通过Python下载图片链接的步骤
要通过Python下载图片链接,可以使用以下几个步骤:导入所需库、获取图片链接、下载图片、保存图片。其中,导入所需库是最基本的步骤,使用requests库可以方便地获取图片链接,最后使用PIL库保存图片,这些步骤将保证图片下载过程顺利进行。接下来我们将详细解释每一个步骤。
一、导入所需库
在开始下载图片之前,首先需要确保导入了所需的Python库。常用的库包括requests
和PIL
(Python Imaging Library)。如果这些库尚未安装,可以使用pip进行安装。
import requests
from PIL import Image
from io import BytesIO
二、获取图片链接
在这一阶段,我们需要获取图片的URL链接。这个链接通常是一个字符串,表示网络上图片的位置。可以通过手动输入URL或者通过网络爬虫等方式获取。
url = 'https://example.com/image.jpg'
三、下载图片
使用requests库发送HTTP请求并获取图片内容。我们可以使用requests.get
方法发送GET请求,获取图片的二进制数据。
response = requests.get(url)
if response.status_code == 200:
img_data = response.content
else:
raise Exception("Failed to retrieve image")
四、保存图片
最后一步是将图片保存到本地磁盘。我们可以使用PIL库将二进制数据转换为图像对象,并使用save
方法保存图像。
img = Image.open(BytesIO(img_data))
img.save('downloaded_image.jpg')
五、代码示例
以下是一个完整的代码示例,展示了如何通过Python下载图片链接并保存图片。
import requests
from PIL import Image
from io import BytesIO
def download_image(url, save_path):
response = requests.get(url)
if response.status_code == 200:
img_data = response.content
img = Image.open(BytesIO(img_data))
img.save(save_path)
print(f"Image successfully downloaded: {save_path}")
else:
print("Failed to retrieve image")
示例使用
url = 'https://example.com/image.jpg'
save_path = 'downloaded_image.jpg'
download_image(url, save_path)
六、处理错误和异常
在实际应用中,可能会遇到各种错误和异常。例如,网络连接问题、无效的URL、图片格式不支持等。为了提高代码的鲁棒性,可以添加错误处理机制。
def download_image(url, save_path):
try:
response = requests.get(url)
response.raise_for_status() # 检查HTTP请求是否成功
img_data = response.content
img = Image.open(BytesIO(img_data))
img.save(save_path)
print(f"Image successfully downloaded: {save_path}")
except requests.exceptions.RequestException as e:
print(f"Failed to retrieve image: {e}")
except IOError as e:
print(f"Failed to save image: {e}")
七、批量下载图片
如果需要下载多个图片,可以将这些URL存储在列表中,并使用循环遍历每个URL进行下载。以下是批量下载图片的示例代码:
def download_images(urls, save_dir):
for i, url in enumerate(urls):
save_path = f"{save_dir}/image_{i+1}.jpg"
download_image(url, save_path)
示例使用
urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
]
save_dir = 'downloaded_images'
download_images(urls, save_dir)
八、并发下载图片
为了提高下载效率,可以使用并发技术。例如,可以使用concurrent.futures
模块实现多线程下载。
import concurrent.futures
def download_images_concurrently(urls, save_dir):
with concurrent.futures.ThreadPoolExecutor() as executor:
futures = [executor.submit(download_image, url, f"{save_dir}/image_{i+1}.jpg") for i, url in enumerate(urls)]
for future in concurrent.futures.as_completed(futures):
try:
future.result()
except Exception as e:
print(f"Error occurred: {e}")
示例使用
urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
]
save_dir = 'downloaded_images'
download_images_concurrently(urls, save_dir)
九、总结
通过上述步骤,我们可以使用Python方便地下载图片链接并保存图片。关键步骤包括导入所需库、获取图片链接、下载图片和保存图片。为了提高代码的鲁棒性,可以添加错误处理机制,并可以通过批量和并发下载提高下载效率。希望这些详细的解释和代码示例能帮助你更好地理解和实现图片下载功能。
十、扩展应用
在实际应用中,下载图片可能只是整个数据处理流程的一部分。例如,可能需要对下载的图片进行进一步的处理,如图像识别、图像增强等。以下是一些扩展应用的示例:
1、图像识别
可以使用OpenCV或TensorFlow等库对下载的图片进行图像识别。
import cv2
def recognize_image(image_path):
img = cv2.imread(image_path)
# 使用OpenCV进行图像识别(例如人脸检测)
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imwrite('recognized_image.jpg', img)
print("Image recognition completed.")
示例使用
recognize_image('downloaded_image.jpg')
2、图像增强
可以使用PIL或OpenCV对下载的图片进行图像增强。
from PIL import ImageEnhance
def enhance_image(image_path):
img = Image.open(image_path)
enhancer = ImageEnhance.Contrast(img)
enhanced_img = enhancer.enhance(2.0)
enhanced_img.save('enhanced_image.jpg')
print("Image enhancement completed.")
示例使用
enhance_image('downloaded_image.jpg')
3、图像分类
可以使用深度学习模型对下载的图片进行图像分类。
import tensorflow as tf
def classify_image(image_path):
model = tf.keras.applications.MobileNetV2(weights='imagenet')
img = tf.keras.preprocessing.image.load_img(image_path, target_size=(224, 224))
img_array = tf.keras.preprocessing.image.img_to_array(img)
img_array = tf.expand_dims(img_array, 0)
predictions = model.predict(img_array)
decoded_predictions = tf.keras.applications.mobilenet_v2.decode_predictions(predictions, top=1)
print("Image classification completed:", decoded_predictions)
示例使用
classify_image('downloaded_image.jpg')
这些扩展应用展示了如何在下载图片后进行进一步的处理,以实现更复杂的功能。希望这些示例能为你的项目提供更多的灵感和帮助。
相关问答FAQs:
如何使用Python下载网络图片?
您可以使用Python中的requests库轻松下载网络图片。只需发送GET请求获取图片数据,然后将其写入本地文件。示例代码如下:
import requests
url = '图片链接'
response = requests.get(url)
if response.status_code == 200:
with open('图片名称.jpg', 'wb') as f:
f.write(response.content)
确保安装requests库,可以通过命令pip install requests
进行安装。
在下载图片时如何处理错误?
在下载图片时,可能会遇到各种错误,比如网络问题或无效链接。通过检查响应状态码,可以有效处理这些错误。例如,您可以在下载之前验证链接的有效性,并在请求失败时使用异常处理来捕获错误信息。
下载大图片时如何优化内存使用?
下载大图片时,一次性将所有数据加载到内存中可能导致内存不足。可以通过流式下载的方式逐块读取数据,使用stream=True
参数来实现。下面是一个流式下载的示例:
response = requests.get(url, stream=True)
if response.status_code == 200:
with open('图片名称.jpg', 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
f.write(chunk)
这种方式可以有效地降低内存占用,适合处理较大的图片文件。
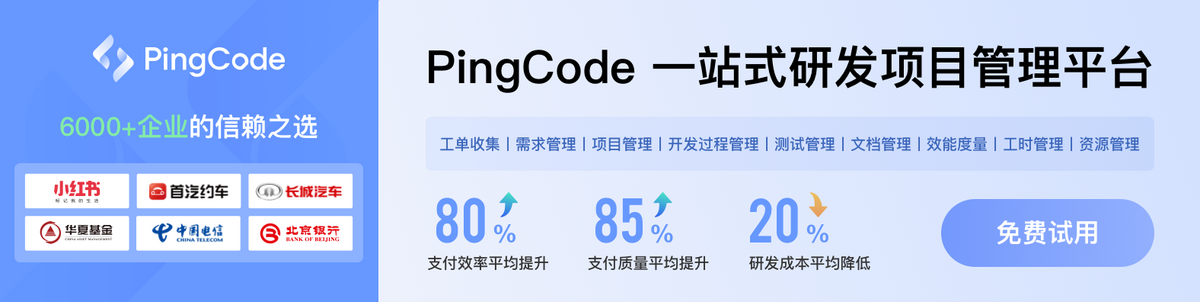