Python 和 Shell 脚本可以结合使用,通过Python调用Shell命令、在Shell中调用Python脚本、使用subprocess模块执行Shell命令。其中,subprocess模块是一个非常强大的工具,它允许你在Python中执行Shell命令并获取结果。通过这种方式,你可以在Python脚本中执行复杂的Shell操作,然后将结果用于进一步的数据处理或分析。下面我将详细介绍这几种方法。
一、PYTHON调用SHELL命令
1. 使用os模块
Python的os模块提供了一些基本的操作系统接口,其中的os.system()
函数可以用来执行Shell命令。
import os
执行一个简单的Shell命令
os.system('ls -l')
虽然os.system()
简单易用,但它有一些缺点,比如无法获取命令的输出结果。因此,不推荐在需要处理Shell命令输出的场景下使用。
2. 使用subprocess模块
subprocess
模块是Python中执行Shell命令的推荐方式,它不仅可以执行命令,还可以捕获输出、错误信息,以及设置输入。
import subprocess
执行Shell命令并获取输出
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在这里,capture_output=True
用于捕获命令的标准输出和标准错误,text=True
表示将输出作为字符串而不是字节返回。
3. 使用subprocess模块的Popen类
对于需要更复杂的交互或者需要处理标准输入输出的情况,可以使用subprocess.Popen
类。
import subprocess
使用Popen执行Shell命令
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print('标准输出:', stdout)
print('标准错误:', stderr)
Popen
提供了更灵活的方式来处理Shell命令的输入输出流,适合需要复杂操作的场景。
二、在SHELL中调用PYTHON脚本
1. 直接调用
如果Python脚本文件具有执行权限,可以在Shell中直接调用。
# 赋予Python脚本执行权限
chmod +x script.py
在Shell中执行Python脚本
./script.py
2. 使用python命令
即使没有执行权限,也可以通过python
或者python3
命令来执行Python脚本。
# 使用python命令执行脚本
python3 script.py
3. 在Shell脚本中嵌入Python代码
有时你可能希望在Shell脚本中嵌入Python代码,可以使用以下方法:
#!/bin/bash
Shell命令
echo "This is a Shell script."
Python代码
python3 - <<END
print("This is Python code executed within a Shell script.")
END
这种方式可以让你在Shell脚本中直接嵌入并执行Python代码,非常适合需要使用Python处理复杂数据的场景。
三、使用SUBPROCESS模块执行SHELL命令
1. 处理命令输出
subprocess.run()
可以捕获命令输出,将其用于进一步处理。
import subprocess
def list_files():
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
files = result.stdout.splitlines()
for file in files:
print(file)
list_files()
在这个例子中,我们使用subprocess.run()
获取了ls -l
命令的输出,并将其逐行打印出来。
2. 处理标准错误
除了标准输出,你也可以处理标准错误。
import subprocess
def run_command(command):
result = subprocess.run(command, capture_output=True, text=True, shell=True)
if result.returncode != 0:
print('Error:', result.stderr)
else:
print('Output:', result.stdout)
run_command('ls non_existent_file')
在这里,ls non_existent_file
会产生错误,我们通过检查returncode
和stderr
来处理这个错误。
3. 使用管道
subprocess.Popen
允许你使用管道将多个命令连接在一起。
import subprocess
def run_piped_commands():
p1 = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, text=True)
p2 = subprocess.Popen(['grep', 'py'], stdin=p1.stdout, stdout=subprocess.PIPE, text=True)
p1.stdout.close()
output, _ = p2.communicate()
print('Filtered Output:', output)
run_piped_commands()
这里,我们使用管道将ls -l
的输出传递给grep py
命令,实现了输出过滤。
四、结合使用的场景
1. 数据处理
在数据处理的场景中,Python通常用于复杂的数据分析,而Shell则用于文件操作和系统管理。通过结合使用,你可以利用各自的优势来提高效率。
import subprocess
def clean_and_analyze_data(file_path):
# 使用Shell命令清理数据
subprocess.run(['sed', '-i', '/^#/d', file_path], check=True)
# 使用Python分析数据
with open(file_path, 'r') as file:
data = file.readlines()
# 简单分析
print('Number of data lines:', len(data))
clean_and_analyze_data('data.txt')
2. 自动化任务
自动化任务中,你可以使用Python来执行逻辑操作,而Shell则用于调度和执行系统命令。
import os
import subprocess
def backup_files():
# 使用Shell命令创建备份目录
os.makedirs('backup', exist_ok=True)
# 使用Python逻辑决定哪些文件需要备份
files_to_backup = [f for f in os.listdir('.') if f.endswith('.txt')]
for file in files_to_backup:
subprocess.run(['cp', file, 'backup/'], check=True)
backup_files()
3. 网络操作
结合使用Python和Shell可以有效地处理网络操作,比如下载文件、解析数据等。
import subprocess
import requests
def download_and_parse_url(url):
# 使用Shell命令下载文件
subprocess.run(['wget', url, '-O', 'downloaded_page.html'], check=True)
# 使用Python解析数据
with open('downloaded_page.html', 'r') as file:
content = file.read()
# 简单解析
print('Page title:', content.split('<title>')[1].split('</title>')[0])
download_and_parse_url('http://example.com')
总之,Python和Shell结合使用可以大大提高开发效率,尤其是在自动化、数据处理和系统管理任务中。通过掌握这两种技术的结合使用,你可以更好地处理复杂的任务,优化工作流程。
相关问答FAQs:
如何将Python脚本与Shell命令结合使用?
在Python中,可以使用内置的subprocess
模块来执行Shell命令。通过subprocess.run()
或subprocess.Popen()
等函数,可以运行Shell命令并捕获输出。例如:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这段代码将执行ls -l
命令,并打印出结果。使用subprocess
模块可以让你在Python中灵活地使用Shell命令。
在Shell中如何调用Python脚本?
要在Shell中调用Python脚本,您需要确保脚本具有执行权限,并在命令行中使用python
命令或直接调用脚本名。例如:
chmod +x myscript.py
./myscript.py
或者使用:
python myscript.py
确保在脚本的第一行包含#!/usr/bin/env python3
,这样可以确保脚本被正确解释。
如何在Python中处理Shell命令的输出?
使用subprocess
模块可以方便地处理Shell命令的输出。通过设置capture_output=True
,您可以捕获标准输出和标准错误输出。例如:
import subprocess
try:
result = subprocess.run(['ls', '-z'], capture_output=True, text=True, check=True)
except subprocess.CalledProcessError as e:
print(f"Error: {e.stderr}")
else:
print(result.stdout)
在这个例子中,如果命令执行失败,Python会捕获错误并通过stderr
输出。这样可以有效地处理Shell命令的执行结果,确保代码的健壮性。
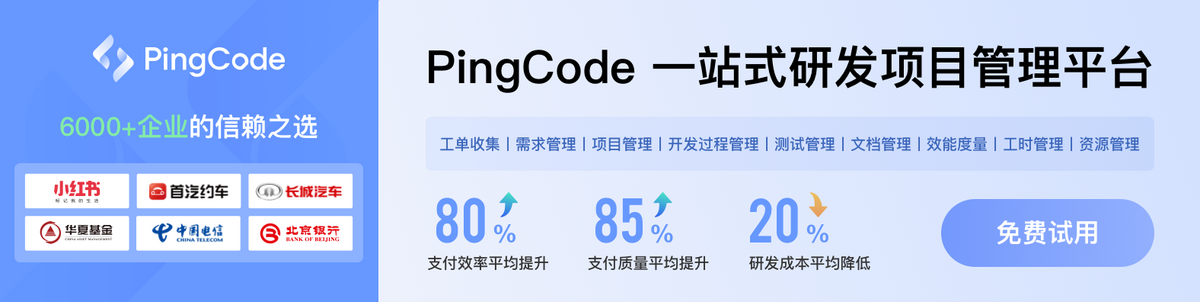