使用Python清理文本的几个关键步骤包括:去除标点符号、消除多余空格、转换大小写、去除停用词、词干提取和正则表达式清理。 在这些步骤中,去除标点符号是非常重要的,因为标点符号通常在文本分析中没有太大意义,它们可能会干扰分析结果。通过使用Python的string
库中的punctuation
方法,我们可以轻松去除文本中的标点符号。这一过程可以帮助提高文本数据的质量,使其更适合后续的文本分析和自然语言处理任务。
一、去除标点符号
去除标点符号是文本清理的第一步,因为标点符号通常不包含有用的信息。Python的string
库提供了一个punctuation
字符串,可以用于识别和去除文本中的标点符号。我们可以使用Python的str.translate()
和str.maketrans()
方法来实现这一功能。以下是一个简单的示例:
import string
def remove_punctuation(text):
translator = str.maketrans('', '', string.punctuation)
return text.translate(translator)
sample_text = "Hello, world! This is an example sentence."
cleaned_text = remove_punctuation(sample_text)
print(cleaned_text)
这段代码将输出“Hello world This is an example sentence”,去除了所有标点符号。
二、消除多余空格
多余空格可能在文本处理中导致问题,特别是在词汇匹配和模式识别时。Python的str.split()
和str.join()
方法可以轻松去除多余的空格,并确保单词之间只有一个空格:
def remove_extra_spaces(text):
return ' '.join(text.split())
sample_text = "This is an example sentence with extra spaces."
cleaned_text = remove_extra_spaces(sample_text)
print(cleaned_text)
这段代码将输出“This is an example sentence with extra spaces”,去除了多余的空格。
三、转换大小写
将文本转换为统一的大小写可以简化文本处理过程,尤其是在进行词汇比较时。通常选择将文本转换为小写,因为这是最常见的格式:
def to_lowercase(text):
return text.lower()
sample_text = "This Is A Mixed CASE Sentence."
cleaned_text = to_lowercase(sample_text)
print(cleaned_text)
这段代码将输出“this is a mixed case sentence”,将所有字符转换为小写。
四、去除停用词
停用词是在文本处理中需要忽略的常见词汇,如“is”、“and”、“the”等。Python的nltk
库提供了一个停用词列表,可以用于清理文本。首先需要安装并导入nltk
库:
pip install nltk
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
def remove_stopwords(text):
stop_words = set(stopwords.words('english'))
word_tokens = text.split()
filtered_sentence = [word for word in word_tokens if word.lower() not in stop_words]
return ' '.join(filtered_sentence)
sample_text = "This is an example sentence demonstrating the removal of stop words."
cleaned_text = remove_stopwords(sample_text)
print(cleaned_text)
这段代码将输出“example sentence demonstrating removal stop words”,去除了所有的停用词。
五、词干提取
词干提取是将单词还原为其词根形式的过程,这在文本分析中可以减少词形的变化。Python的nltk
库提供了一个PorterStemmer
类来实现词干提取:
from nltk.stem import PorterStemmer
def stem_words(text):
ps = PorterStemmer()
word_tokens = text.split()
stemmed_sentence = [ps.stem(word) for word in word_tokens]
return ' '.join(stemmed_sentence)
sample_text = "running runner ran runs"
cleaned_text = stem_words(sample_text)
print(cleaned_text)
这段代码将输出“run runner ran run”,通过词干提取减少了词形变化。
六、正则表达式清理
正则表达式是一种强大的文本处理工具,可以用于识别和清除特定的模式。Python的re
库提供了正则表达式功能。以下是一个示例,展示如何使用正则表达式去除文本中的数字:
import re
def remove_numbers(text):
return re.sub(r'\d+', '', text)
sample_text = "This sentence contains numbers like 123 and 456."
cleaned_text = remove_numbers(sample_text)
print(cleaned_text)
这段代码将输出“This sentence contains numbers like and .”,去除了所有的数字。
通过以上步骤,您可以使用Python清理文本数据,提高数据质量,为后续的分析或自然语言处理任务做好准备。这些方法在实际应用中可以根据具体需求进行调整和组合,以满足不同的文本清理要求。
相关问答FAQs:
如何用Python清理文本中的特殊字符?
清理文本中的特殊字符可以通过使用Python的正则表达式模块re
实现。可以通过re.sub()
函数替换掉不需要的字符。例如,re.sub(r'[^a-zA-Z0-9\s]', '', text)
可以移除所有非字母数字和空格的字符。这样,文本将更加干净,适合后续分析。
在文本清理中,如何处理多余的空格?
处理多余空格的方法是使用str.split()
和str.join()
组合。text.split()
会将文本分割成单词,并自动去除多余的空格,接着可以用' '.join()
将它们重新连接起来。例如,' '.join(text.split())
将返回一个没有多余空格的干净文本。
如何在Python中进行文本小写化处理?
将文本转换为小写可以使用str.lower()
方法,这样有助于统一文本格式,避免在处理文本时出现大小写不一致的问题。例如,cleaned_text = text.lower()
将把原始文本中的所有字母转换为小写形式,从而提高后续文本分析的准确性。
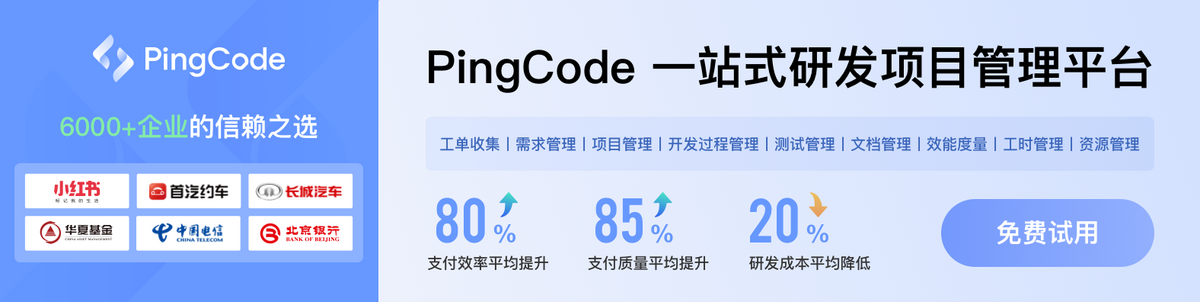