Python爬取亚马逊网页指定数据的方法包括使用请求库、解析HTML内容、处理反爬机制
在开始爬取亚马逊网页指定数据时,首先需要明确爬取的目标、使用的工具和处理反爬机制的方法。使用requests库发送HTTP请求、利用BeautifulSoup解析HTML内容、使用代理和模拟浏览器行为处理反爬是爬取亚马逊网页的关键步骤。接下来我们将详细介绍每个步骤。
一、发送HTTP请求
要爬取亚马逊网页数据,首先需要发送HTTP请求获取网页内容。Python的requests库是一个非常方便的HTTP库,可以轻松发送GET请求来获取页面内容。
import requests
url = 'https://www.amazon.com/dp/B08N5WRWNW' # 替换为目标产品URL
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
page_content = response.text
else:
print(f"Failed to retrieve page, status code: {response.status_code}")
二、解析HTML内容
获取到网页内容后,需要解析HTML以提取指定数据。BeautifulSoup是一个强大的HTML解析库,可以方便地提取HTML标签中的内容。
from bs4 import BeautifulSoup
soup = BeautifulSoup(page_content, 'html.parser')
示例:提取产品标题
title = soup.find(id='productTitle').get_text(strip=True)
print(f"Product Title: {title}")
示例:提取产品价格
price = soup.find('span', {'class': 'a-price-whole'}).get_text(strip=True)
print(f"Product Price: {price}")
三、处理反爬机制
亚马逊有严格的反爬机制,包括IP封禁、CAPTCHA验证等。为了避免被封禁,可以使用以下方法:
- 使用代理:通过代理服务器发送请求,避免IP被封禁。
proxies = {
'http': 'http://your_proxy:port',
'https': 'https://your_proxy:port'
}
response = requests.get(url, headers=headers, proxies=proxies)
- 模拟浏览器行为:使用Selenium等工具模拟浏览器行为,增加请求的真实性。
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
options = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
options.add_argument('--no-sandbox')
options.add_argument('--disable-dev-shm-usage')
options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36")
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
driver.get(url)
page_content = driver.page_source
driver.quit()
soup = BeautifulSoup(page_content, 'html.parser')
四、代码示例与总结
以下是一个完整的代码示例,展示如何爬取亚马逊产品的标题和价格,并处理反爬机制:
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
使用requests库发送HTTP请求
def fetch_page_content(url, headers, proxies=None):
response = requests.get(url, headers=headers, proxies=proxies)
if response.status_code == 200:
return response.text
else:
print(f"Failed to retrieve page, status code: {response.status_code}")
return None
使用BeautifulSoup解析HTML内容
def parse_product_details(page_content):
soup = BeautifulSoup(page_content, 'html.parser')
title = soup.find(id='productTitle').get_text(strip=True)
price = soup.find('span', {'class': 'a-price-whole'}).get_text(strip=True)
return title, price
使用Selenium模拟浏览器行为
def fetch_page_content_with_selenium(url):
options = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
options.add_argument('--no-sandbox')
options.add_argument('--disable-dev-shm-usage')
options.add_argument("user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36")
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
driver.get(url)
page_content = driver.page_source
driver.quit()
return page_content
def main():
url = 'https://www.amazon.com/dp/B08N5WRWNW' # 替换为目标产品URL
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
# 直接使用requests获取页面内容
page_content = fetch_page_content(url, headers)
if page_content:
title, price = parse_product_details(page_content)
print(f"Product Title: {title}")
print(f"Product Price: {price}")
# 使用Selenium获取页面内容
page_content_selenium = fetch_page_content_with_selenium(url)
if page_content_selenium:
title, price = parse_product_details(page_content_selenium)
print(f"Product Title (Selenium): {title}")
print(f"Product Price (Selenium): {price}")
if __name__ == "__main__":
main()
通过上述代码示例,我们展示了如何使用Python爬取亚马逊网页的指定数据,包括产品标题和价格,并处理亚马逊的反爬机制。使用requests库发送HTTP请求、利用BeautifulSoup解析HTML内容、使用代理和模拟浏览器行为处理反爬是爬取亚马逊网页数据的关键步骤。希望通过本文的详细介绍,能够帮助读者更好地理解和实现亚马逊网页数据的爬取。
相关问答FAQs:
如何使用Python爬取亚马逊网页的商品信息?
要爬取亚马逊网页的商品信息,可以使用Python中的库如BeautifulSoup和requests。首先,需要发送HTTP请求获取网页内容,然后解析HTML结构,提取所需数据,例如商品名称、价格和评价等。确保遵循亚马逊的使用条款,避免过于频繁的请求,以免被封禁。
爬取亚马逊数据时需要注意哪些法律和道德问题?
在爬取亚马逊数据时,遵循法律法规和道德规范是非常重要的。应尊重网站的robots.txt文件中规定的爬虫规则,避免对网站造成过大压力。此外,使用爬取的数据时,避免用于商业用途,尤其是未经授权的情况下,以免侵犯知识产权或违反服务条款。
如何处理爬取亚马逊网页时遇到的反爬虫机制?
亚马逊采用多种反爬虫机制来保护其数据,常见的包括IP封锁、验证码和动态内容加载等。为应对这些挑战,可以使用代理服务器来更换IP地址,使用selenium等工具模拟人工操作,或通过引入延时机制来降低请求频率,从而减少被检测的风险。确保使用时遵循相关法律法规。
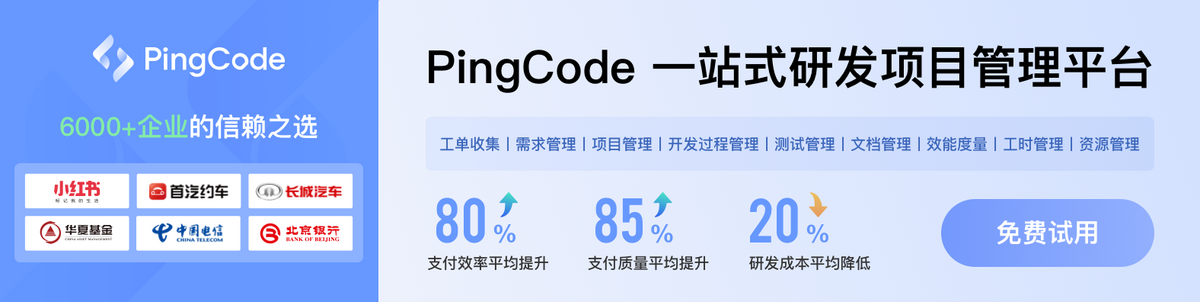