在Python中,中断多线程的方法有:使用标志变量、使用threading.Event
对象、使用Thread.join
方法、使用守护线程。这些方法可以协同工作来确保线程能够被安全地中断。下面将详细介绍如何使用标志变量中断多线程。
使用标志变量是一种常见的方法,它通过在线程中定期检查某个共享变量的值来确定是否应该退出。以下是详细的介绍:
标志变量
在多线程编程中,使用标志变量是一种简单而有效的方法来控制线程的终止。标志变量通常是一个布尔值,当主线程希望子线程停止工作时,会将这个标志变量设置为True
。子线程在运行过程中会定期检查这个标志变量的值,如果发现其值为True
,则会中断自身的工作并退出。
以下是一个示例代码:
import threading
import time
定义一个全局的标志变量
stop_thread = False
def thread_function():
while not stop_thread:
print("Thread is running")
time.sleep(1)
print("Thread is stopping")
创建并启动线程
thread = threading.Thread(target=thread_function)
thread.start()
主线程等待5秒,然后设置标志变量为True
time.sleep(5)
stop_thread = True
等待子线程结束
thread.join()
print("Main thread is exiting")
在这个示例中,stop_thread
是一个全局变量,初始值为False
。子线程在运行过程中会不断检查stop_thread
的值,如果发现其值变为True
,则会终止循环并退出。在主线程中,等待5秒后将stop_thread
设置为True
,从而通知子线程停止工作。
其他方法
除了使用标志变量,还有其他一些常用的方法来中断多线程:
使用threading.Event
对象
threading.Event
对象可以用于在线程之间通信。与标志变量不同,Event
对象提供了更高层次的控制。它有两个主要方法:set()
和clear()
,用于设置和清除事件标志;还有一个wait()
方法,线程可以调用它来等待事件发生。
以下是一个示例代码:
import threading
import time
创建一个Event对象
stop_event = threading.Event()
def thread_function():
while not stop_event.is_set():
print("Thread is running")
time.sleep(1)
print("Thread is stopping")
创建并启动线程
thread = threading.Thread(target=thread_function)
thread.start()
主线程等待5秒,然后设置事件
time.sleep(5)
stop_event.set()
等待子线程结束
thread.join()
print("Main thread is exiting")
在这个示例中,stop_event
是一个Event
对象。子线程通过调用stop_event.is_set()
来检查事件是否已经设置。如果事件被设置,子线程将终止循环并退出。在主线程中,等待5秒后调用stop_event.set()
,从而通知子线程停止工作。
使用Thread.join
方法
Thread.join
方法可以用于等待线程的结束。当主线程调用join
方法时,它会阻塞,直到子线程结束。在某些情况下,可以结合标志变量或Event
对象来实现线程的中断。
以下是一个示例代码:
import threading
import time
定义一个全局的标志变量
stop_thread = False
def thread_function():
while not stop_thread:
print("Thread is running")
time.sleep(1)
print("Thread is stopping")
创建并启动线程
thread = threading.Thread(target=thread_function)
thread.start()
主线程等待5秒,然后设置标志变量为True
time.sleep(5)
stop_thread = True
等待子线程结束
thread.join()
print("Main thread is exiting")
在这个示例中,join
方法用于等待子线程的结束。主线程在设置标志变量为True
后,调用join
方法等待子线程结束。
使用守护线程
守护线程是一种特殊的线程,它会在主线程结束时自动退出。可以通过将线程的daemon
属性设置为True
来将其设置为守护线程。守护线程通常用于后台任务,不需要显式中断。
以下是一个示例代码:
import threading
import time
def thread_function():
while True:
print("Daemon thread is running")
time.sleep(1)
创建并启动守护线程
thread = threading.Thread(target=thread_function)
thread.daemon = True
thread.start()
主线程等待5秒
time.sleep(5)
print("Main thread is exiting")
在这个示例中,thread
被设置为守护线程。主线程在等待5秒后结束,守护线程也会自动退出。
总结
在Python中,中断多线程的方法有多种,包括使用标志变量、threading.Event
对象、Thread.join
方法和守护线程。选择哪种方法取决于具体的应用场景和需求。无论使用哪种方法,都需要确保线程的中断是安全的,不会导致资源泄漏或其他问题。使用标志变量和Event
对象是比较常见且简单的方法,而Thread.join
方法和守护线程适用于特定的情况。希望这些方法能够帮助你在实际应用中更好地管理多线程的中断。
相关问答FAQs:
如何在Python中优雅地终止一个线程?
在Python中,优雅地终止线程通常意味着要设置一个标志,让线程在完成当前任务后主动退出。可以使用threading
模块中的Event
对象来实现这一功能。通过在主线程中设置事件标志,工作线程可以在合适的时候检查这个标志,并在发现它被设置后安全地退出。
Python中是否有方法可以强制终止线程?
Python并没有提供直接强制终止线程的方式。这是因为强制终止可能会导致数据不一致或资源泄漏。最好的做法是通过设置标志或使用Event
对象来请求线程停止,并让线程根据需要自行清理并退出。
多线程中断会对程序的性能产生什么影响?
中断多线程会产生一定的性能开销,特别是在频繁创建和销毁线程的情况下。合理使用线程池或管理线程的生命周期可以有效减少这种影响。此外,确保线程安全和资源的正确释放也是保证性能的关键。因此,在设计多线程程序时,应合理规划线程的使用和中断方式,以优化性能。
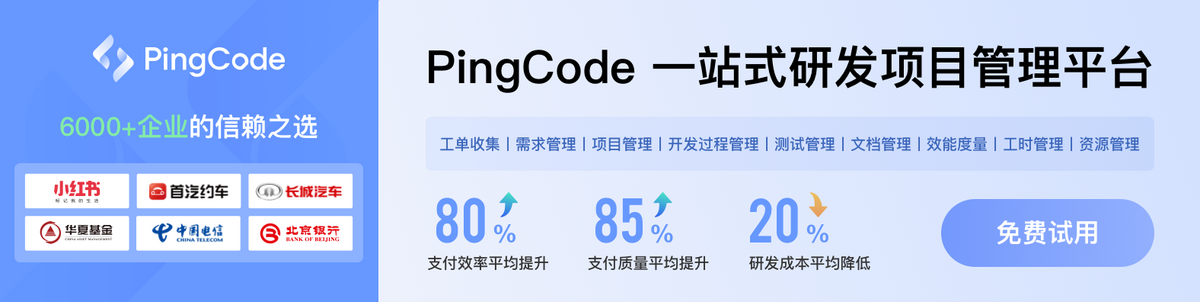