在Python中提取字符的常用方法包括字符串索引、切片操作、使用正则表达式、字符串方法等。其中,最常用的是通过索引和切片来提取字符。例如,通过索引可以直接访问字符串中的单个字符,而通过切片可以获取字符串中的一部分。切片操作尤其强大,可以通过指定开始和结束位置来提取子字符串。正则表达式适用于复杂的匹配和提取需求,可以通过模式匹配来提取特定格式的子字符串。
让我们详细讨论一下字符串切片操作。切片操作允许我们提取字符串的一个子字符串,语法为string[start:stop:step]
。其中,start
表示子字符串的起始位置,stop
表示结束位置(不包括该位置),step
表示步长。例如,string[1:5:2]
将提取从位置1到位置5(不包括5),并且步长为2的字符。
接下来,我们将详细讨论各种方法,并用实际代码示例进行说明。
一、字符串索引
字符串索引是指通过字符串中的位置来访问字符。Python中的字符串是一个有序的字符序列,每个字符都有一个唯一的索引值。字符串索引从0开始,负索引从-1开始,表示从字符串末尾开始计数。
正向索引
正向索引从0开始计数。例如,字符串"hello"
中,字符'h'
的索引是0,字符'e'
的索引是1,依此类推。
string = "hello"
print(string[0]) # 输出 'h'
print(string[1]) # 输出 'e'
负向索引
负向索引从-1开始计数,表示从字符串的末尾开始。例如,字符串"hello"
中,字符'o'
的索引是-1,字符'l'
的索引是-2,依此类推。
string = "hello"
print(string[-1]) # 输出 'o'
print(string[-2]) # 输出 'l'
二、字符串切片
字符串切片是指通过指定开始和结束位置来提取子字符串。切片的语法为string[start:stop:step]
,其中start
表示起始位置,stop
表示结束位置(不包括该位置),step
表示步长。
基本切片
基本切片通过指定起始和结束位置来提取子字符串。例如,字符串"hello"
中,string[1:4]
将提取从位置1到位置4(不包括4)的字符。
string = "hello"
print(string[1:4]) # 输出 'ell'
带步长的切片
带步长的切片通过指定步长来提取子字符串。例如,字符串"hello"
中,string[0:5:2]
将提取从位置0到位置5(不包括5),并且步长为2的字符。
string = "hello"
print(string[0:5:2]) # 输出 'hlo'
省略参数的切片
可以省略切片中的某些参数。例如,省略start
参数表示从字符串的开头开始,省略stop
参数表示一直到字符串的末尾,省略step
参数表示步长为1。
string = "hello"
print(string[:4]) # 输出 'hell'
print(string[2:]) # 输出 'llo'
print(string[::2]) # 输出 'hlo'
三、正则表达式
正则表达式是一种强大的字符串匹配和提取工具。Python的re
模块提供了对正则表达式的支持。通过正则表达式,可以匹配和提取特定格式的子字符串。
基本用法
使用re.search
和re.findall
可以匹配和提取子字符串。例如,匹配并提取字符串中的数字。
import re
string = "abc123def456"
pattern = r'\d+'
matches = re.findall(pattern, string)
print(matches) # 输出 ['123', '456']
分组提取
通过正则表达式中的分组,可以提取特定部分的子字符串。例如,提取字符串中的年份和月份。
import re
string = "2023-10-05"
pattern = r'(\d{4})-(\d{2})-(\d{2})'
match = re.search(pattern, string)
if match:
year, month, day = match.groups()
print(f"Year: {year}, Month: {month}, Day: {day}")
四、字符串方法
Python的字符串方法提供了许多方便的操作来提取和操作字符串。例如,split
方法可以将字符串分割成子字符串列表,replace
方法可以替换子字符串,join
方法可以将子字符串列表连接成一个字符串。
split方法
split
方法通过指定分隔符将字符串分割成子字符串列表。例如,将字符串按空格分割成单词列表。
string = "hello world"
words = string.split(' ')
print(words) # 输出 ['hello', 'world']
replace方法
replace
方法通过指定的旧子字符串和新子字符串来替换字符串中的子字符串。例如,将字符串中的'hello'
替换为'hi'
。
string = "hello world"
new_string = string.replace('hello', 'hi')
print(new_string) # 输出 'hi world'
join方法
join
方法通过指定的连接符将子字符串列表连接成一个字符串。例如,将单词列表按空格连接成一个字符串。
words = ['hello', 'world']
string = ' '.join(words)
print(string) # 输出 'hello world'
五、字符串格式化
字符串格式化是指通过指定格式将变量值插入到字符串中。Python提供了多种字符串格式化方法,包括%
操作符、str.format
方法和f字符串(f-strings)。
%操作符
%
操作符通过指定格式将变量值插入到字符串中。例如,将整数变量插入到字符串中。
name = "Alice"
age = 30
string = "Name: %s, Age: %d" % (name, age)
print(string) # 输出 'Name: Alice, Age: 30'
str.format方法
str.format
方法通过指定格式将变量值插入到字符串中。例如,将整数变量插入到字符串中。
name = "Alice"
age = 30
string = "Name: {}, Age: {}".format(name, age)
print(string) # 输出 'Name: Alice, Age: 30'
f字符串
f字符串通过在字符串前添加f
,并在字符串中使用大括号{}
插入变量值。例如,将整数变量插入到字符串中。
name = "Alice"
age = 30
string = f"Name: {name}, Age: {age}"
print(string) # 输出 'Name: Alice, Age: 30'
六、字符串遍历
字符串遍历是指逐个访问字符串中的每个字符。可以使用for
循环或while
循环来遍历字符串。
for循环遍历
使用for
循环遍历字符串中的每个字符。例如,遍历字符串并打印每个字符。
string = "hello"
for char in string:
print(char)
while循环遍历
使用while
循环遍历字符串中的每个字符。例如,通过索引遍历字符串并打印每个字符。
string = "hello"
index = 0
while index < len(string):
print(string[index])
index += 1
七、字符串查找
字符串查找是指在字符串中搜索特定子字符串。Python的字符串方法提供了多种查找方法,包括find
、rfind
、index
、rindex
等。
find方法
find
方法返回子字符串在字符串中第一次出现的位置,如果没有找到则返回-1。例如,在字符串中查找子字符串'world'
。
string = "hello world"
position = string.find('world')
print(position) # 输出 6
rfind方法
rfind
方法返回子字符串在字符串中最后一次出现的位置,如果没有找到则返回-1。例如,在字符串中查找子字符串'o'
。
string = "hello world"
position = string.rfind('o')
print(position) # 输出 7
index方法
index
方法返回子字符串在字符串中第一次出现的位置,如果没有找到则抛出ValueError
异常。例如,在字符串中查找子字符串'world'
。
string = "hello world"
position = string.index('world')
print(position) # 输出 6
rindex方法
rindex
方法返回子字符串在字符串中最后一次出现的位置,如果没有找到则抛出ValueError
异常。例如,在字符串中查找子字符串'o'
。
string = "hello world"
position = string.rindex('o')
print(position) # 输出 7
八、字符串替换
字符串替换是指在字符串中用新的子字符串替换旧的子字符串。Python的字符串方法提供了多种替换方法,包括replace
、translate
等。
replace方法
replace
方法通过指定的旧子字符串和新子字符串来替换字符串中的子字符串。例如,将字符串中的'hello'
替换为'hi'
。
string = "hello world"
new_string = string.replace('hello', 'hi')
print(new_string) # 输出 'hi world'
translate方法
translate
方法通过指定的转换表来替换字符串中的字符。例如,将字符串中的'h'
替换为'H'
。
string = "hello world"
translation_table = str.maketrans('h', 'H')
new_string = string.translate(translation_table)
print(new_string) # 输出 'Hello world'
九、字符串分割
字符串分割是指通过指定分隔符将字符串分割成子字符串列表。Python的字符串方法提供了多种分割方法,包括split
、rsplit
、splitlines
等。
split方法
split
方法通过指定分隔符将字符串分割成子字符串列表。例如,将字符串按空格分割成单词列表。
string = "hello world"
words = string.split(' ')
print(words) # 输出 ['hello', 'world']
rsplit方法
rsplit
方法从右向左通过指定分隔符将字符串分割成子字符串列表。例如,将字符串按空格从右向左分割成单词列表。
string = "hello world"
words = string.rsplit(' ', 1)
print(words) # 输出 ['hello', 'world']
splitlines方法
splitlines
方法按行分割字符串。例如,将多行字符串分割成行列表。
string = "hello\nworld"
lines = string.splitlines()
print(lines) # 输出 ['hello', 'world']
十、字符串连接
字符串连接是指通过指定的连接符将子字符串列表连接成一个字符串。Python的字符串方法提供了多种连接方法,包括join
、concatenation
等。
join方法
join
方法通过指定的连接符将子字符串列表连接成一个字符串。例如,将单词列表按空格连接成一个字符串。
words = ['hello', 'world']
string = ' '.join(words)
print(string) # 输出 'hello world'
字符串拼接
字符串拼接是指通过+
操作符将多个字符串连接成一个字符串。例如,将两个字符串连接成一个字符串。
string1 = "hello"
string2 = "world"
new_string = string1 + " " + string2
print(new_string) # 输出 'hello world'
十一、字符串编码和解码
字符串编码是指将字符串转换为字节序列,字符串解码是指将字节序列转换为字符串。Python的字符串方法提供了多种编码和解码方法,包括encode
、decode
等。
encode方法
encode
方法将字符串转换为字节序列。例如,将字符串转换为UTF-8编码的字节序列。
string = "hello"
bytes_string = string.encode('utf-8')
print(bytes_string) # 输出 b'hello'
decode方法
decode
方法将字节序列转换为字符串。例如,将UTF-8编码的字节序列转换为字符串。
bytes_string = b'hello'
string = bytes_string.decode('utf-8')
print(string) # 输出 'hello'
十二、字符串比较
字符串比较是指比较两个字符串的大小。Python的字符串方法提供了多种比较方法,包括==
、!=
、>
、<
、>=
、<=
等。
相等比较
相等比较是指比较两个字符串是否相等。例如,比较两个字符串是否相等。
string1 = "hello"
string2 = "hello"
print(string1 == string2) # 输出 True
大小比较
大小比较是指比较两个字符串的大小。例如,比较两个字符串的大小。
string1 = "hello"
string2 = "world"
print(string1 < string2) # 输出 True
十三、字符串常用操作
Python提供了许多常用的字符串操作方法,包括strip
、upper
、lower
、capitalize
、title
、count
等。
strip方法
strip
方法去除字符串两端的空白字符。例如,去除字符串两端的空白字符。
string = " hello "
new_string = string.strip()
print(new_string) # 输出 'hello'
upper方法
upper
方法将字符串转换为大写。例如,将字符串转换为大写。
string = "hello"
new_string = string.upper()
print(new_string) # 输出 'HELLO'
lower方法
lower
方法将字符串转换为小写。例如,将字符串转换为小写。
string = "HELLO"
new_string = string.lower()
print(new_string) # 输出 'hello'
capitalize方法
capitalize
方法将字符串的第一个字符转换为大写,其他字符转换为小写。例如,将字符串的第一个字符转换为大写。
string = "hello"
new_string = string.capitalize()
print(new_string) # 输出 'Hello'
title方法
title
方法将字符串中的每个单词的第一个字符转换为大写。例如,将字符串中的每个单词的第一个字符转换为大写。
string = "hello world"
new_string = string.title()
print(new_string) # 输出 'Hello World'
count方法
count
方法返回子字符串在字符串中出现的次数。例如,返回子字符串'l'
在字符串中出现的次数。
string = "hello"
count = string.count('l')
print(count) # 输出 2
十四、字符串反转
字符串反转是指将字符串中的字符顺序颠倒。Python提供了多种字符串反转方法,包括切片操作、reversed
函数等。
切片操作反转
通过切片操作可以反转字符串。例如,反转字符串。
string = "hello"
reversed_string = string[::-1]
print(reversed_string) # 输出 'olleh'
reversed函数反转
通过reversed
函数可以反转字符串。例如,反转字符串。
string = "hello"
reversed_string = ''.join(reversed(string))
print(reversed_string) # 输出 'olleh'
十五、字符串去重
字符串去重是指去除字符串中的重复字符。Python提供了多种字符串去重方法,包括set
集合、OrderedDict
等。
set集合去重
通过set
集合可以去除字符串中的重复字符。例如,去除字符串中的重复字符。
string = "hello"
unique_chars = ''.join(set(string))
print(unique_chars) # 输出 'helo'(顺序可能不一致)
OrderedDict去重
通过OrderedDict
可以保持字符顺序去重。例如,去除字符串中的重复字符并保持顺序。
from collections import OrderedDict
string = "hello"
unique_chars = ''.join(OrderedDict.fromkeys(string))
print(unique
相关问答FAQs:
如何在Python中提取特定字符或字符串?
在Python中,可以使用多种方法提取特定字符或子字符串。常见的方法包括使用切片、字符串的find()
和index()
方法,以及正则表达式。切片允许你通过指定起始和结束索引来提取字符串的一部分。find()
方法可以找到子字符串的起始位置,而index()
方法则类似,但在子字符串不存在时会引发错误。使用正则表达式则适合需要更复杂模式匹配的场景。
Python中有哪些内置函数可以帮助提取字符?
Python提供了丰富的内置字符串方法来帮助用户提取字符。例如,split()
方法可以根据指定的分隔符将字符串分割为多个部分,而strip()
方法可以去除字符串两端的空白字符。此外,replace()
方法可以替换字符串中的特定字符或子字符串,这在提取字符时也非常有用。
是否可以使用正则表达式来提取符合特定模式的字符?
正则表达式是处理字符串的强大工具,可以用来提取符合特定模式的字符。使用re
模块中的findall()
函数,可以找到所有匹配的字符串并返回一个列表。此方法非常适合需要从复杂字符串中提取特定格式的数据,比如电子邮件地址、电话号码等。通过编写正则表达式,用户可以灵活定义所需的字符模式。
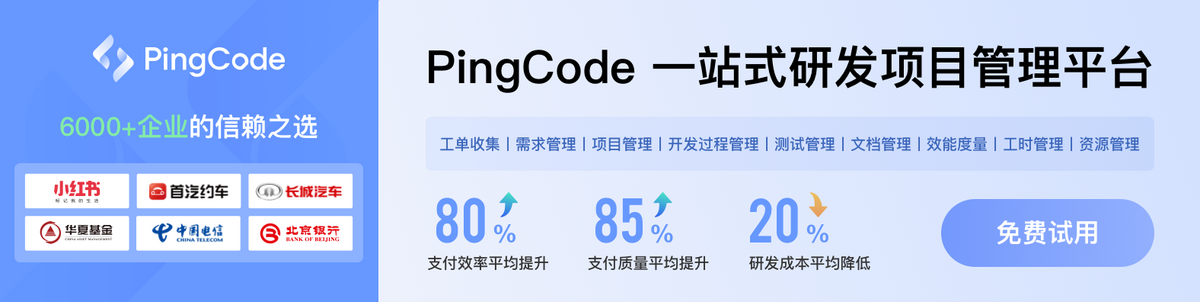