Python中减少最后一行换行的方法有:使用end参数、使用rstrip方法、使用write方法。最常用的方法是使用end参数,在print函数中通过设置end参数来控制输出的结尾字符。
在Python中,print函数默认会在每次输出后添加一个换行符。为了减少最后一行的换行符,可以通过设置end参数来控制输出的结尾字符。下面将详细介绍这几种方法。
一、使用end参数
1、设置end参数为空字符串
通过设置end参数为空字符串,可以控制print函数输出后不自动添加换行符:
print("Hello, world!", end="")
print(" This is on the same line.")
输出结果为:
Hello, world! This is on the same line.
2、结合条件控制最后一行换行
如果需要在循环输出中控制最后一行不换行,可以结合条件判断:
items = ["apple", "banana", "cherry"]
for i, item in enumerate(items):
if i == len(items) - 1:
print(item, end="")
else:
print(item)
输出结果为:
apple
banana
cherry
这样,最后一行不会额外添加换行符。
二、使用rstrip方法
1、去除字符串末尾的换行符
在某些情况下,字符串末尾可能包含换行符,可以使用rstrip方法去除:
text = "Hello, world!\n"
print(text.rstrip())
输出结果为:
Hello, world!
2、结合文件输出
在文件写入操作中,也可以使用rstrip方法去除末尾换行符:
with open("output.txt", "w") as file:
file.write("Hello, world!\n".rstrip())
这样写入文件时就不会在末尾添加额外的换行符。
三、使用write方法
1、直接写入字符串
使用sys.stdout.write方法直接写入字符串,不会自动添加换行符:
import sys
sys.stdout.write("Hello, world!")
sys.stdout.write(" This is on the same line.")
输出结果为:
Hello, world! This is on the same line.
2、结合文件写入
在文件写入操作中,可以直接使用write方法,不会自动添加换行符:
with open("output.txt", "w") as file:
file.write("Hello, world!")
file.write(" This is on the same line.")
输出结果为:
Hello, world! This is on the same line.
四、使用字符串拼接
1、手动拼接字符串
通过手动拼接字符串,可以控制输出的格式:
text = "Hello, world!" + " This is on the same line."
print(text)
输出结果为:
Hello, world! This is on the same line.
2、结合循环和条件
在循环输出中,结合条件判断手动拼接字符串:
items = ["apple", "banana", "cherry"]
output = ""
for i, item in enumerate(items):
if i == len(items) - 1:
output += item
else:
output += item + "\n"
print(output)
输出结果为:
apple
banana
cherry
通过以上几种方法,可以在Python中有效地控制和减少最后一行的换行符,确保输出格式符合预期需求。
相关问答FAQs:
如何在Python中避免打印时产生多余的换行符?
在Python中,使用print()
函数时,可以通过设置参数end
来控制输出的结尾字符。默认情况下,print()
会在每次输出后添加一个换行符(\n
)。如果希望避免在最后一行输出时产生换行,可以将end
参数设置为空字符串,例如:print("内容", end="")
。
如何在文件操作中减少换行符的影响?
在处理文件时,如果希望在写入文件的最后一行不添加换行符,可以在写入内容时,手动判断是否是最后一行。可以先读取文件内容,确定最后一行的内容,然后根据需要选择性地添加换行符。这种方法可以确保文件格式保持一致。
在字符串连接中如何避免多余的换行?
如果需要拼接多个字符串,并且希望在最后一个字符串后不添加换行符,可以使用字符串的join()
方法。通过将所有需要的字符串放入一个列表中,并使用join()
方法连接它们,可以自定义连接符。例如:result = "\n".join(list_of_strings)
,这样就能确保在最后一行没有多余的换行符。
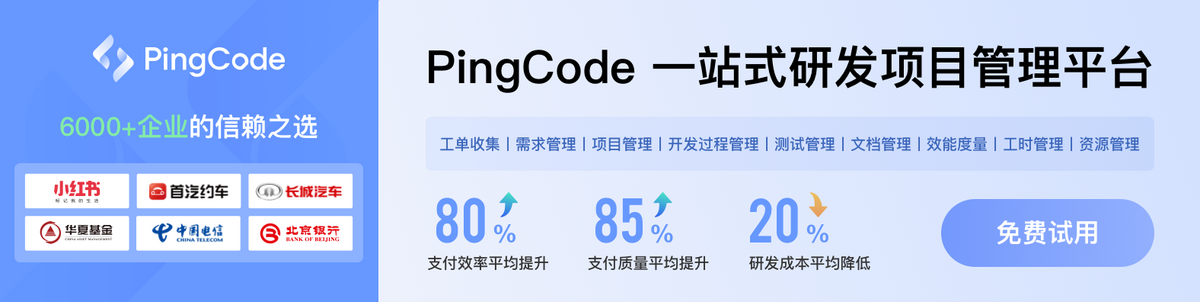