要去掉Python图表中的副坐标轴,可以使用Matplotlib库。通过设置secondary_y
参数为False
来去掉副坐标轴。 在绘制图表时,有时我们会在同一张图上绘制两个具有不同量纲的数据,这时可能会用到副坐标轴。但是,当我们不需要副坐标轴时,可以简单地禁用或删除它。
下面将详细介绍如何去掉副坐标轴,以及在使用Matplotlib进行高级图形绘制时的一些技巧和注意事项。
一、基本绘图与去掉副坐标轴
1、基本绘图
Matplotlib是Python中最常用的绘图库之一。我们首先展示如何用Matplotlib绘制一个简单的图表。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Plot')
plt.show()
2、绘制带有副坐标轴的图表
在一些情况下,我们可能需要添加副坐标轴,以便在同一图上显示不同量纲的数据。
fig, ax1 = plt.subplots()
color = 'tab:blue'
ax1.set_xlabel('X-axis')
ax1.set_ylabel('Primary Y-axis', color=color)
ax1.plot(x, y, color=color)
ax1.tick_params(axis='y', labelcolor=color)
ax2 = ax1.twinx() # instantiate a second axes that shares the same x-axis
color = 'tab:red'
ax2.set_ylabel('Secondary Y-axis', color=color)
ax2.plot(x, np.cos(x), color=color)
ax2.tick_params(axis='y', labelcolor=color)
fig.tight_layout()
plt.show()
3、去掉副坐标轴
当我们决定不需要副坐标轴时,可以简单地不创建它。这里是如何做到的:
fig, ax1 = plt.subplots()
color = 'tab:blue'
ax1.set_xlabel('X-axis')
ax1.set_ylabel('Primary Y-axis', color=color)
ax1.plot(x, y, color=color)
ax1.tick_params(axis='y', labelcolor=color)
Don't create ax2, no secondary y-axis
fig.tight_layout()
plt.show()
二、详细讲解去掉副坐标轴的方法
1、通过条件判断去掉副坐标轴
有时,我们可能需要根据某些条件去决定是否绘制副坐标轴。可以通过条件判断来实现。
should_display_secondary_axis = False
fig, ax1 = plt.subplots()
color = 'tab:blue'
ax1.set_xlabel('X-axis')
ax1.set_ylabel('Primary Y-axis', color=color)
ax1.plot(x, y, color=color)
ax1.tick_params(axis='y', labelcolor=color)
if should_display_secondary_axis:
ax2 = ax1.twinx()
color = 'tab:red'
ax2.set_ylabel('Secondary Y-axis', color=color)
ax2.plot(x, np.cos(x), color=color)
ax2.tick_params(axis='y', labelcolor=color)
fig.tight_layout()
plt.show()
2、动态添加或删除副坐标轴
在某些交互式图表中,可能需要动态地添加或删除副坐标轴。可以使用按钮或其他控件来实现这一点。
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
fig, ax1 = plt.subplots()
plt.subplots_adjust(bottom=0.2)
color = 'tab:blue'
ax1.set_xlabel('X-axis')
ax1.set_ylabel('Primary Y-axis', color=color)
ax1.plot(x, y, color=color)
ax1.tick_params(axis='y', labelcolor=color)
Initially, no secondary axis
ax2 = None
def toggle_secondary_axis(event):
global ax2
if ax2 is None:
ax2 = ax1.twinx()
color = 'tab:red'
ax2.set_ylabel('Secondary Y-axis', color=color)
ax2.plot(x, np.cos(x), color=color)
ax2.tick_params(axis='y', labelcolor=color)
else:
ax2.remove()
ax2 = None
fig.canvas.draw()
ax_button = plt.axes([0.81, 0.05, 0.1, 0.075])
btn = Button(ax_button, 'Toggle Axis')
btn.on_clicked(toggle_secondary_axis)
plt.show()
三、其他相关的高级绘图技巧
1、使用多个子图
有时,我们需要在同一个图表中显示多个子图,以便更好地比较不同的数据集。
fig, axs = plt.subplots(2)
axs[0].plot(x, y)
axs[0].set_title('Sine Wave')
axs[1].plot(x, np.cos(x), 'tab:orange')
axs[1].set_title('Cosine Wave')
for ax in axs.flat:
ax.set(xlabel='X-axis', ylabel='Y-axis')
plt.tight_layout()
plt.show()
2、调整子图之间的间距
使用plt.tight_layout()
可以自动调整子图之间的间距,以避免标签重叠。但是,有时我们需要手动调整这些间距。
fig, axs = plt.subplots(2, 2, figsize=(10, 10))
fig.subplots_adjust(hspace=0.4, wspace=0.4)
axs[0, 0].plot(x, y)
axs[0, 0].set_title('Plot 1')
axs[0, 1].plot(x, np.cos(x), 'tab:orange')
axs[0, 1].set_title('Plot 2')
axs[1, 0].plot(x, np.tan(x), 'tab:green')
axs[1, 0].set_title('Plot 3')
axs[1, 1].plot(x, -y, 'tab:red')
axs[1, 1].set_title('Plot 4')
for ax in axs.flat:
ax.set(xlabel='X-axis', ylabel='Y-axis')
plt.show()
3、高级自定义绘图
Matplotlib提供了丰富的自定义选项,可以满足各种复杂的绘图需求。例如,设置网格、添加注释、改变线型和颜色等。
fig, ax = plt.subplots()
ax.plot(x, y, label='Sine Wave', linestyle='--', color='blue', linewidth=2)
ax.plot(x, np.cos(x), label='Cosine Wave', linestyle='-', color='red', linewidth=2)
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Advanced Custom Plot')
ax.grid(True)
ax.legend()
Add annotations
ax.annotate('Local max', xy=(np.pi/2, 1), xytext=(np.pi/2 + 1, 1.5),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
四、总结
通过上述内容,我们详细介绍了如何在Python中使用Matplotlib去掉副坐标轴,以及一些相关的高级绘图技巧。主要的方法包括:不创建副坐标轴、通过条件判断、动态添加或删除副坐标轴。此外,还介绍了使用多个子图、调整子图间距、以及高级自定义绘图的方法。掌握这些技巧,能够帮助我们在数据可视化过程中更加灵活和高效地展示数据。
相关问答FAQs:
如何在Python绘图中完全移除副坐标轴?
要完全移除副坐标轴,您可以使用Matplotlib库中的spines
属性。通过设置副坐标轴的可见性为False,您可以隐藏它。例如,使用ax.spines['right'].set_visible(False)
可以有效去掉右侧的副坐标轴。
在Matplotlib中,如何控制副坐标轴的显示与隐藏?
通过使用Matplotlib的Axes
对象,可以轻松控制副坐标轴的显示。您可以设置副坐标轴的可见性,或者通过set_ylim
方法调整副坐标轴的范围,达到隐藏的效果。具体代码示例可以参考官方文档或在线教程。
使用Seaborn绘图时,如何处理副坐标轴的显示问题?
Seaborn库通常会基于Matplotlib进行绘图,因此可以使用与Matplotlib相同的方法处理副坐标轴。您可以在绘图后,通过调用Matplotlib的相关函数来隐藏或调整副坐标轴,从而实现您想要的效果。
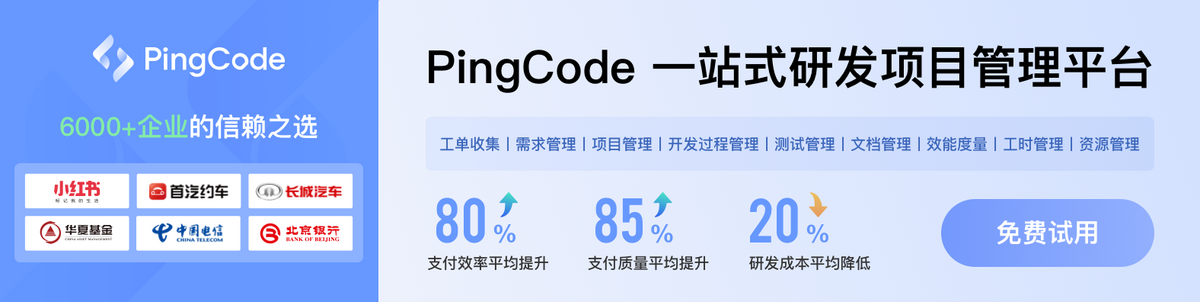