Python对比两个点云的方法包括:使用PCL库、使用Open3D库、计算点云的Hausdorff距离、使用ICP算法对齐点云。 其中,使用Open3D库是一个高效和便捷的方法。
详细描述:Open3D库 提供了丰富的点云处理工具,包括点云的加载、保存、可视化、滤波、配准等操作。使用Open3D库对比两个点云的常用方法是使用ICP(Iterative Closest Point)算法进行配准,通过计算配准后的误差来判断两点云的相似度。ICP算法通过迭代地调整点云的相对位置,使得两点云的重合程度最大,从而计算出它们的相似度。
Python对比两个点云的方法详解
一、使用PCL库
PCL(Point Cloud Library)是一个功能强大的点云处理库,提供了丰富的工具来处理点云数据。使用PCL可以实现点云的读取、写入、滤波、配准、特征提取等操作。
1. 安装PCL库
在Python中使用PCL需要安装pclpy
库,这是PCL的Python绑定。可以通过以下命令安装:
pip install pclpy
2. 读取和显示点云
使用PCL库可以轻松地读取和显示点云数据。以下是一个简单的示例:
import pclpy
from pclpy import pcl
读取点云
cloud = pcl.PointCloud.PointXYZ()
pcl.io.loadPLYFile("path_to_point_cloud.ply", cloud)
显示点云
viewer = pcl.visualization.PCLVisualizer("3D Viewer")
viewer.addPointCloud(cloud, "sample cloud")
viewer.spin()
3. 点云配准
PCL库提供了多种点云配准算法,包括ICP、NDT(Normal Distributions Transform)、RANSAC等。以下是使用ICP算法进行点云配准的示例:
# 创建ICP对象
icp = pcl.registration.IterativeClosestPoint.PointXYZ()
设置输入点云和目标点云
icp.setInputSource(cloud_source)
icp.setInputTarget(cloud_target)
执行配准
final_cloud = pcl.PointCloud.PointXYZ()
icp.align(final_cloud)
输出配准结果
print("Has converged:", icp.hasConverged())
print("Fitness score:", icp.getFitnessScore())
二、使用Open3D库
Open3D是一个开源的3D数据处理库,提供了点云、网格、几何体等3D数据的处理工具。Open3D库的特点是简单易用,功能强大,特别适合用于科研和工程应用。
1. 安装Open3D库
可以通过以下命令安装Open3D库:
pip install open3d
2. 读取和显示点云
使用Open3D库可以方便地读取和显示点云数据。以下是一个简单的示例:
import open3d as o3d
读取点云
cloud = o3d.io.read_point_cloud("path_to_point_cloud.ply")
显示点云
o3d.visualization.draw_geometries([cloud])
3. 点云配准
Open3D库提供了多种点云配准算法,包括ICP、Colored ICP、Global Registration等。以下是使用ICP算法进行点云配准的示例:
# 加载源点云和目标点云
source_cloud = o3d.io.read_point_cloud("path_to_source_cloud.ply")
target_cloud = o3d.io.read_point_cloud("path_to_target_cloud.ply")
下采样
source_down = source_cloud.voxel_down_sample(voxel_size=0.05)
target_down = target_cloud.voxel_down_sample(voxel_size=0.05)
计算法线
source_down.estimate_normals(search_param=o3d.geometry.KDTreeSearchParamHybrid(radius=0.1, max_nn=30))
target_down.estimate_normals(search_param=o3d.geometry.KDTreeSearchParamHybrid(radius=0.1, max_nn=30))
执行ICP配准
trans_init = np.eye(4)
reg_p2p = o3d.pipelines.registration.registration_icp(
source_down, target_down, 0.02, trans_init,
o3d.pipelines.registration.TransformationEstimationPointToPoint())
print("Transformation matrix:")
print(reg_p2p.transformation)
print("Fitness score:", reg_p2p.fitness)
应用变换并显示配准结果
source_cloud.transform(reg_p2p.transformation)
o3d.visualization.draw_geometries([source_cloud, target_cloud])
三、计算点云的Hausdorff距离
Hausdorff距离是一种常用的点云相似度度量方法,用于衡量两个点云之间的最大距离。可以通过以下步骤计算点云的Hausdorff距离:
1. 安装相关库
需要安装scipy
库来计算Hausdorff距离:
pip install scipy
2. 计算Hausdorff距离
以下是计算两个点云的Hausdorff距离的示例:
import numpy as np
from scipy.spatial import distance
加载点云数据
cloud1 = np.loadtxt("path_to_cloud1.txt")
cloud2 = np.loadtxt("path_to_cloud2.txt")
计算Hausdorff距离
dist = distance.directed_hausdorff(cloud1, cloud2)[0]
print("Hausdorff distance:", dist)
四、使用ICP算法对齐点云
ICP(Iterative Closest Point)算法是一种常用的点云配准算法,通过迭代地调整点云的相对位置,使得两点云的重合程度最大。以下是使用ICP算法对齐点云的详细步骤:
1. 安装相关库
需要安装numpy
和scipy
库:
pip install numpy scipy
2. 实现ICP算法
以下是一个简单的ICP算法实现示例:
import numpy as np
from scipy.spatial import KDTree
def icp(A, B, max_iterations=100, tolerance=1e-6):
# 初始化变换矩阵
T = np.eye(4)
prev_error = float('inf')
for i in range(max_iterations):
# 计算最近点对
tree = KDTree(B)
distances, indices = tree.query(A)
# 计算均值
A_mean = np.mean(A, axis=0)
B_mean = np.mean(B[indices], axis=0)
# 去均值
A_centered = A - A_mean
B_centered = B[indices] - B_mean
# 计算协方差矩阵
H = np.dot(A_centered.T, B_centered)
# 计算SVD
U, S, Vt = np.linalg.svd(H)
# 计算旋转矩阵
R = np.dot(Vt.T, U.T)
# 计算平移向量
t = B_mean - np.dot(R, A_mean)
# 更新变换矩阵
T[:3, :3] = R
T[:3, 3] = t
# 应用变换
A = np.dot(A, R.T) + t
# 计算误差
error = np.mean(distances)
if np.abs(prev_error - error) < tolerance:
break
prev_error = error
return T, error
加载点云数据
cloud1 = np.loadtxt("path_to_cloud1.txt")
cloud2 = np.loadtxt("path_to_cloud2.txt")
执行ICP配准
transformation, error = icp(cloud1, cloud2)
print("Transformation matrix:")
print(transformation)
print("ICP error:", error)
通过以上方法,可以在Python中使用PCL库、Open3D库、Hausdorff距离和ICP算法对比两个点云。这些方法各有优劣,可以根据具体需求选择合适的方法。
相关问答FAQs:
如何使用Python对比两个点云的相似性?
在Python中,可以使用点云处理库如Open3D或PCL(Point Cloud Library)来对比两个点云的相似性。通常可以通过计算点云之间的距离(如Hausdorff距离)或使用ICP(Iterative Closest Point)算法来评估它们的匹配程度。Open3D提供了丰富的函数来实现这些功能,包括点云的配准、距离计算和可视化。
对比点云时,有哪些常用的算法和技术?
常见的对比点云的算法包括ICP(Iterative Closest Point),用于对齐两个点云,和RANSAC(随机采样一致性算法),用于识别点云中的特征并排除异常值。此外,利用特征描述符(如FPFH或SHOT)可以提取点云的几何特征,以便更好地进行对比和匹配。
在Python中如何可视化对比结果?
使用Open3D等库,您可以轻松地可视化点云数据及其对比结果。通过绘制原始点云和经过对齐的点云,您可以直观地观察它们之间的差异。Open3D提供的可视化工具允许您在3D视图中旋转、缩放和移动,以便更好地分析点云的结构和相似性。
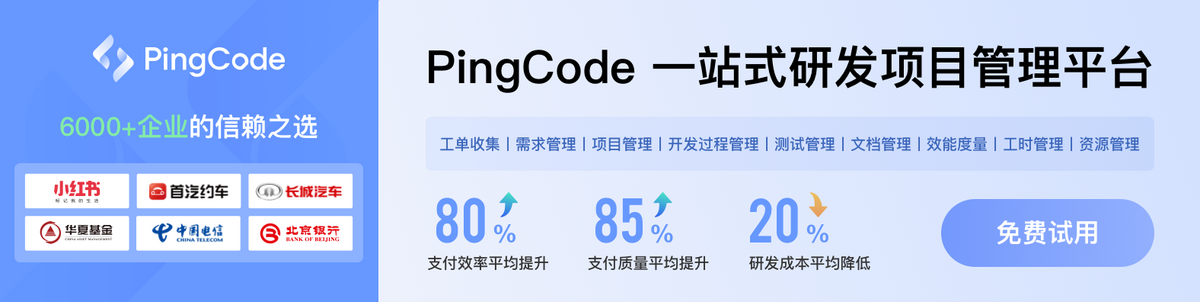