将原始数据进行平滑处理的方法包括:使用移动平均法、使用指数平滑法、使用小波变换、使用低通滤波器。 其中,移动平均法是最简单且常用的平滑方法之一。移动平均法通过计算数据在一定窗口内的平均值来平滑数据,从而减小数据中的噪声和波动。具体来说,移动平均法有简单移动平均(SMA)、加权移动平均(WMA)和指数移动平均(EMA)等多种变体。本文将详细介绍这些方法以及如何在Python中实现它们。
一、移动平均法
移动平均法是一种通过取一段时间窗口内数据的平均值来平滑数据的技术。主要有以下几种变体:
1、简单移动平均(SMA)
简单移动平均(Simple Moving Average, SMA)是最基础的移动平均方法。在SMA中,每个数据点的值是其前N个数据点的平均值。假设我们有一个数据序列[x_1, x_2, …, x_t],SMA的计算公式为:
[SMA_t = \frac{1}{N} \sum_{i=t-N+1}^{t} x_i]
其中N是窗口大小。
Python实现
import numpy as np
def simple_moving_average(data, window_size):
return np.convolve(data, np.ones(window_size)/window_size, mode='valid')
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
window_size = 3
sma = simple_moving_average(data, window_size)
print(sma)
2、加权移动平均(WMA)
加权移动平均(Weighted Moving Average, WMA)在计算平均值时对不同的数据点赋予不同的权重。通常,较新的数据点权重较大。
Python实现
def weighted_moving_average(data, weights):
window_size = len(weights)
return np.convolve(data, weights[::-1], mode='valid')
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
weights = [0.1, 0.2, 0.3, 0.4] # 权重之和应为1
wma = weighted_moving_average(data, weights)
print(wma)
3、指数移动平均(EMA)
指数移动平均(Exponential Moving Average, EMA)是一种递归计算的移动平均方法。EMA对较新的数据点赋予更大的权重,使得其对数据的变化更加敏感。EMA的计算公式为:
[EMA_t = \alpha \cdot x_t + (1 – \alpha) \cdot EMA_{t-1}]
其中,(\alpha) 是平滑因子,通常取 (\alpha = \frac{2}{N+1}),N为窗口大小。
Python实现
def exponential_moving_average(data, window_size):
alpha = 2 / (window_size + 1)
ema = [data[0]] # 初始值为第一个数据点
for i in range(1, len(data)):
ema.append(alpha * data[i] + (1 - alpha) * ema[-1])
return np.array(ema)
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
window_size = 3
ema = exponential_moving_average(data, window_size)
print(ema)
二、指数平滑法
指数平滑法是一种加权平均方法,类似于EMA,但其权重随时间指数递减。指数平滑法分为单指数平滑、双指数平滑和三指数平滑。
1、单指数平滑(SES)
单指数平滑(Single Exponential Smoothing, SES)是对数据进行一次指数平滑。其计算公式与EMA类似。
Python实现
def single_exponential_smoothing(data, alpha):
ses = [data[0]] # 初始值为第一个数据点
for i in range(1, len(data)):
ses.append(alpha * data[i] + (1 - alpha) * ses[-1])
return np.array(ses)
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
alpha = 0.5
ses = single_exponential_smoothing(data, alpha)
print(ses)
2、双指数平滑(DES)
双指数平滑(Double Exponential Smoothing, DES)用于处理具有趋势的数据。它通过对一次指数平滑的结果再次进行指数平滑来捕捉趋势。
Python实现
def double_exponential_smoothing(data, alpha, beta):
ses = [data[0]] # 初始值为第一个数据点
trend = [data[1] - data[0]] # 初始趋势
des = [ses[0] + trend[0]]
for i in range(1, len(data)):
ses.append(alpha * data[i] + (1 - alpha) * (ses[-1] + trend[-1]))
trend.append(beta * (ses[-1] - ses[-2]) + (1 - beta) * trend[-1])
des.append(ses[-1] + trend[-1])
return np.array(des)
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
alpha = 0.5
beta = 0.5
des = double_exponential_smoothing(data, alpha, beta)
print(des)
3、三指数平滑(TES)
三指数平滑(Triple Exponential Smoothing, TES)用于处理具有季节性变化的数据。它通过对双指数平滑的结果再次进行指数平滑来捕捉季节性变化。
Python实现
from statsmodels.tsa.holtwinters import ExponentialSmoothing
def triple_exponential_smoothing(data, seasonal_periods, trend='add', seasonal='add'):
model = ExponentialSmoothing(data, trend=trend, seasonal=seasonal, seasonal_periods=seasonal_periods)
fit = model.fit()
return fit.fittedvalues
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
seasonal_periods = 4
tes = triple_exponential_smoothing(data, seasonal_periods)
print(tes)
三、小波变换
小波变换是一种时频分析工具,可以用于平滑和降噪。通过将数据分解成不同的频率成分,然后对高频成分进行平滑处理,可以实现数据的平滑处理。
Python实现
import pywt
def wavelet_smoothing(data, wavelet='db1', level=1):
coeff = pywt.wavedec(data, wavelet, mode='symmetric')
sigma = (1/0.6745) * np.median(np.abs(coeff[-level] - np.median(coeff[-level])))
uthresh = sigma * np.sqrt(2 * np.log(len(data)))
coeff[1:] = (pywt.threshold(i, value=uthresh, mode='soft') for i in coeff[1:])
return pywt.waverec(coeff, wavelet, mode='symmetric')
示例
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
smoothed_data = wavelet_smoothing(data)
print(smoothed_data)
四、低通滤波器
低通滤波器是一种信号处理工具,用于滤除高频噪声信号,从而达到平滑数据的目的。常用的低通滤波器有Butterworth滤波器、Chebyshev滤波器等。
1、Butterworth滤波器
Butterworth滤波器是一种常用的低通滤波器,其频率响应曲线在通带内是平坦的。
Python实现
from scipy.signal import butter, filtfilt
def butterworth_lowpass_filter(data, cutoff, fs, order=4):
nyquist = 0.5 * fs
normal_cutoff = cutoff / nyquist
b, a = butter(order, normal_cutoff, btype='low', analog=False)
y = filtfilt(b, a, data)
return y
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
cutoff = 2.5 # 截止频率
fs = 10 # 采样频率
filtered_data = butterworth_lowpass_filter(data, cutoff, fs)
print(filtered_data)
2、Chebyshev滤波器
Chebyshev滤波器是一种可以更好地控制通带和阻带特性的滤波器。Chebyshev滤波器有I型和II型两种,I型在通带内有波纹,而II型在阻带内有波纹。
Python实现
from scipy.signal import cheby1
def chebyshev_lowpass_filter(data, cutoff, fs, order=4, rp=0.1):
nyquist = 0.5 * fs
normal_cutoff = cutoff / nyquist
b, a = cheby1(order, rp, normal_cutoff, btype='low', analog=False)
y = filtfilt(b, a, data)
return y
示例
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
cutoff = 2.5 # 截止频率
fs = 10 # 采样频率
filtered_data = chebyshev_lowpass_filter(data, cutoff, fs)
print(filtered_data)
总结
平滑处理是数据预处理中一个重要的步骤,通过平滑处理可以有效地减少数据中的噪声,增强信号的可解释性。本文介绍了几种常用的平滑处理方法,包括移动平均法、指数平滑法、小波变换和低通滤波器,并提供了详细的Python实现代码。这些方法各有优缺点,选择合适的方法需要根据具体的数据特性和应用场景来决定。
相关问答FAQs:
平滑处理的目的是什么?
平滑处理的主要目的是减少数据中的噪声,从而使趋势和模式更加明显。这对于数据分析和可视化非常重要,特别是在时间序列分析中,平滑可以帮助我们更好地理解数据的长期行为。
在Python中有哪些常用的平滑处理方法?
Python提供了多种平滑处理的方法,包括移动平均、指数平滑和Savitzky-Golay滤波器等。移动平均是最常用的方法之一,它通过取连续数据点的平均值来减少波动。而指数平滑则通过给最近的数据点更高的权重来进行平滑,适合处理具有趋势性的数据。Savitzky-Golay滤波器则可以在保持数据特征的同时平滑数据,常用于信号处理。
如何选择合适的平滑参数?
选择合适的平滑参数通常需要考虑数据的特性和分析目的。例如,在使用移动平均法时,需要选择窗口大小,窗口过大会导致过度平滑,重要信息可能会丢失;窗口过小则可能无法有效去除噪声。可以通过交叉验证等技术来评估不同参数的效果,找到最合适的参数设置。
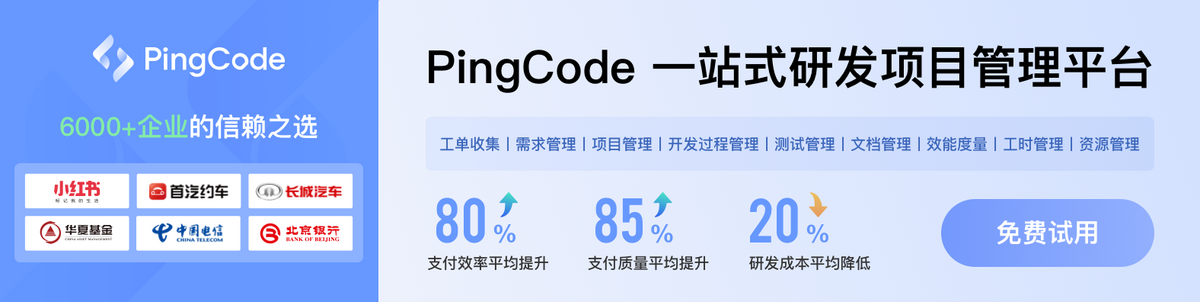