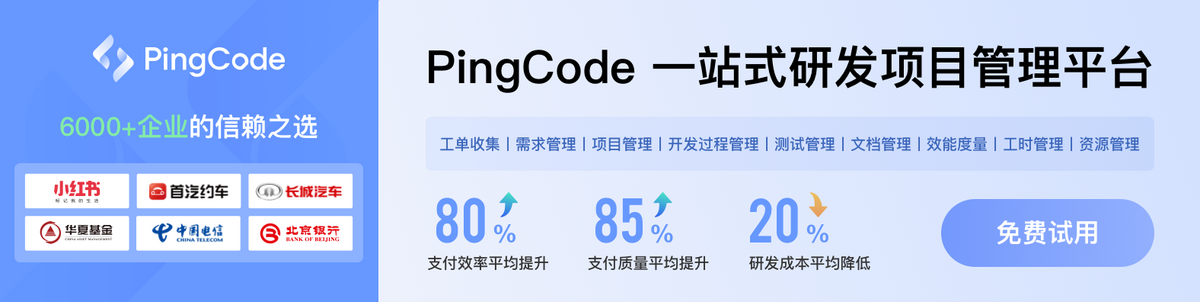