使用Python计算积分的方法包括:使用数值积分库如SciPy、使用符号计算库SymPy、以及自定义数值积分算法。我们将详细介绍使用SciPy库进行数值积分的步骤。
使用SciPy库进行数值积分是计算积分的常用方法之一。SciPy库是一个强大的科学计算库,提供了丰富的数学函数,其中包括积分计算。使用SciPy库的积分函数,我们可以方便地计算出定积分和不定积分。SciPy的quad
函数是用于计算一维定积分的函数,它使用自适应的高斯求积法,能够在大多数情况下提供准确的结果。我们可以通过传入被积函数、积分区间和其他参数来使用quad
函数,从而实现积分计算。在实际应用中,SciPy库的数值积分功能广泛应用于工程和科学计算中,帮助我们解决复杂的积分问题。
一、NUMERICAL INTEGRATION USING SCIPY
SciPy is a powerful scientific computing library in Python that provides a wide range of mathematical functions, including integration. The scipy.integrate
module offers several functions for numerical integration, with the quad
function being the most commonly used for calculating definite integrals.
1. Introduction to SciPy's Quad Function
SciPy's quad
function is designed to compute the definite integral of a function. It utilizes an adaptive quadrature method, specifically the quadrature method developed by Piessens and de Doncker. This method is highly efficient for a wide range of integrals, providing a balance between speed and accuracy.
To use quad
, you need to define the integrand function and specify the limits of integration. The function signature is as follows:
from scipy.integrate import quad
result, error = quad(func, a, b, args=())
func
: The integrand function.a
,b
: The limits of integration.args
: Additional arguments to pass tofunc
.
2. Example: Computing a Simple Integral
Consider computing the integral of ( f(x) = x^2 ) over the interval [0, 1]. This can be done using quad
as follows:
import numpy as np
from scipy.integrate import quad
Define the integrand function
def integrand(x):
return x2
Compute the integral
result, error = quad(integrand, 0, 1)
Output the result
print(f"The integral of x^2 from 0 to 1 is approximately {result:.5f}")
The output should be close to the analytical result of 1/3, demonstrating the accuracy of the quad
function.
3. Handling Infinite Limits
SciPy's quad
function can also handle integrals with infinite limits. To specify infinity, you can use numpy.inf
or float('inf')
. For example, calculating the integral of ( f(x) = e^{-x} ) from 0 to infinity:
from scipy.integrate import quad
import numpy as np
Define the integrand function
def integrand(x):
return np.exp(-x)
Compute the integral with an infinite upper limit
result, error = quad(integrand, 0, np.inf)
Output the result
print(f"The integral of exp(-x) from 0 to infinity is approximately {result:.5f}")
This integral evaluates to 1, which matches the expected analytical result.
二、SYMBOLIC INTEGRATION USING SYMPY
SymPy is a Python library for symbolic mathematics. It provides tools for algebraic manipulation, calculus, and more. For integration, SymPy can perform both definite and indefinite integrals symbolically, providing exact results when possible.
1. Introduction to SymPy Integration
SymPy's integrate
function can perform both definite and indefinite integrals. The function signature is:
from sympy import integrate, symbols
result = integrate(expr, var)
expr
: The expression to integrate.var
: The variable of integration.
For definite integrals, you can specify the limits:
result = integrate(expr, (var, a, b))
2. Example: Indefinite Integral
To compute the indefinite integral of ( f(x) = x^2 ), use:
from sympy import symbols, integrate
Define the symbol
x = symbols('x')
Define the expression
expr = x2
Compute the indefinite integral
indefinite_integral = integrate(expr, x)
Output the result
print(f"The indefinite integral of x^2 is {indefinite_integral}")
The result is ( \frac{x^3}{3} ), which is the expected antiderivative.
3. Example: Definite Integral
For a definite integral, say ( f(x) = x^2 ) from 0 to 1:
from sympy import symbols, integrate
Define the symbol
x = symbols('x')
Define the expression
expr = x2
Compute the definite integral
definite_integral = integrate(expr, (x, 0, 1))
Output the result
print(f"The definite integral of x^2 from 0 to 1 is {definite_integral}")
This yields 1/3, matching the numerical result from SciPy.
三、CUSTOM NUMERICAL INTEGRATION ALGORITHMS
While libraries like SciPy and SymPy provide ready-to-use functions for integration, understanding the underlying algorithms can be beneficial. Below, we explore some basic numerical integration methods.
1. Trapezoidal Rule
The trapezoidal rule is a simple method for approximating the integral of a function. It works by dividing the area under the curve into trapezoids and summing their areas.
The formula for the trapezoidal rule is:
[ \int_a^b f(x) , dx \approx \frac{b-a}{n} \left[ \frac{f(a) + f(b)}{2} + \sum_{i=1}^{n-1} f(x_i) \right] ]
Here's how you can implement it in Python:
import numpy as np
def trapezoidal_rule(func, a, b, n):
h = (b - a) / n
integral = (func(a) + func(b)) / 2.0
x = np.linspace(a, b, n+1)
integral += np.sum(func(x[1:-1]))
integral *= h
return integral
Example usage
def f(x):
return x2
result = trapezoidal_rule(f, 0, 1, 1000)
print(f"Trapezoidal rule result: {result:.5f}")
2. Simpson's Rule
Simpson's rule is another method for numerical integration. It provides better accuracy than the trapezoidal rule by fitting parabolas to the sections of the curve.
The formula for Simpson's rule is:
[ \int_a^b f(x) , dx \approx \frac{b-a}{6} \left[ f(a) + 4f\left(\frac{a+b}{2}\right) + f(b) \right] ]
Here's a Python implementation:
def simpsons_rule(func, a, b, n):
if n % 2 == 1:
n += 1 # n must be even
h = (b - a) / n
integral = func(a) + func(b)
for i in range(1, n, 2):
integral += 4 * func(a + i * h)
for i in range(2, n-1, 2):
integral += 2 * func(a + i * h)
integral *= h / 3
return integral
Example usage
result = simpsons_rule(f, 0, 1, 1000)
print(f"Simpson's rule result: {result:.5f}")
四、PRACTICAL APPLICATIONS OF INTEGRATION
Integration is a fundamental operation in many scientific and engineering disciplines. Below are some practical applications where integration plays a crucial role.
1. Physics and Engineering
In physics and engineering, integration is used to calculate quantities such as area, volume, mass, and moment of inertia. For example, the work done by a force along a path can be calculated as the integral of the force over the distance.
2. Probability and Statistics
In probability theory, integrals are used to compute probabilities and expected values. The integral of a probability density function over a given interval gives the probability that a random variable falls within that interval.
3. Economics and Finance
In economics and finance, integrals are used to model and predict market trends, calculate consumer and producer surplus, and evaluate risk and return on investments.
4. Biology and Medicine
In biology and medicine, integration is used to model biological processes, analyze medical data, and simulate the spread of diseases.
五、ADVANCED INTEGRATION TECHNIQUES
While basic numerical and symbolic integration methods cover many use cases, advanced techniques are sometimes necessary for complex integrals or when high precision is required.
1. Monte Carlo Integration
Monte Carlo integration is a probabilistic method for approximating integrals, particularly useful for high-dimensional integrals. It relies on random sampling and is widely used in fields such as statistical physics and quantitative finance.
2. Gaussian Quadrature
Gaussian quadrature is a technique for approximating the integral of a function, particularly effective when the function can be approximated by a polynomial. It involves choosing specific points and weights to maximize accuracy.
3. Adaptive Quadrature
Adaptive quadrature methods, like those used in SciPy's quad
function, dynamically adjust the number of evaluation points based on the function's behavior. This approach improves accuracy for functions with varying smoothness.
六、CONCLUSION
Calculating integrals in Python can be achieved through various methods, ranging from numerical approaches using SciPy to symbolic methods with SymPy. Understanding these techniques allows you to tackle a wide range of integration problems, whether they arise in academic research, industry applications, or personal projects. By mastering these tools, you can efficiently perform integration tasks and apply them to solve real-world challenges.
相关问答FAQs:
如何在Python中选择合适的库来计算积分?
Python提供了多个库来进行积分计算,其中最常用的是SciPy和SymPy。SciPy专注于数值计算,适合处理复杂的数值积分问题,而SymPy则是一个符号计算库,可以帮助用户得到精确的积分解。选择哪个库取决于具体需求:需要数值解还是符号解。
在Python中如何处理不定积分与定积分的区别?
不定积分通常用于寻找原函数,而定积分则用于计算函数在某一范围内的面积。在Python中,使用SymPy可以很容易地计算不定积分,示例代码如下:integrate(f, x)
。对于定积分,使用integrate(f, (x, a, b))
可以计算函数f在区间[a, b]上的积分。
如何解决在使用Python进行积分时可能遇到的精度问题?
在进行数值积分时,精度可能受到多种因素影响,如积分区间的选择、函数的性质等。为了提高精度,可以考虑使用更高阶的积分方法,例如使用SciPy中的quad
函数,它提供了自动适应的积分算法。此外,调整积分的精度参数也能有效改善结果,如通过设定epsabs
和epsrel
来控制绝对和相对误差。
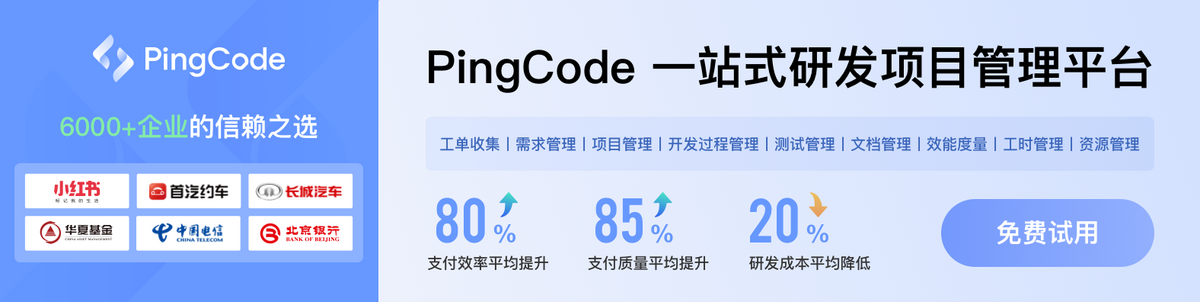