如何用Python构建机器人的路径规划
Python提供了多种方法来实现机器人路径规划,例如A算法、Dijkstra算法、基于概率的快速扩展随机树(RRT)等。 在这些方法中,A算法因其高效性和简单性,常被广泛应用。A*算法结合了启发式搜索和代价最小的路径搜索,使其能够快速找到从起点到终点的最优路径。
一、A*算法
A算法是路径规划中最常用的算法之一。它通过评估每个节点的代价(从起点到节点的实际代价加上从节点到终点的估计代价)来选择最优路径。A算法具有高效性和准确性。以下是详细的实现步骤和代码示例。
1.1、基本原理
A*算法的核心在于启发式函数,它结合了实际代价和估计代价,使得搜索更加高效。启发式函数一般采用曼哈顿距离或欧几里得距离。
1.2、实现步骤
- 初始化: 创建一个开放列表和一个封闭列表。开放列表用于存储待评估的节点,封闭列表用于存储已评估的节点。
- 选择节点: 从开放列表中选择代价最小的节点。
- 评估节点: 评估当前节点的邻居节点。如果邻居节点未在封闭列表中,则计算其代价并添加到开放列表中。
- 更新路径: 如果找到终点,则更新路径并返回;否则,继续从开放列表中选择下一个节点。
1.3、代码示例
import heapq
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 # Distance from start to node
self.h = 0 # Heuristic - estimated distance from node to end
self.f = 0 # Total cost
def __lt__(self, other):
return self.f < other.f
def astar(maze, start, end):
open_list = []
closed_list = set()
start_node = Node(start)
end_node = Node(end)
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node.position)
if current_node.position == end:
path = []
while current_node:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
neighbors = [(0, -1), (0, 1), (-1, 0), (1, 0)]
for new_position in neighbors:
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
if node_position[0] > (len(maze) - 1) or node_position[0] < 0 or node_position[1] > (len(maze[len(maze)-1]) - 1) or node_position[1] < 0:
continue
if maze[node_position[0]][node_position[1]] != 0:
continue
new_node = Node(node_position, current_node)
if new_node.position in closed_list:
continue
new_node.g = current_node.g + 1
new_node.h = ((new_node.position[0] - end_node.position[0]) <strong> 2) + ((new_node.position[1] - end_node.position[1]) </strong> 2)
new_node.f = new_node.g + new_node.h
if add_to_open(open_list, new_node):
heapq.heappush(open_list, new_node)
return None
def add_to_open(open_list, new_node):
for node in open_list:
if new_node == node and new_node.f >= node.f:
return False
return True
maze = [
[0, 1, 0, 0, 0, 0, 0],
[0, 1, 0, 1, 1, 1, 0],
[0, 0, 0, 1, 0, 0, 0],
[0, 1, 1, 1, 0, 1, 0],
[0, 0, 0, 0, 0, 0, 0]
]
start = (0, 0)
end = (4, 6)
path = astar(maze, start, end)
print(path)
二、Dijkstra算法
Dijkstra算法是一种经典的图搜索算法,适用于加权图中的最短路径问题。它通过逐步扩展最短路径树来找到从起点到终点的最短路径。
2.1、基本原理
Dijkstra算法通过优先级队列(如最小堆)来管理待处理的节点。每次从队列中取出代价最小的节点,更新其邻居节点的代价,并将未处理的邻居节点加入队列。
2.2、实现步骤
- 初始化: 创建一个优先级队列和一个距离字典。优先级队列用于存储待处理的节点,距离字典存储从起点到每个节点的最短距离。
- 选择节点: 从优先级队列中选择距离最小的节点。
- 更新距离: 计算当前节点的邻居节点的距离,并更新距离字典和优先级队列。
- 返回路径: 如果找到终点,则返回路径;否则,继续从优先级队列中选择下一个节点。
2.3、代码示例
import heapq
def dijkstra(graph, start, end):
queue = [(0, start)]
distances = {start: 0}
parent = {start: None}
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_node == end:
path = []
while current_node is not None:
path.append(current_node)
current_node = parent[current_node]
return path[::-1]
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances.get(neighbor, float('inf')):
distances[neighbor] = distance
parent[neighbor] = current_node
heapq.heappush(queue, (distance, neighbor))
return None
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
start = 'A'
end = 'D'
path = dijkstra(graph, start, end)
print(path)
三、快速扩展随机树(RRT)
RRT是一种基于随机采样的路径规划算法,适用于高维空间中的路径规划问题。RRT通过随机生成节点并逐步连接这些节点,最终形成一棵树,从而找到从起点到终点的路径。
3.1、基本原理
RRT通过在空间中随机生成节点,并尝试将这些节点连接到现有树中。如果新的节点可以连接到树中且不与障碍物碰撞,则将其添加到树中,直到找到终点。
3.2、实现步骤
- 初始化: 创建一棵树,树的根节点为起点。
- 随机采样: 在空间中随机生成一个节点。
- 连接节点: 尝试将随机生成的节点连接到树中最接近的节点。
- 检查碰撞: 如果连接过程中没有碰撞,则将新节点添加到树中。
- 终止条件: 如果找到终点,则返回路径;否则,继续随机生成节点。
3.3、代码示例
import random
import math
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
def get_distance(node1, node2):
return math.sqrt((node1.x - node2.x) <strong> 2 + (node1.y - node2.y) </strong> 2)
def is_collision(node1, node2, obstacles):
for (ox, oy, size) in obstacles:
if get_distance(node1, Node(ox, oy)) < size or get_distance(node2, Node(ox, oy)) < size:
return True
return False
def rrt(start, end, obstacles, max_iter=500, step_size=1.0):
start_node = Node(start[0], start[1])
end_node = Node(end[0], end[1])
tree = [start_node]
for _ in range(max_iter):
random_node = Node(random.uniform(0, 10), random.uniform(0, 10))
nearest_node = min(tree, key=lambda node: get_distance(node, random_node))
theta = math.atan2(random_node.y - nearest_node.y, random_node.x - nearest_node.x)
new_node = Node(nearest_node.x + step_size * math.cos(theta), nearest_node.y + step_size * math.sin(theta))
new_node.parent = nearest_node
if not is_collision(nearest_node, new_node, obstacles):
tree.append(new_node)
if get_distance(new_node, end_node) < step_size:
end_node.parent = new_node
tree.append(end_node)
path = []
current_node = end_node
while current_node is not None:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
return None
start = (0, 0)
end = (9, 9)
obstacles = [(5, 5, 1), (3, 6, 1)]
path = rrt(start, end, obstacles)
print(path)
四、基于强化学习的路径规划
强化学习是一种机器学习方法,通过与环境的交互来学习最优策略。在路径规划中,可以使用Q-learning或深度Q-learning(DQN)来实现机器人路径规划。
4.1、基本原理
强化学习通过与环境的交互,更新Q表或神经网络的权重,以学习最优策略。Q表记录了每个状态-动作对的价值,而DQN使用神经网络来逼近Q值函数。
4.2、实现步骤
- 初始化: 初始化Q表或神经网络,并设置学习参数。
- 探索和利用: 机器人在环境中执行动作,依据ε-greedy策略选择动作,即有一定概率随机选择动作(探索),其余时间选择最优动作(利用)。
- 更新策略: 根据奖励和下一状态的Q值,更新Q表或神经网络的权重。
- 终止条件: 如果机器人到达终点或达到最大步数,则终止学习。
4.3、代码示例(Q-learning)
import numpy as np
import random
class QLearning:
def __init__(self, maze, start, end, alpha=0.1, gamma=0.9, epsilon=0.1):
self.maze = maze
self.start = start
self.end = end
self.alpha = alpha
self.gamma = gamma
self.epsilon = epsilon
self.q_table = np.zeros((*maze.shape, 4))
def is_valid(self, state):
x, y = state
return 0 <= x < self.maze.shape[0] and 0 <= y < self.maze.shape[1] and self.maze[x, y] == 0
def get_next_state(self, state, action):
x, y = state
if action == 0: # Up
return x - 1, y
elif action == 1: # Down
return x + 1, y
elif action == 2: # Left
return x, y - 1
elif action == 3: # Right
return x, y + 1
def learn(self, episodes=1000, max_steps=100):
for _ in range(episodes):
state = self.start
for _ in range(max_steps):
if random.uniform(0, 1) < self.epsilon:
action = random.randint(0, 3)
else:
action = np.argmax(self.q_table[state])
next_state = self.get_next_state(state, action)
if not self.is_valid(next_state):
continue
reward = 1 if next_state == self.end else -1
next_max = np.max(self.q_table[next_state])
self.q_table[state][action] = (1 - self.alpha) * self.q_table[state][action] + self.alpha * (reward + self.gamma * next_max)
state = next_state
if state == self.end:
break
def get_path(self):
state = self.start
path = [state]
while state != self.end:
action = np.argmax(self.q_table[state])
state = self.get_next_state(state, action)
path.append(state)
return path
maze = np.array([
[0, 1, 0, 0, 0, 0, 0],
[0, 1, 0, 1, 1, 1, 0],
[0, 0, 0, 1, 0, 0, 0],
[0, 1, 1, 1, 0, 1, 0],
[0, 0, 0, 0, 0, 0, 0]
])
start = (0, 0)
end = (4, 6)
ql = QLearning(maze, start, end)
ql.learn()
path = ql.get_path()
print(path)
总结
用Python构建机器人的路径规划有很多方法,每种方法都有其优缺点和适用场景。A*算法适用于网格地图中的最优路径搜索,Dijkstra算法适用于加权图中的最短路径搜索,RRT适用于高维空间中的路径规划,强化学习适用于动态和复杂环境中的路径规划。选择合适的方法不仅能提高路径规划的效率,还能更好地应对不同的应用场景。
相关问答FAQs:
如何在Python中实现机器人路径规划的基本步骤是什么?
在Python中实现机器人路径规划通常包括几个关键步骤:首先,选择合适的算法,比如A*、Dijkstra或RRT等。接着,构建环境地图,确定起点和终点的位置。然后,利用选定的算法进行路径计算,生成从起点到终点的最优路径。最后,可以通过可视化工具(如Matplotlib)展示路径和环境的交互情况,以便进行调试和优化。
对于初学者,有哪些Python库可以帮助路径规划?
对于初学者,推荐使用一些流行的Python库,如numpy
和matplotlib
用于基本的数学运算和可视化,scipy
用于科学计算,networkx
用于图形和网络分析。此外,pygame
可以用于创建可交互的2D游戏环境,便于模拟机器人的运动和路径规划。还有一些专门的机器人库,如ROS
(Robot Operating System)也可以用于更复杂的机器人项目。
如何评估路径规划算法的效率和效果?
评估路径规划算法的效率和效果可以从多个方面入手。首先,可以通过计算路径长度和计算时间来评估算法的性能。其次,路径的平滑度和可行性也是重要的指标,路径不应出现过多的急转弯。此外,可以在不同的环境复杂性下测试算法的表现,观察其在动态障碍物和变化环境中的适应能力。最后,使用不同的评估标准和指标(如成功率、碰撞次数)进行多次实验,可以更全面地了解算法的优劣。
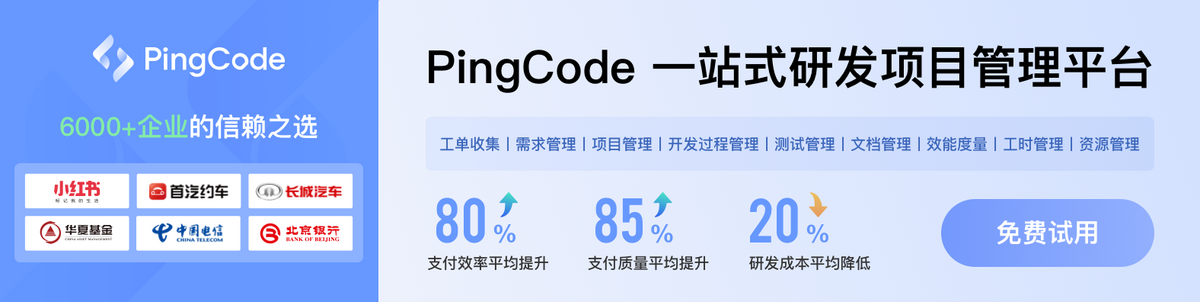