Python中可以通过字典推导式、filter函数、字典的方法(如items、keys、values)来过滤字典内容。其中,字典推导式是最常用的方法,因为它的语法简洁且直观。在这篇文章中,我们将详细讨论这些方法,并提供示例代码来帮助您更好地理解如何在Python中过滤字典内容。
一、字典推导式
字典推导式是一种非常强大的工具,可以用于创建和过滤字典。通过字典推导式,我们可以根据条件生成一个新的字典。
1.1、使用字典推导式过滤字典
字典推导式的基本语法如下:
{key: value for key, value in original_dict.items() if condition}
通过这种方式,我们可以根据需要的条件过滤字典中的键值对。例如,假设我们有一个字典,其中包含学生的名字及其分数,我们想过滤出所有分数大于70的学生:
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
filtered_students = {name: score for name, score in students.items() if score > 70}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
在这个例子中,我们通过字典推导式过滤出了所有分数大于70的学生。
1.2、结合多个条件过滤
字典推导式不仅可以用于单一条件过滤,还可以结合多个条件进行复杂的过滤操作。假设我们希望过滤出分数在70到90之间的学生:
filtered_students = {name: score for name, score in students.items() if 70 < score < 90}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
通过这种方式,我们可以根据多个条件过滤字典内容,从而获取更精确的结果。
二、filter函数
filter函数是Python的内置函数,可以用于过滤任何可迭代对象。虽然filter函数主要用于过滤列表,但我们也可以将其用于字典的过滤。
2.1、使用filter函数过滤字典
要使用filter函数过滤字典,我们首先需要将字典转换为一个可迭代的列表,然后应用filter函数,最后再将结果转换回字典。以下是一个示例:
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
def is_passing(student):
return student[1] > 70
filtered_students = dict(filter(is_passing, students.items()))
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
在这个例子中,我们定义了一个函数is_passing
,用于判断学生的分数是否大于70。然后,我们使用filter函数过滤出满足条件的键值对,最后将结果转换为字典。
2.2、使用lambda表达式
filter函数常常与lambda表达式结合使用,以便更简洁地表达过滤条件。以下是使用lambda表达式的示例:
filtered_students = dict(filter(lambda student: student[1] > 70, students.items()))
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
通过这种方式,我们可以在一行代码中完成过滤操作,提高代码的简洁性和可读性。
三、字典的方法(items、keys、values)
除了字典推导式和filter函数外,我们还可以使用字典的内置方法来过滤字典内容。这些方法包括items、keys和values。
3.1、使用items方法
items方法返回一个包含字典所有键值对的视图对象。我们可以使用items方法结合列表推导式或其他迭代工具来过滤字典。
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
filtered_students = dict((name, score) for name, score in students.items() if score > 70)
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
在这个例子中,我们通过items方法获取字典的键值对,然后使用生成器表达式进行过滤。
3.2、使用keys方法
keys方法返回一个包含字典所有键的视图对象。我们可以使用keys方法结合字典推导式来过滤字典的键。
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
filtered_students = {name: students[name] for name in students.keys() if students[name] > 70}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
通过这种方式,我们可以根据键的某些特性进行过滤。
3.3、使用values方法
values方法返回一个包含字典所有值的视图对象。虽然values方法主要用于获取字典的值,但我们仍然可以在过滤操作中使用它。
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
filtered_students = {name: score for name, score in students.items() if score in students.values() and score > 70}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
在这个例子中,我们使用values方法结合字典推导式来过滤字典的值。
四、结合其他数据结构进行过滤
在实际应用中,我们可能需要结合其他数据结构(如列表、集合)进行更加复杂的字典过滤。
4.1、结合列表
假设我们有一个包含学生姓名的列表,我们希望过滤出字典中这些学生的信息:
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
student_names = ['Alice', 'Charlie']
filtered_students = {name: students[name] for name in student_names if name in students}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
通过这种方式,我们可以根据列表中的信息来过滤字典。
4.2、结合集合
集合(set)是另一种常用的数据结构,可以用于高效地检查元素是否存在于集合中。我们可以结合集合来过滤字典。
students = {'Alice': 85, 'Bob': 65, 'Charlie': 75, 'David': 50}
passing_students = {'Alice', 'Charlie'}
filtered_students = {name: score for name, score in students.items() if name in passing_students}
print(filtered_students)
Output: {'Alice': 85, 'Charlie': 75}
在这个例子中,我们使用集合来存储通过的学生姓名,然后根据集合过滤字典。
五、实际应用场景
在实际应用中,字典过滤操作可以用于各种场景,如数据清洗、数据分析、配置管理等。
5.1、数据清洗
数据清洗是数据分析的重要步骤之一。在数据清洗过程中,我们可能需要从数据集中移除不符合条件的记录。字典过滤操作可以帮助我们实现这一目标。
data = {
'record1': {'name': 'Alice', 'age': 25, 'score': 85},
'record2': {'name': 'Bob', 'age': 22, 'score': 65},
'record3': {'name': 'Charlie', 'age': 23, 'score': 75},
}
cleaned_data = {key: value for key, value in data.items() if value['score'] > 70}
print(cleaned_data)
Output: {'record1': {'name': 'Alice', 'age': 25, 'score': 85}, 'record3': {'name': 'Charlie', 'age': 23, 'score': 75}}
5.2、数据分析
在数据分析过程中,我们通常需要根据某些条件对数据进行分组和过滤,从而获取更有意义的结果。
data = {
'Alice': {'math': 85, 'science': 78},
'Bob': {'math': 65, 'science': 68},
'Charlie': {'math': 75, 'science': 82},
}
math_above_70 = {name: scores for name, scores in data.items() if scores['math'] > 70}
print(math_above_70)
Output: {'Alice': {'math': 85, 'science': 78}, 'Charlie': {'math': 75, 'science': 82}}
5.3、配置管理
在配置管理中,我们可能需要根据某些条件过滤配置项,以便进行动态配置或更新。
config = {
'feature1': True,
'feature2': False,
'feature3': True,
}
enabled_features = {key: value for key, value in config.items() if value}
print(enabled_features)
Output: {'feature1': True, 'feature3': True}
通过这些实际应用场景,我们可以看到字典过滤在各个领域的广泛应用。无论是数据清洗、数据分析还是配置管理,字典过滤都可以帮助我们更高效地处理和管理数据。
总结
在这篇文章中,我们讨论了Python中过滤字典内容的多种方法,包括字典推导式、filter函数、字典的方法以及结合其他数据结构进行过滤。每种方法都有其独特的优势和适用场景,可以根据具体需求选择合适的方法进行字典过滤。通过对这些方法的掌握,您将能够更灵活地处理和管理字典数据,提高代码的可读性和效率。希望这篇文章能为您在Python编程中提供有价值的指导和帮助。
相关问答FAQs:
如何使用Python过滤字典中的特定键?
在Python中,可以使用字典推导式来过滤字典中的特定键。通过指定想要保留的键列表,可以轻松创建一个新的字典。例如,如果你有一个字典my_dict = {'a': 1, 'b': 2, 'c': 3}
,并想保留键'a'
和'b'
,可以使用以下代码:
filtered_dict = {k: my_dict[k] for k in ['a', 'b'] if k in my_dict}
这将创建一个新的字典{'a': 1, 'b': 2}
。
在Python中如何根据值过滤字典?
如果需要根据字典中的值进行过滤,可以同样利用字典推导式。假设你有一个字典my_dict = {'a': 1, 'b': 2, 'c': 3}
,想要保留所有值大于1的键值对,可以使用如下代码:
filtered_dict = {k: v for k, v in my_dict.items() if v > 1}
这样会得到一个新的字典{'b': 2, 'c': 3}
。
如何使用Lambda函数过滤字典?
如果你希望使用更灵活的过滤条件,Lambda函数是一个很好的选择。可以结合filter()
和dict()
函数来实现。例如,考虑一个字典my_dict = {'a': 1, 'b': 2, 'c': 3}
,想要保留所有值是偶数的项,可以这样做:
filtered_dict = dict(filter(lambda item: item[1] % 2 == 0, my_dict.items()))
这将返回一个字典{'b': 2}
,只包含符合条件的项。
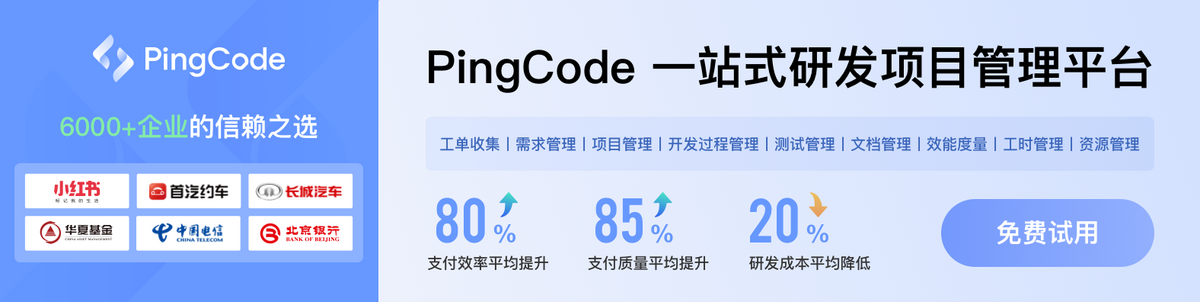