Python 拷贝文件夹的方法有多种,包括使用shutil模块、os模块等。推荐使用shutil模块,因为它提供了方便的高级文件操作函数,比如copytree()。
在详细描述之前,先简单回答标题问题:可以使用shutil模块、copytree()函数来拷贝一个文件夹。shutil.copytree()函数是Python中最常用来拷贝整个文件夹及其所有内容的方法之一。下面将详细介绍如何使用它。
一、shutil模块的介绍
shutil
是Python的一个高层次的文件操作模块。它提供了许多用于文件和目录操作的高级函数,适用于拷贝、移动、重命名和删除文件及目录。以下是shutil模块的一些常见函数:
- shutil.copy() – 拷贝文件内容和权限,但不包括文件的元数据。
- shutil.copy2() – 拷贝文件内容和元数据。
- shutil.copytree() – 递归地拷贝整个目录树。
- shutil.move() – 移动文件或目录。
- shutil.rmtree() – 递归地删除目录树。
由于我们需要拷贝整个文件夹,因此shutil.copytree()
是最合适的选择。
二、使用shutil.copytree()拷贝文件夹
shutil.copytree()
函数可以将源目录及其所有内容递归地拷贝到目标目录。以下是其基本用法:
import shutil
定义源目录和目标目录
src_dir = 'path/to/source/folder'
dst_dir = 'path/to/destination/folder'
拷贝整个目录树
shutil.copytree(src_dir, dst_dir)
详细说明:
src_dir
是源目录的路径。dst_dir
是目标目录的路径。如果目标目录不存在,shutil.copytree()
会自动创建它。
下面是一个完整的示例:
import shutil
import os
def copy_folder(src, dst):
"""
拷贝源目录及其所有内容到目标目录
:param src: 源目录路径
:param dst: 目标目录路径
"""
# 检查源目录是否存在
if not os.path.exists(src):
print(f"源目录 {src} 不存在")
return
# 如果目标目录存在,提示用户是否覆盖
if os.path.exists(dst):
print(f"目标目录 {dst} 已存在,是否覆盖?")
user_input = input("输入 'y' 覆盖,输入其他键取消: ")
if user_input.lower() != 'y':
print("操作取消")
return
# 删除目标目录
shutil.rmtree(dst)
# 执行拷贝操作
shutil.copytree(src, dst)
print(f"成功拷贝 {src} 到 {dst}")
示例调用
source_folder = 'example_source_folder'
destination_folder = 'example_destination_folder'
copy_folder(source_folder, destination_folder)
三、处理文件夹中的符号链接
在拷贝目录树时,可能会遇到符号链接。默认情况下,shutil.copytree()
会拷贝符号链接本身,而不是它们指向的文件或目录。如果希望拷贝符号链接指向的实际内容,可以使用copy_function
参数:
import shutil
def copy_function(src, dst, *, follow_symlinks=True):
if follow_symlinks:
shutil.copy2(src, dst)
else:
shutil.copy(src, dst)
src_dir = 'path/to/source/folder'
dst_dir = 'path/to/destination/folder'
shutil.copytree(src_dir, dst_dir, copy_function=copy_function)
四、使用ignore参数排除特定文件或文件夹
有时可能需要在拷贝文件夹时排除某些文件或文件夹。可以使用ignore
参数:
import shutil
def ignore_patterns(*patterns):
def _ignore_patterns(path, names):
import fnmatch
ignored_names = set()
for pattern in patterns:
ignored_names.update(fnmatch.filter(names, pattern))
return ignored_names
return _ignore_patterns
src_dir = 'path/to/source/folder'
dst_dir = 'path/to/destination/folder'
排除所有以 .tmp 结尾的文件
shutil.copytree(src_dir, dst_dir, ignore=ignore_patterns('*.tmp'))
五、处理权限错误
在拷贝文件夹时,可能会遇到权限问题。可以使用onerror
参数来处理这些错误:
import shutil
import os
import stat
def handle_remove_readonly(func, path, exc):
excvalue = exc[1]
if func in (os.rmdir, os.remove) and excvalue.errno == errno.EACCES:
os.chmod(path, stat.S_IRWXU | stat.S_IWUSR)
func(path)
else:
raise
src_dir = 'path/to/source/folder'
dst_dir = 'path/to/destination/folder'
shutil.copytree(src_dir, dst_dir, onerror=handle_remove_readonly)
六、总结
通过上文的详细描述,我们可以看到,在Python中拷贝一个文件夹最简单和高效的方法是使用shutil.copytree()
函数。这个函数不仅可以拷贝整个目录树,还可以处理符号链接、排除特定文件或文件夹,并处理权限错误。通过灵活使用shutil
模块,我们可以轻松地实现各种文件和目录操作。
相关问答FAQs:
如何在Python中复制文件夹及其内容?
使用Python可以通过shutil模块来实现文件夹的复制。shutil.copytree()函数可以将整个文件夹及其所有子文件夹和文件复制到指定的目标位置。确保目标位置不存在同名文件夹,否则会引发错误。以下是一个简单的示例代码:
import shutil
shutil.copytree('源文件夹路径', '目标文件夹路径')
使用Python复制文件夹时需要注意哪些事项?
在复制文件夹时,要确保源路径正确且目标路径不与现存文件夹冲突。此外,确保有足够的权限访问源文件夹和写入目标文件夹。对于大文件夹的复制,建议使用try-except语句来捕获可能的异常,以防止程序崩溃。
Python中复制文件夹的其他可选方法是什么?
除了shutil模块,os模块和pathlib模块也可以用于文件夹操作。例如,os模块中的os.listdir()结合os.makedirs()和文件的shutil.copy()可以实现更灵活的复制操作。这种方法适合需要自定义复制逻辑的场景。以下是一个示例:
import os
import shutil
def copy_folder(src, dst):
if not os.path.exists(dst):
os.makedirs(dst)
for item in os.listdir(src):
s = os.path.join(src, item)
d = os.path.join(dst, item)
if os.path.isdir(s):
copy_folder(s, d)
else:
shutil.copy2(s, d)
copy_folder('源文件夹路径', '目标文件夹路径')
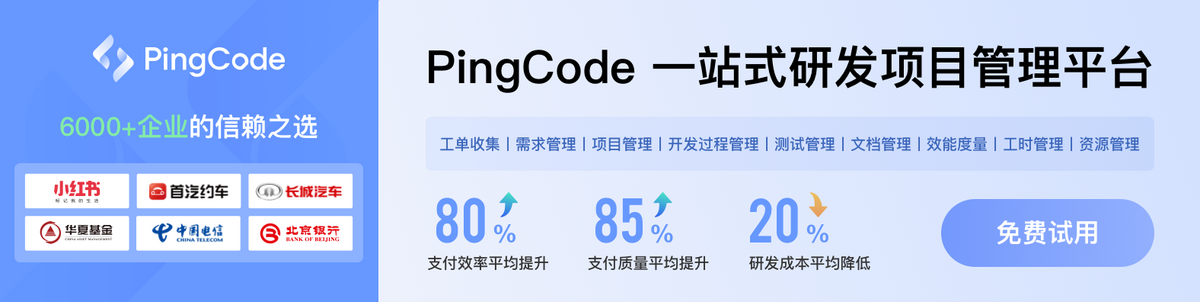