判断对象是否为空的方法有:使用if not
语句、利用len()
函数、使用==
或is
运算符、采用bool()
函数、结合try-except
语句。其中,最常用的方法是通过if not
语句来判断对象是否为空。具体展开如下:
在Python编程中,判断一个对象是否为空是一个常见的需求。空对象可以是空字符串、空列表、空字典、空集合等。常用的判断方法是使用if not
语句,因为它简洁且易读。例如:
my_list = []
if not my_list:
print("The list is empty")
这种方法利用了Python的真值测试(truth value testing),空对象在布尔上下文中会被评估为False
,因此if not
可以有效判断空对象。
一、使用if not
语句
if not
语句是判断对象是否为空的最常用方法,因为它直接利用了Python的真值测试机制。以下是一些常见的例子:
1.1、判断空字符串
my_string = ""
if not my_string:
print("The string is empty")
1.2、判断空列表
my_list = []
if not my_list:
print("The list is empty")
1.3、判断空字典
my_dict = {}
if not my_dict:
print("The dictionary is empty")
1.4、判断空集合
my_set = set()
if not my_set:
print("The set is empty")
二、利用len()
函数
len()
函数可以返回对象的长度或大小。对于可迭代对象(如列表、字符串、字典等),如果len()
返回0,则表示对象为空。
2.1、判断空字符串
my_string = ""
if len(my_string) == 0:
print("The string is empty")
2.2、判断空列表
my_list = []
if len(my_list) == 0:
print("The list is empty")
2.3、判断空字典
my_dict = {}
if len(my_dict) == 0:
print("The dictionary is empty")
2.4、判断空集合
my_set = set()
if len(my_set) == 0:
print("The set is empty")
三、使用==
或is
运算符
==
运算符可以判断对象是否等于特定的空值,如空字符串、空列表等。而is
运算符可以判断对象是否与特定的单例(如None
)相同。
3.1、判断空字符串
my_string = ""
if my_string == "":
print("The string is empty")
3.2、判断空列表
my_list = []
if my_list == []:
print("The list is empty")
3.3、判断空字典
my_dict = {}
if my_dict == {}:
print("The dictionary is empty")
3.4、判断空集合
my_set = set()
if my_set == set():
print("The set is empty")
四、采用bool()
函数
bool()
函数可以将对象转换为布尔值,空对象会被转换为False
,非空对象会被转换为True
。
4.1、判断空字符串
my_string = ""
if not bool(my_string):
print("The string is empty")
4.2、判断空列表
my_list = []
if not bool(my_list):
print("The list is empty")
4.3、判断空字典
my_dict = {}
if not bool(my_dict):
print("The dictionary is empty")
4.4、判断空集合
my_set = set()
if not bool(my_set):
print("The set is empty")
五、结合try-except
语句
在某些情况下,可能需要处理对象为空时抛出的异常。通过try-except
语句,可以捕获异常并处理空对象的情况。
5.1、判断空字符串
try:
my_string = ""
if my_string == "":
raise ValueError("The string is empty")
except ValueError as e:
print(e)
5.2、判断空列表
try:
my_list = []
if not my_list:
raise ValueError("The list is empty")
except ValueError as e:
print(e)
5.3、判断空字典
try:
my_dict = {}
if not my_dict:
raise ValueError("The dictionary is empty")
except ValueError as e:
print(e)
5.4、判断空集合
try:
my_set = set()
if not my_set:
raise ValueError("The set is empty")
except ValueError as e:
print(e)
六、结合自定义函数
在实际开发中,有时需要封装判断对象是否为空的逻辑。可以通过定义函数来实现这一功能,从而提高代码的重用性和可读性。
6.1、定义判断空对象的函数
def is_empty(obj):
if obj:
return False
return True
6.2、使用自定义函数判断空字符串
my_string = ""
if is_empty(my_string):
print("The string is empty")
6.3、使用自定义函数判断空列表
my_list = []
if is_empty(my_list):
print("The list is empty")
6.4、使用自定义函数判断空字典
my_dict = {}
if is_empty(my_dict):
print("The dictionary is empty")
6.5、使用自定义函数判断空集合
my_set = set()
if is_empty(my_set):
print("The set is empty")
七、判断自定义对象是否为空
在某些情况下,可能需要判断自定义类的实例是否为空。可以通过在类中定义__bool__
或__len__
方法来实现这一功能。
7.1、通过__bool__
方法判断
class MyClass:
def __init__(self, data):
self.data = data
def __bool__(self):
return bool(self.data)
my_instance = MyClass("")
if not my_instance:
print("The instance is empty")
7.2、通过__len__
方法判断
class MyClass:
def __init__(self, data):
self.data = data
def __len__(self):
return len(self.data)
my_instance = MyClass("")
if len(my_instance) == 0:
print("The instance is empty")
八、总结
判断对象是否为空是Python编程中的一个基本操作。本文介绍了多种判断方法,包括使用if not
语句、利用len()
函数、使用==
或is
运算符、采用bool()
函数、结合try-except
语句,以及通过自定义函数和自定义类方法。不同的方法适用于不同的场景,可以根据实际需求选择合适的判断方式。
在实际开发中,使用if not
语句是最为推荐的,因为它简洁且符合Python的惯用法。另外,结合自定义函数和类方法,可以提高代码的可读性和重用性,从而编写出更为优雅和高效的Python代码。
相关问答FAQs:
如何在Python中判断一个变量是否为空?
在Python中,判断一个变量是否为空通常可以通过使用if not
语句来实现。如果变量是空字符串、空列表、空字典或None
,条件将会为真。例如:
my_var = ""
if not my_var:
print("变量是空的")
这种方法适用于大多数数据类型,使得代码更加简洁和易读。
Python中有哪些常见的数据结构会被视为空?
在Python中,常见的被视为空的对象包括:空字符串""
、空列表[]
、空元组()
、空字典{}
、空集合set()
以及None
。这些对象在布尔上下文中都会返回False
,因此在进行条件判断时可以直接使用。
如何处理空值以避免程序错误?
处理空值时,可以使用try-except
语句来捕获可能的错误,或者在进行操作之前使用条件判断确认对象不为空。这种方式可以有效避免因操作空对象而导致的程序崩溃。例如:
my_list = None
if my_list is not None:
print(len(my_list))
else:
print("列表为空,无法获取长度")
通过这种方式,可以确保代码的健壮性,提升用户体验。
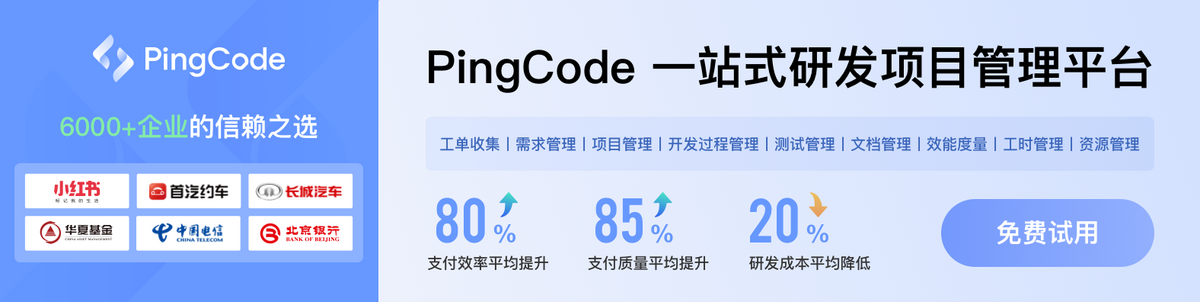