在Python中,self
是类的方法中的一个常用参数,用于指代类的实例对象,允许访问该类的属性和方法、使得类的方法能够操作实例的状态、在类定义中是必要的。self
本质上是一个约定俗成的名称,可以替换为其他任何有效的变量名,但为了代码的可读性和一致性,通常使用self
。在类的方法中,self
通常作为第一个参数传递,这是因为Python自动将调用方法的实例对象作为第一个参数传递给方法。一个详细的例子是,当你定义一个方法来修改类实例的属性时,你可以通过self
来访问和修改这些属性。
self
在类中的应用
self
在Python类中扮演了一个至关重要的角色。它不仅用于访问实例变量和方法,还用于区分实例变量和局部变量。当你在类的方法中定义变量时,使用self
来指定这是一个实例变量而不是方法的局部变量。通过这种方式,可以确保每个实例都有自己独立的属性集,并且可以通过方法进行修改。
例如,假设你有一个名为Car
的类,你希望每个Car
对象都有自己的颜色和型号。你可以在类中定义这些属性,并使用self
来访问和修改它们:
class Car:
def __init__(self, color, model):
self.color = color
self.model = model
def describe(self):
return f"This car is a {self.color} {self.model}."
def paint(self, new_color):
self.color = new_color
在这个示例中,self.color
和self.model
是实例变量。通过构造方法__init__
,每次创建一个新的Car
实例时,都会为这些变量赋值。describe
方法使用self
来访问这些实例变量并返回一条描述信息。而paint
方法则使用self
来修改color
属性。
一、self
在类的构造函数中
构造函数__init__
是类在创建实例时自动调用的方法。self
在构造函数中用于初始化实例的属性。通过在构造函数中定义self
,你可以确保每个实例都有自己的属性集。
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def get_info(self):
return f"'{self.title}' by {self.author}."
在这个例子中,Book
类的构造函数接受两个参数title
和author
,并将它们赋值给实例变量self.title
和self.author
。通过这种方式,每本书都有自己独特的标题和作者。
二、self
在实例方法中
self
在实例方法中的作用是用来访问和操作实例的属性和其他方法。通过使用self
,你可以在方法中读取或修改实例的属性。
class BankAccount:
def __init__(self, owner, balance=0):
self.owner = owner
self.balance = balance
def deposit(self, amount):
self.balance += amount
return f"{amount} deposited. New balance: {self.balance}"
def withdraw(self, amount):
if amount > self.balance:
return "Insufficient funds."
else:
self.balance -= amount
return f"{amount} withdrawn. New balance: {self.balance}"
在这个BankAccount
类中,self
用于访问和修改balance
属性。在deposit
和withdraw
方法中,通过self
操作账户余额,确保每个账户实例都能独立地管理自己的资金。
三、self
与类变量的区别
在Python中,类变量是所有类的实例共享的变量,而实例变量(通过self
定义)是特定于每个实例的变量。理解这一点对于正确使用self
至关重要。
class School:
total_students = 0 # 类变量
def __init__(self, name):
self.name = name # 实例变量
School.total_students += 1
def get_name(self):
return self.name
@classmethod
def get_total_students(cls):
return cls.total_students
在这个School
类中,total_students
是一个类变量,用于跟踪所有School
实例的总数量。self.name
是一个实例变量,特定于每个School
实例。类方法get_total_students
使用cls
访问类变量,而实例方法get_name
使用self
访问实例变量。
四、self
在继承中的使用
在面向对象编程中,继承允许一个类继承另一个类的属性和方法。在继承中,self
的使用与在普通类中相同,但需要注意的是,子类可以使用super()
来调用父类的方法。
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclasses must implement this method")
class Dog(Animal):
def speak(self):
return f"{self.name} says woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says meow!"
在这个例子中,Animal
类定义了一个抽象方法speak
,其子类Dog
和Cat
必须实现这个方法。self
用于访问每个动物实例的name
属性。在这种情况下,self
确保了每个动物实例都可以独立地实现其特定的行为。
五、self
与Python的特殊方法
Python中的特殊方法(也称为魔法方法)允许类与内置操作进行交互,例如算术运算、比较、字符串表示等。self
在这些方法中同样起到关键作用。
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Vector({self.x}, {self.y})"
在Vector
类中,__add__
方法允许两个向量对象相加,__str__
方法定义了向量对象的字符串表示。在这两个方法中,self
用于访问实例的x
和y
属性,使得每个向量对象都能正确地进行运算和表示。
六、self
在多态中的使用
多态是面向对象编程中的一个重要概念,指的是不同类的对象可以通过相同的接口调用不同的方法。在Python中,self
帮助实现多态行为。
class Shape:
def area(self):
raise NotImplementedError("Subclasses must implement this method")
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
在这个例子中,Shape
类定义了一个抽象的area
方法,子类Circle
和Rectangle
分别实现了该方法。self
用于访问每个形状实例的特定属性(如radius
、width
和height
),从而计算其面积。
七、self
与装饰器的结合使用
Python中的装饰器是一种高级功能,允许在不修改原函数的情况下扩展函数的行为。在类方法中使用装饰器时,self
可以帮助我们在装饰器中处理实例的状态。
def logged(func):
def wrapper(self, *args, kwargs):
print(f"Calling {func.__name__} with {args} and {kwargs}")
return func(self, *args, kwargs)
return wrapper
class Calculator:
@logged
def add(self, a, b):
return a + b
@logged
def subtract(self, a, b):
return a - b
在这个Calculator
类中,logged
装饰器用于记录方法调用的信息。self
作为方法的第一个参数传递给装饰器,使得装饰器能够正确调用和处理实例方法。
八、self
在类的迭代器中的应用
迭代器是Python中用于遍历容器对象的一个重要概念。在类中实现迭代器时,self
用于保存迭代器的状态。
class Countdown:
def __init__(self, start):
self.current = start
def __iter__(self):
return self
def __next__(self):
if self.current <= 0:
raise StopIteration
else:
self.current -= 1
return self.current + 1
在这个Countdown
类中,__iter__
和__next__
方法使得对象可以作为迭代器使用。self.current
用于跟踪计数器的当前状态,并在每次迭代时更新。
九、self
在单例模式中的使用
单例模式是一种设计模式,确保一个类只有一个实例,并提供全局访问点。在实现单例模式时,self
可以用于控制实例的创建。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def __init__(self, value):
self.value = value
在这个Singleton
类中,__new__
方法用于控制实例的创建,确保只有一个实例存在。self.value
用于存储实例的状态,并在创建后可以访问和修改。
十、self
在数据封装中的应用
数据封装是面向对象编程的一个基本原则,它通过将数据和操作封装在类中来保护数据不被外部直接访问。在实现数据封装时,self
用于定义和访问私有属性。
class Account:
def __init__(self, owner, balance):
self._owner = owner
self._balance = balance
def deposit(self, amount):
if amount > 0:
self._balance += amount
return f"{amount} deposited. New balance: {self._balance}"
else:
return "Invalid deposit amount."
def withdraw(self, amount):
if 0 < amount <= self._balance:
self._balance -= amount
return f"{amount} withdrawn. New balance: {self._balance}"
else:
return "Invalid withdrawal amount."
def get_balance(self):
return self._balance
在这个Account
类中,_owner
和_balance
属性是私有的,通过self
定义和访问。这样,外部代码无法直接修改这些属性,必须通过类的方法进行操作,从而实现数据的封装和保护。
总结,self
在Python类中是一个核心概念,用于引用类实例本身。它允许类的方法访问和操作实例的属性,确保每个实例都有独立的状态,并在类的继承、多态、封装、迭代器、单例模式等高级特性中发挥关键作用。在编写Python类时,理解和正确使用self
是实现面向对象编程的基础。
相关问答FAQs:
在Python中,self的主要作用是什么?
self是Python类中一个指向实例自身的引用。它使得类的方法能够访问实例的属性和其他方法。在定义类的方法时,必须将self作为第一个参数,以便在调用该方法时,Python能够将实例自身传递给该方法。这使得类的每个实例都可以拥有独立的属性和行为。
使用self时需要注意哪些常见错误?
在使用self时,常见的错误包括忘记在类方法中将self作为第一个参数,或者在调用类方法时没有通过实例调用,而是直接调用方法名。这些错误会导致程序无法正确访问实例的属性或方法,因此在编写类时应时刻关注self的使用方式。
如何在Python类中使用self来管理属性的访问?
通过使用self,开发者可以在类的构造函数中定义实例属性,并在类的方法中进行访问和修改。可以使用self.属性名的方式来设置和获取属性的值。这种方式确保了属性的封装性,并且可以通过定义getter和setter方法来进一步控制属性的访问逻辑,提高代码的可维护性和可读性。
在多重继承中,self的行为有什么特别之处?
在多重继承的情况下,self仍然指向当前实例,但方法解析顺序(MRO)会影响方法的调用顺序。当调用方法时,Python会按照MRO的顺序查找相应的方法。这意味着在设计类时,理解self在多重继承中的行为非常重要,以避免潜在的混淆和错误。
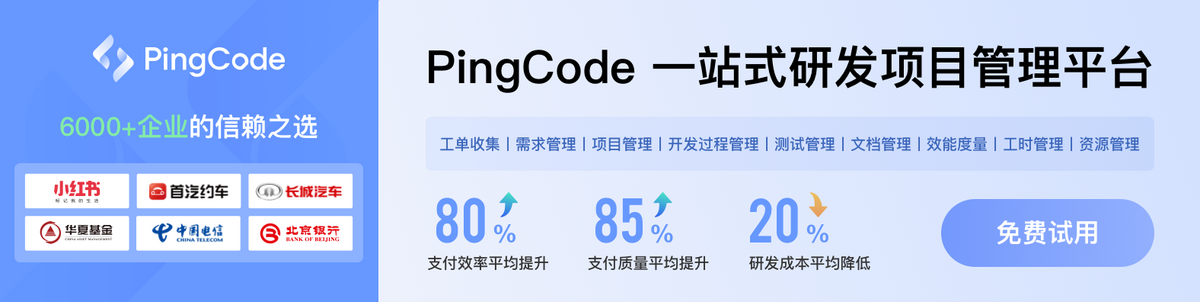