在Python中,可以通过文件扩展名、MIME类型、文件头信息等方法来区分文件类型。其中,使用文件扩展名是最常见和简单的方法,而使用MIME类型和文件头信息则更为准确。使用文件头信息判断文件类型是通过读取文件的前几个字节来确定其类型,这种方法比单纯依赖文件扩展名更为可靠。
为了详细描述其中的一种方法,我们来看一下如何通过MIME类型来区分文件类型。MIME(Multipurpose Internet Mail Extensions)类型是一种标准,用于表示文档、文件或字节流的性质和格式。Python中的mimetypes
模块可以帮助我们根据文件扩展名来推断MIME类型。以下是具体的方法和示例代码:
import mimetypes
def get_file_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
file_path = 'example.pdf'
mime_type = get_file_mime_type(file_path)
print(f'The MIME type of the file is: {mime_type}')
一、使用文件扩展名区分文件类型
文件扩展名是文件名的最后一部分,通常由点(.)分隔。通过检查文件的扩展名,我们可以大致判断出文件的类型。例如,扩展名为.txt
的文件通常是文本文件,扩展名为.jpg
的文件通常是JPEG图像文件。
def get_file_extension(file_path):
return file_path.split('.')[-1]
file_path = 'example.txt'
file_extension = get_file_extension(file_path)
print(f'The file extension is: {file_extension}')
尽管这种方法简单直观,但不够可靠,因为用户可以随意更改文件的扩展名,从而导致误判。
二、使用MIME类型区分文件类型
MIME类型提供了一种更为标准和准确的方法来区分文件类型。Python的mimetypes
模块可以根据文件扩展名推断出文件的MIME类型。
import mimetypes
def get_file_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
file_path = 'example.jpg'
mime_type = get_file_mime_type(file_path)
print(f'The MIME type of the file is: {mime_type}')
MIME类型不仅可以表示常见的文件类型,还可以表示一些特殊的文件类型,例如音视频文件、压缩文件等。
三、使用文件头信息区分文件类型
文件头信息是文件的前几个字节,通常包含了文件的标识符。通过读取文件头信息,我们可以更加准确地确定文件类型。Python的python-magic
库可以帮助我们实现这一功能。
首先,需要安装python-magic
库:
pip install python-magic
然后,使用该库来获取文件类型:
import magic
def get_file_type(file_path):
file_type = magic.from_file(file_path, mime=True)
return file_type
file_path = 'example.png'
file_type = get_file_type(file_path)
print(f'The file type is: {file_type}')
这种方法比单纯依赖文件扩展名更加可靠,因为它直接读取文件内容来判断类型,而不是依赖用户提供的信息。
四、结合多种方法提高准确性
为了提高文件类型判断的准确性,我们可以结合使用文件扩展名、MIME类型和文件头信息的方法。这种多层次的方法可以在不同情况下提供更加准确的结果。
import mimetypes
import magic
def get_file_extension(file_path):
return file_path.split('.')[-1]
def get_file_mime_type(file_path):
mime_type, encoding = mimetypes.guess_type(file_path)
return mime_type
def get_file_type(file_path):
file_type = magic.from_file(file_path, mime=True)
return file_type
file_path = 'example.docx'
file_extension = get_file_extension(file_path)
file_mime_type = get_file_mime_type(file_path)
file_type = get_file_type(file_path)
print(f'File extension: {file_extension}')
print(f'MIME type: {file_mime_type}')
print(f'File type: {file_type}')
通过上述方法,我们可以在不同层次上对文件类型进行判断,从而获得更加可靠的结果。
五、实际应用中的文件类型判断
在实际应用中,文件类型判断非常重要。例如,在文件上传系统中,我们需要确保用户上传的文件是允许的类型。在邮件附件扫描中,我们需要确保附件文件没有安全隐患。在数据处理系统中,我们需要根据文件类型选择合适的处理方式。
import os
def is_allowed_file_type(file_path, allowed_types):
file_type = get_file_type(file_path)
return file_type in allowed_types
allowed_types = ['image/jpeg', 'image/png', 'application/pdf']
uploaded_file_path = 'uploaded_file.png'
if is_allowed_file_type(uploaded_file_path, allowed_types):
print('File type is allowed.')
else:
print('File type is not allowed.')
通过上述代码,我们可以在文件上传过程中检查文件类型,确保只允许特定类型的文件上传。这种方法可以有效提高系统的安全性和可靠性。
六、文件类型判断的性能优化
在处理大量文件时,文件类型判断的性能非常重要。我们可以通过一些优化方法来提高判断的效率。例如,可以使用缓存机制来减少重复判断的开销。
import functools
@functools.lru_cache(maxsize=128)
def cached_get_file_type(file_path):
return get_file_type(file_path)
file_path = 'example.mp4'
file_type = cached_get_file_type(file_path)
print(f'File type (cached): {file_type}')
通过使用functools.lru_cache
装饰器,我们可以缓存最近使用的文件类型判断结果,从而减少重复判断的开销。这种方法在处理大量文件时可以显著提高性能。
七、文件类型判断的扩展性
在实际应用中,我们可能会遇到一些特殊的文件类型,这些文件类型可能不在标准MIME类型列表中。为了处理这些特殊情况,我们可以扩展文件类型判断的方法。
def custom_get_file_type(file_path):
custom_types = {
'special_format': 'application/x-special-format'
}
file_extension = get_file_extension(file_path)
if file_extension in custom_types:
return custom_types[file_extension]
return get_file_type(file_path)
file_path = 'example.special_format'
file_type = custom_get_file_type(file_path)
print(f'Custom file type: {file_type}')
通过定义自定义类型映射,我们可以处理一些特殊的文件类型,从而提高文件类型判断的扩展性和灵活性。
八、总结
在Python中区分文件类型的方法有多种,包括使用文件扩展名、MIME类型、文件头信息等。每种方法都有其优缺点,结合使用多种方法可以提高判断的准确性。在实际应用中,文件类型判断非常重要,可以帮助我们提高系统的安全性和可靠性。通过性能优化和扩展性设计,我们可以在处理大量文件和特殊文件类型时保持高效和灵活。
相关问答FAQs:
如何在Python中识别文件的类型?
在Python中,可以使用mimetypes
模块来识别文件的类型。这个模块提供了根据文件扩展名返回MIME类型的功能。你可以通过以下代码来实现:
import mimetypes
file_path = 'example.txt'
mime_type, _ = mimetypes.guess_type(file_path)
print(mime_type) # 输出:text/plain
通过这种方式,你可以快速识别出文件的类型,便于后续的处理。
使用Python如何根据文件内容判断文件类型?
除了根据文件扩展名判断外,Python还可以通过读取文件的内容来判断文件类型。使用python-magic
库可以实现这一功能。首先需要安装该库:
pip install python-magic
接着可以使用如下代码来检测文件类型:
import magic
file_path = 'example.pdf'
file_type = magic.from_file(file_path, mime=True)
print(file_type) # 输出:application/pdf
这种方法能够提供更为准确的文件类型判断,尤其是在文件扩展名不可靠的情况下。
在Python中是否有办法批量处理文件类型判断?
当然可以!你可以编写一个循环来遍历文件夹中的所有文件,并对每个文件进行类型判断。以下是一个示例代码:
import os
import mimetypes
directory_path = 'your_directory'
for filename in os.listdir(directory_path):
file_path = os.path.join(directory_path, filename)
mime_type, _ = mimetypes.guess_type(file_path)
print(f'File: {filename}, Type: {mime_type}')
这样的处理方式能有效地帮助你管理和识别多个文件的类型,提升工作效率。
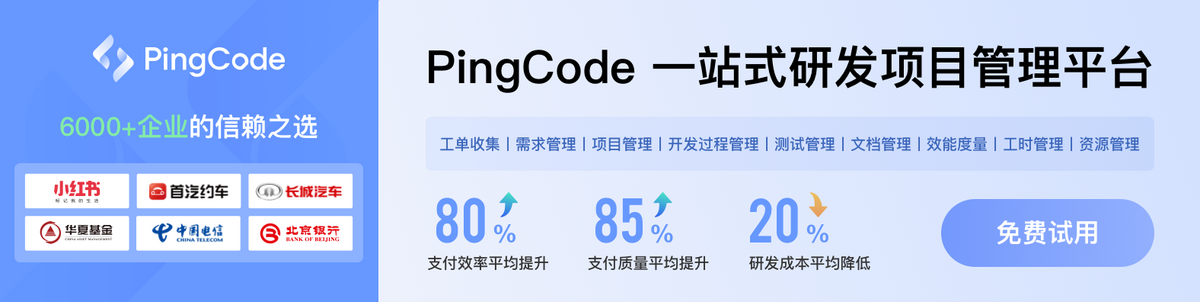