在Python中导入parser模块涉及以下几个步骤:选择合适的解析库、安装所需库、编写代码导入并使用解析功能。在Python中,解析器的选择取决于具体的需求,如解析XML、JSON、HTML或其他数据格式。以下是关于如何在Python中导入和使用解析器的详细说明。
一、选择合适的解析库
在Python中,有多种用于解析不同数据格式的库。以下是一些常用的解析库:
- XML解析库:Python标准库中自带
xml.etree.ElementTree
,以及更为强大的第三方库lxml
。 - JSON解析库:Python自带的
json
库足以应对大多数JSON解析需求。 - HTML解析库:
BeautifulSoup
(需要安装bs4包)和lxml
都是非常流行的选择。 - 通用解析库:
argparse
用于解析命令行参数。
对于解析XML,可以选择xml.etree.ElementTree
或lxml
。ElementTree
是Python自带的库,无需安装即可使用,而lxml
提供了更强大的功能和更好的性能。
二、安装所需库
对于标准库中的解析器,不需要进行额外安装。对于第三方库,如lxml
或BeautifulSoup
,需要通过pip进行安装:
pip install lxml
pip install beautifulsoup4
三、编写代码导入并使用解析功能
以下是如何在Python中导入和使用不同解析器的示例:
1. XML解析器
使用xml.etree.ElementTree
解析XML:
import xml.etree.ElementTree as ET
xml_data = '''<root>
<child name="child1">Child 1 text</child>
<child name="child2">Child 2 text</child>
</root>'''
root = ET.fromstring(xml_data)
for child in root:
print(child.tag, child.attrib, child.text)
使用lxml
解析XML:
from lxml import etree
xml_data = '''<root>
<child name="child1">Child 1 text</child>
<child name="child2">Child 2 text</child>
</root>'''
root = etree.fromstring(xml_data)
for child in root:
print(child.tag, child.attrib, child.text)
2. JSON解析器
Python自带的json
库解析JSON:
import json
json_data = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_data)
print(data['name'], data['age'], data['city'])
3. HTML解析器
使用BeautifulSoup
解析HTML:
from bs4 import BeautifulSoup
html_data = '''<html>
<head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>'''
soup = BeautifulSoup(html_data, 'html.parser')
print(soup.title.string)
print(soup.find_all('a'))
4. 命令行参数解析器
使用argparse
解析命令行参数:
import argparse
parser = argparse.ArgumentParser(description='Process some integers.')
parser.add_argument('integers', metavar='N', type=int, nargs='+', help='an integer for the accumulator')
parser.add_argument('--sum', dest='accumulate', action='store_const', const=sum, default=max, help='sum the integers (default: find the max)')
args = parser.parse_args()
print(args.accumulate(args.integers))
总结:
在Python中使用解析器的关键在于选择适合的库并正确导入和使用。在选择解析库时,应根据具体的数据格式和项目需求来选择合适的库。通过合理使用解析器,可以有效地处理和转换数据,提升程序的效率和功能。
相关问答FAQs:
如何在Python中导入parser模块?
在Python中,parser模块是一个用于解析字符串和处理语法的标准库。要导入parser模块,只需在你的Python代码中使用import parser
。在导入后,你可以使用模块中的各种函数和类来处理文本解析任务。
parser模块有哪些常用功能?
parser模块提供了多种功能,包括解析Python源代码、处理语法树以及对Python表达式进行评估。通过使用parser.expr()
函数,你可以将字符串形式的Python表达式转换为解析树。此外,parser还支持自定义的语法解析,可以帮助开发者处理特定格式的数据。
是否有替代parser模块的其他选项?
是的,Python中有许多其他库可以用于解析任务。例如,lxml
和BeautifulSoup
非常适合处理HTML和XML文档,而json
模块可以帮助解析JSON格式的数据。如果你的需求更复杂,可以考虑使用ANTLR
或PLY
等工具,它们能够提供更强大的语法分析功能。
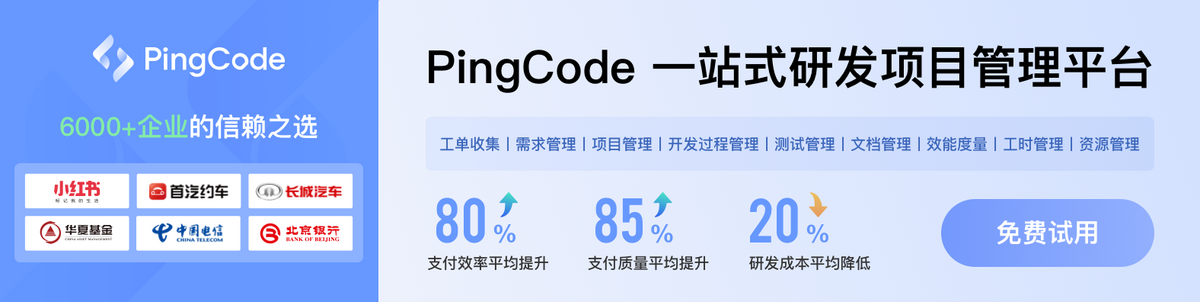