如何用Python调用文件内容
使用Python调用文件内容有多种方法,如使用内置的open
函数、通过pandas
库读取数据文件、使用os
库操作文件。 其中,使用open
函数是最基本也是最常用的方法,通过这种方法可以读取文本文件的内容。下面将详细介绍这种方法。
使用open
函数读取文件内容: 可以通过open
函数打开文件,并使用不同的模式(如读取模式r
、写入模式w
等)进行文件操作。以下是一个简单的示例:
# 打开文件
with open('example.txt', 'r') as file:
# 读取文件内容
content = file.read()
# 打印文件内容
print(content)
在这个示例中,with open('example.txt', 'r') as file:
打开了一个名为 example.txt
的文件,并将其内容读取到变量 content
中,然后打印出来。使用 with
关键字可以确保文件在使用完毕后自动关闭,避免资源泄漏。
一、使用open
函数读取文件
1.1 读取整个文件内容
使用 read()
方法可以读取整个文件的内容并将其作为一个字符串返回。这种方法适用于文件内容较小的情况。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
1.2 逐行读取文件内容
使用 readlines()
方法可以读取文件的每一行,并将其作为一个列表返回。每一行作为列表中的一个元素。
with open('example.txt', 'r') as file:
lines = file.readlines()
for line in lines:
print(line.strip())
1.3 逐行读取文件内容(迭代器方式)
可以直接对文件对象进行迭代,每次读取一行内容。这种方法适用于处理大文件,因为它不会一次性将整个文件内容读入内存。
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
二、使用pandas
库读取数据文件
2.1 读取CSV文件
pandas
是一个强大的数据分析库,提供了方便的方法来读取和操作数据文件。使用 read_csv()
方法可以读取CSV文件。
import pandas as pd
df = pd.read_csv('example.csv')
print(df.head())
2.2 读取Excel文件
使用 read_excel()
方法可以读取Excel文件。
df = pd.read_excel('example.xlsx')
print(df.head())
2.3 读取JSON文件
使用 read_json()
方法可以读取JSON文件。
df = pd.read_json('example.json')
print(df.head())
三、使用os
库操作文件
3.1 获取文件列表
使用 os.listdir()
方法可以获取指定目录下的文件列表。
import os
files = os.listdir('.')
print(files)
3.2 检查文件是否存在
使用 os.path.exists()
方法可以检查文件是否存在。
if os.path.exists('example.txt'):
print('File exists')
else:
print('File does not exist')
3.3 获取文件大小
使用 os.path.getsize()
方法可以获取文件大小。
file_size = os.path.getsize('example.txt')
print(f'File size: {file_size} bytes')
四、使用pathlib
库操作文件
4.1 读取文件内容
pathlib
是Python 3.4引入的一个模块,提供了面向对象的文件系统路径操作方法。使用 Path
对象可以方便地读取文件内容。
from pathlib import Path
file_path = Path('example.txt')
content = file_path.read_text()
print(content)
4.2 写入文件内容
使用 write_text()
方法可以向文件写入内容。
file_path = Path('example.txt')
file_path.write_text('Hello, World!')
4.3 获取文件列表
使用 glob()
方法可以获取指定模式的文件列表。
from pathlib import Path
files = Path('.').glob('*.txt')
for file in files:
print(file)
4.4 检查文件是否存在
使用 exists()
方法可以检查文件是否存在。
file_path = Path('example.txt')
if file_path.exists():
print('File exists')
else:
print('File does not exist')
五、使用csv
库读取和写入CSV文件
5.1 读取CSV文件
csv
是Python内置的一个模块,用于读写CSV文件。使用 csv.reader()
方法可以读取CSV文件内容。
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
5.2 写入CSV文件
使用 csv.writer()
方法可以向CSV文件写入内容。
import csv
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles']
]
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
六、使用json
库读取和写入JSON文件
6.1 读取JSON文件
json
是Python内置的一个模块,用于处理JSON数据。使用 json.load()
方法可以读取JSON文件内容。
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
6.2 写入JSON文件
使用 json.dump()
方法可以向JSON文件写入内容。
import json
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.json', 'w') as file:
json.dump(data, file, indent=4)
七、使用pickle
库读取和写入二进制文件
7.1 读取二进制文件
pickle
是Python内置的一个模块,用于序列化和反序列化Python对象。使用 pickle.load()
方法可以读取二进制文件内容。
import pickle
with open('example.pkl', 'rb') as file:
data = pickle.load(file)
print(data)
7.2 写入二进制文件
使用 pickle.dump()
方法可以向二进制文件写入内容。
import pickle
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
with open('example.pkl', 'wb') as file:
pickle.dump(data, file)
八、使用io
库操作内存中的文件
8.1 读取内存中的文件
io
是Python内置的一个模块,提供了操作内存中文件的方法。使用 io.StringIO
可以操作内存中的文本文件。
import io
file = io.StringIO('Hello, World!')
content = file.read()
print(content)
8.2 写入内存中的文件
使用 io.StringIO
可以向内存中的文本文件写入内容。
import io
file = io.StringIO()
file.write('Hello, World!')
content = file.getvalue()
print(content)
8.3 操作内存中的二进制文件
使用 io.BytesIO
可以操作内存中的二进制文件。
import io
file = io.BytesIO(b'Hello, World!')
content = file.read()
print(content)
九、使用shutil
库操作文件
9.1 复制文件
shutil
是Python内置的一个模块,提供了高级的文件操作方法。使用 shutil.copy()
可以复制文件。
import shutil
shutil.copy('example.txt', 'copy_example.txt')
9.2 移动文件
使用 shutil.move()
可以移动文件。
import shutil
shutil.move('example.txt', 'moved_example.txt')
9.3 删除文件
使用 os.remove()
可以删除文件。
import os
os.remove('example.txt')
十、处理大文件
10.1 分块读取文件
对于大文件,可以分块读取文件内容,避免一次性将整个文件读入内存。
def read_in_chunks(file_object, chunk_size=1024):
while True:
data = file_object.read(chunk_size)
if not data:
break
yield data
with open('large_file.txt', 'r') as file:
for chunk in read_in_chunks(file):
print(chunk)
10.2 使用迭代器处理大文件
可以使用文件对象的迭代器特性逐行读取大文件内容。
with open('large_file.txt', 'r') as file:
for line in file:
print(line.strip())
10.3 使用pandas
库读取大文件
对于大数据集,可以使用 pandas
的 chunksize
参数分块读取文件内容。
import pandas as pd
chunksize = 1000
for chunk in pd.read_csv('large_file.csv', chunksize=chunksize):
print(chunk.head())
总结
通过本文的介绍,我们了解了多种使用Python调用文件内容的方法,包括使用内置的open
函数、通过pandas
库读取数据文件、使用os
库操作文件、使用pathlib
库操作文件、使用csv
库读取和写入CSV文件、使用json
库读取和写入JSON文件、使用pickle
库读取和写入二进制文件、使用io
库操作内存中的文件、使用shutil
库操作文件以及处理大文件的方法。根据具体的需求和文件类型,选择合适的方法可以更加高效地进行文件操作。
相关问答FAQs:
如何使用Python读取文本文件的内容?
要读取文本文件的内容,可以使用Python内置的open()
函数。通过指定文件路径和模式(例如'r'表示只读模式),您可以打开文件并使用read()
方法读取全部内容。示例代码如下:
with open('yourfile.txt', 'r') as file:
content = file.read()
print(content)
使用with
语句可以确保文件在读取后自动关闭,避免资源泄露。
在Python中如何逐行读取文件内容?
逐行读取文件可以使用readline()
或直接在for
循环中遍历文件对象。这样可以节省内存,适合处理大型文件。示例代码为:
with open('yourfile.txt', 'r') as file:
for line in file:
print(line.strip()) # strip()用于去掉行末的换行符
这种方法使您可以逐行处理数据,便于数据分析或处理。
如何在Python中处理文件读取错误?
处理文件读取错误非常重要,您可以使用try-except
语句来捕获异常。例如,文件可能不存在或路径错误。示例代码如下:
try:
with open('yourfile.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件路径。")
except IOError:
print("读取文件时发生错误。")
通过这种方式,您可以优雅地处理可能出现的错误,提高程序的健壮性。
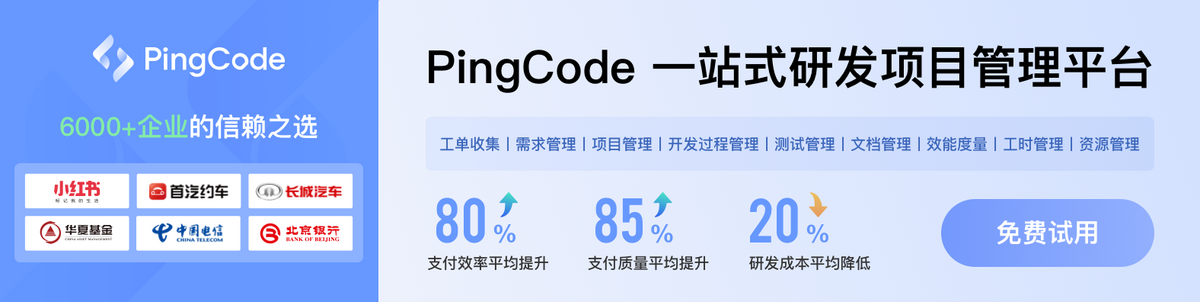