要在Python解释器中安装lxml库,你需要使用Python的包管理工具pip进行安装。安装lxml库的步骤包括:确保你有Python环境、使用命令行或终端、执行pip安装命令。 其中,最重要的一步是使用pip命令,因为pip是Python的包管理系统,能方便地从Python Package Index (PyPI) 下载并安装软件包。详细步骤如下:
-
确保你有Python环境:首先,确认你已经安装了Python并配置了环境变量。你可以在命令行中输入
python --version
或python3 --version
来查看Python版本。 -
使用命令行或终端:打开你的命令行工具(Windows下的命令提示符或PowerShell,macOS和Linux下的终端)。
-
执行pip安装命令:在命令行中输入
pip install lxml
或pip3 install lxml
(如果你的Python 3.x版本使用的是pip3)即可。这个命令会从PyPI下载并安装lxml库及其依赖。
接下来,我们将详细介绍每一步的具体操作和注意事项。
一、确保你有Python环境
在安装lxml之前,你需要先确保你的计算机已经安装了Python。如果你还没有安装Python,可以前往Python官方网站(https://www.python.org/)下载并安装适合你操作系统的Python版本。安装完成后,记得将Python添加到系统的环境变量中,以便在命令行中使用。
检查Python安装情况:
python --version
或
python3 --version
如果命令行返回了Python的版本号,说明Python已经正确安装。如果没有返回版本号或显示错误信息,则需要重新安装或检查环境变量配置。
二、使用命令行或终端
在Windows系统中,你可以使用“命令提示符”或“PowerShell”。在macOS和Linux系统中,使用“终端”即可。
三、执行pip安装命令
Windows系统:
- 打开命令提示符或PowerShell。
- 输入以下命令安装lxml库:
pip install lxml
如果你使用的是Python 3.x版本:
pip3 install lxml
macOS和Linux系统:
- 打开终端。
- 输入以下命令安装lxml库:
pip install lxml
如果你使用的是Python 3.x版本:
pip3 install lxml
四、验证lxml库安装
安装完成后,你可以通过在Python解释器中导入lxml库来验证安装是否成功。打开命令行或终端,并输入以下命令:
python
或
python3
进入Python解释器后,输入以下代码:
import lxml
print(lxml.__version__)
如果没有出现错误,并且成功输出了lxml的版本号,说明安装成功。
五、解决安装过程中可能遇到的问题
1. pip版本问题
有时,你的pip版本可能比较旧,无法安装某些库。在这种情况下,你需要先升级pip。可以使用以下命令升级pip:
pip install --upgrade pip
或
pip3 install --upgrade pip
2. 权限问题
在某些系统中,安装软件包可能需要管理员权限。如果遇到权限问题,可以尝试在命令前加上sudo
(适用于macOS和Linux):
sudo pip install lxml
或
sudo pip3 install lxml
3. 网络问题
如果由于网络问题无法安装,可以尝试使用国内的镜像源,比如阿里云的镜像源:
pip install lxml -i https://mirrors.aliyun.com/pypi/simple/
或
pip3 install lxml -i https://mirrors.aliyun.com/pypi/simple/
六、使用lxml库解析XML和HTML
安装完成后,你就可以在你的Python代码中使用lxml库来解析XML和HTML文档。下面是一些常见的使用示例:
解析XML文档
from lxml import etree
xml_string = '''
<root>
<child name="child1">Content 1</child>
<child name="child2">Content 2</child>
</root>
'''
root = etree.fromstring(xml_string)
for child in root:
print(child.tag, child.attrib, child.text)
解析HTML文档
from lxml import html
html_string = '''
<html>
<body>
<div id="content">
<p class="text">Hello, World!</p>
</div>
</body>
</html>
'''
tree = html.fromstring(html_string)
content = tree.get_element_by_id('content')
print(content.text_content())
七、lxml库的高级用法
lxml库不仅支持解析XML和HTML文档,还提供了许多高级功能,例如XPath、XSLT、Schema验证等。
使用XPath进行查询
from lxml import etree
xml_string = '''
<root>
<child name="child1">Content 1</child>
<child name="child2">Content 2</child>
</root>
'''
root = etree.fromstring(xml_string)
result = root.xpath('//child[@name="child1"]')
for r in result:
print(r.tag, r.attrib, r.text)
使用XSLT进行转换
from lxml import etree
xml_string = '''
<root>
<child name="child1">Content 1</child>
<child name="child2">Content 2</child>
</root>
'''
xslt_string = '''
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/root">
<html>
<body>
<h2>Child Elements</h2>
<ul>
<xsl:for-each select="child">
<li>
<xsl:value-of select="."/>
</li>
</xsl:for-each>
</ul>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
'''
xml_doc = etree.fromstring(xml_string)
xslt_doc = etree.fromstring(xslt_string)
transform = etree.XSLT(xslt_doc)
result = transform(xml_doc)
print(str(result))
Schema验证
from lxml import etree
xml_string = '''
<root>
<child name="child1">Content 1</child>
<child name="child2">Content 2</child>
</root>
'''
xsd_string = '''
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="root">
<xs:complexType>
<xs:sequence>
<xs:element name="child" maxOccurs="unbounded">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute name="name" type="xs:string" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
'''
xml_doc = etree.fromstring(xml_string)
xsd_doc = etree.fromstring(xsd_string)
schema = etree.XMLSchema(xsd_doc)
is_valid = schema.validate(xml_doc)
print("XML is valid:", is_valid)
八、总结
通过以上步骤,你可以轻松地在Python解释器中安装并使用lxml库。lxml库功能强大,性能优越,适用于各种XML和HTML文档的解析、查询和转换任务。希望通过这篇文章,你能够掌握lxml库的安装和基本使用方法,为你的Python开发提供有力支持。
相关问答FAQs:
如何确定我的Python版本与lxml库的兼容性?
在安装lxml库之前,确认你的Python版本非常重要。lxml库通常支持Python 2.7及以上版本,以及Python 3.x系列。如果你使用的是较旧的Python版本,可能会遇到兼容性问题。你可以通过在命令行中输入python --version
或python3 --version
来检查当前安装的Python版本。确保你的版本符合lxml的要求,以便顺利安装。
lxml库的安装需要依赖哪些其他库或工具?
在大多数情况下,lxml的安装需要依赖一些编译工具和库,例如libxml2和libxslt。如果你在Windows上安装,通常建议使用预编译的二进制包,这样可以避免手动安装这些依赖。如果你是在Linux或macOS上,确保已经安装了相应的开发工具和库,可以使用包管理工具(如apt或brew)进行安装。
如果在安装lxml库时遇到错误,我该如何解决?
在安装lxml库时,可能会遇到各种错误,比如编译失败或找不到依赖项。如果出现这种情况,建议查看错误信息,并根据提示进行相应的调整。常见的解决方案包括更新pip工具,使用pip install --upgrade pip
命令;确保你的开发环境配置正确;或者使用Anaconda等工具来简化环境管理和库安装。此外,查阅lxml的官方文档和社区支持也是解决问题的好方法。
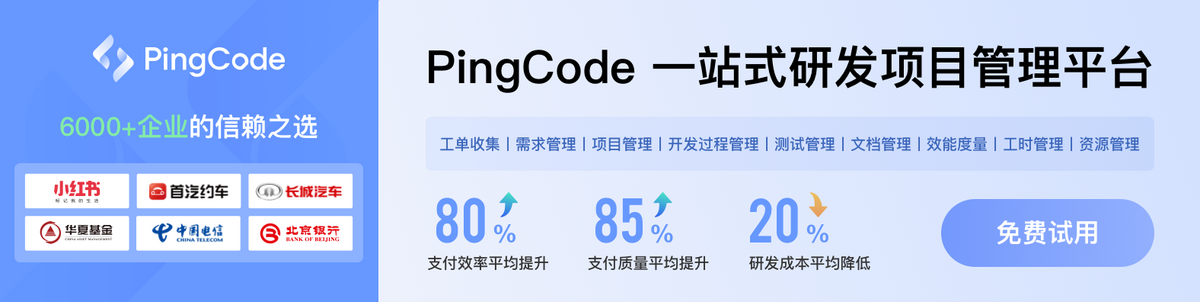