Python可以通过多种方式实现生日祝福,其中包括编写简单的命令行程序、创建图形用户界面(GUI)应用以及发送电子邮件或短信来实现。其中,使用Python的smtplib库发送电子邮件是一种常用的方式。接下来,我们将详细探讨如何利用Python实现生日祝福的不同方法。
一、命令行程序实现生日祝福
- 编写一个简单的命令行程序
Python可以用来编写一个简单的命令行程序,用户可以输入名字和生日,然后程序会输出一条生日祝福信息。例如:
def birthday_wish(name, birthday):
return f"Happy Birthday, {name}! Wishing you a fantastic day on {birthday}!"
name = input("Enter the name: ")
birthday = input("Enter the birthday (MM-DD): ")
print(birthday_wish(name, birthday))
通过这种方式,我们可以快速生成一条个性化的生日祝福信息。
- 使用条件语句增加个性化
为了让生日祝福更加个性化,可以根据用户输入的年龄或星座,输出不同的祝福信息:
def birthday_wish(name, age):
if age < 18:
return f"Happy Birthday, {name}! Enjoy your youthful days!"
elif 18 <= age < 30:
return f"Happy Birthday, {name}! Here's to exciting adventures ahead!"
else:
return f"Happy Birthday, {name}! Wishing you a wonderful year filled with joy!"
name = input("Enter the name: ")
age = int(input("Enter the age: "))
print(birthday_wish(name, age))
二、创建图形用户界面(GUI)应用
- 使用Tkinter库
Tkinter是Python的标准GUI库,可以用来创建桌面应用程序。我们可以使用Tkinter来制作一个简单的生日祝福应用:
import tkinter as tk
def show_wish():
name = entry_name.get()
message = f"Happy Birthday, {name}!"
label_wish.config(text=message)
app = tk.Tk()
app.title("Birthday Wish")
label_name = tk.Label(app, text="Enter your name:")
label_name.pack()
entry_name = tk.Entry(app)
entry_name.pack()
button_wish = tk.Button(app, text="Get Birthday Wish", command=show_wish)
button_wish.pack()
label_wish = tk.Label(app, text="")
label_wish.pack()
app.mainloop()
这个程序创建了一个简单的窗口,用户可以输入名字并点击按钮来获得生日祝福。
- 增加更多功能
可以在GUI应用中加入更多功能,比如选择生日蛋糕的图片或播放生日歌:
from PIL import ImageTk, Image
import tkinter as tk
def show_wish():
name = entry_name.get()
message = f"Happy Birthday, {name}!"
label_wish.config(text=message)
img = Image.open("birthday_cake.jpg")
img = img.resize((250, 250), Image.ANTIALIAS)
img = ImageTk.PhotoImage(img)
label_img.config(image=img)
label_img.image = img
app = tk.Tk()
app.title("Birthday Wish")
label_name = tk.Label(app, text="Enter your name:")
label_name.pack()
entry_name = tk.Entry(app)
entry_name.pack()
button_wish = tk.Button(app, text="Get Birthday Wish", command=show_wish)
button_wish.pack()
label_wish = tk.Label(app, text="")
label_wish.pack()
label_img = tk.Label(app)
label_img.pack()
app.mainloop()
三、通过电子邮件发送生日祝福
- 使用smtplib库发送电子邮件
Python的smtplib库可以用来发送电子邮件,我们可以利用它来发送生日祝福:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_birthday_wish(email, name):
sender_email = "your_email@example.com"
receiver_email = email
password = "your_password"
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = "Happy Birthday!"
body = f"Happy Birthday, {name}! Wishing you a fantastic day!"
message.attach(MIMEText(body, "plain"))
with smtplib.SMTP("smtp.example.com", 587) as server:
server.starttls()
server.login(sender_email, password)
server.send_message(message)
email = input("Enter the recipient's email: ")
name = input("Enter the recipient's name: ")
send_birthday_wish(email, name)
- 添加HTML格式
为了让邮件看起来更美观,可以使用HTML格式的邮件:
from email.mime.text import MIMEText
def send_birthday_wish(email, name):
# ... (other parts remain the same)
html = f"""\
<html>
<body>
<h1>Happy Birthday, {name}!</h1>
<p>Wishing you a fantastic day!</p>
</body>
</html>
"""
message.attach(MIMEText(html, "html"))
# ... (send email as before)
四、通过短信发送生日祝福
- 使用Twilio API
Twilio是一个云通讯平台,可以用来发送短信。我们可以利用Twilio的API来发送生日祝福短信:
from twilio.rest import Client
def send_birthday_sms(to_number, name):
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=f"Happy Birthday, {name}! Have a great day!",
from_='+1234567890',
to=to_number
)
to_number = input("Enter the recipient's phone number: ")
name = input("Enter the recipient's name: ")
send_birthday_sms(to_number, name)
- 定时发送短信
为了在生日当天自动发送祝福,可以使用Python的schedule库来安排任务:
import schedule
import time
def job():
# Call the send_birthday_sms function with appropriate parameters
schedule.every().day.at("09:00").do(job)
while True:
schedule.run_pending()
time.sleep(60)
以上是如何用Python实现生日祝福的几种方法。通过这些代码示例,您可以创建一个个性化的生日祝福程序,从简单的命令行工具到复杂的GUI应用或自动化的邮件和短信发送工具。希望这些示例能帮助您更好地理解如何利用Python来实现生日祝福。
相关问答FAQs:
如何使用Python发送生日祝福?
可以通过编写一个简单的Python程序,使用内置的datetime
模块来获取当前日期,并检查是否与朋友的生日相符。如果匹配,可以使用smptlib
库发送电子邮件祝福,或者使用twilio
等API发送短信祝福。程序也可以设置定时任务在特定日期自动运行。
有哪些库可以帮助我实现生日祝福的自动发送?
在Python中,smtplib
库非常适合发送电子邮件,twilio
库则可以用来发送短信。此外,schedule
库可以帮助你设置定时任务,使得生日祝福可以在特定时间自动发送。此外,使用pandas
可以方便地管理和读取生日信息,提升程序的灵活性。
我可以如何个性化我的生日祝福内容?
可以通过定义一个包含多种祝福语的列表,根据接收者的年龄或喜好随机选择祝福内容。还可以添加个性化信息,如接收者的名字、过去的美好回忆等。此外,使用Python的random
库可以使每次发送的祝福都不尽相同,增加惊喜感。
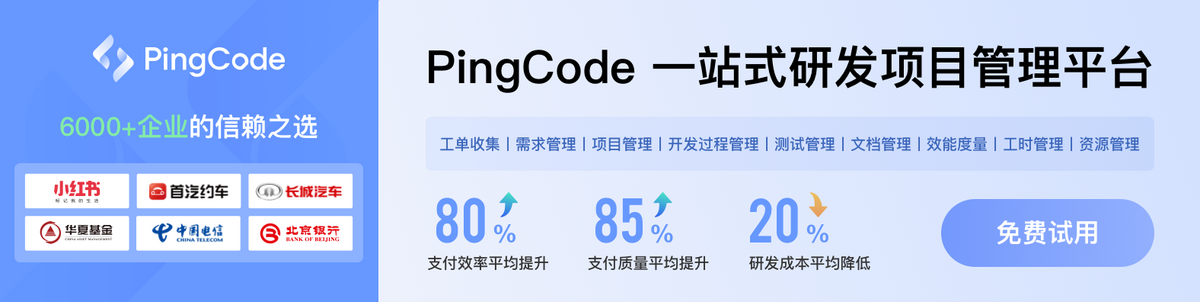