使用Python爬数据通常涉及以下几个步骤:选择合适的Python库、发送HTTP请求、解析网页内容、提取所需数据、存储数据。其中,选择合适的Python库是非常重要的一步。Python有多个流行的库可以用来进行网页爬取和解析,比如requests
、BeautifulSoup
、Scrapy
等。下面我们将详细描述如何使用这些库进行数据爬取。
一、选择合适的Python库
选择合适的Python库是爬取网页数据的第一步。requests
库用于发送HTTP请求,它简单易用,适合初学者。BeautifulSoup
库用于解析HTML和XML文档,它可以轻松地从网页中提取所需的数据。Scrapy
是一个功能强大的爬虫框架,适合需要爬取大量数据或需要更复杂的爬虫逻辑的项目。
1. Requests库
requests
库是一个简单易用的HTTP库,它使得发送HTTP请求变得非常简单。你可以使用requests
库发送GET、POST等请求,并获取网页的响应内容。以下是一个简单的示例:
import requests
response = requests.get('https://example.com')
print(response.text)
2. BeautifulSoup库
BeautifulSoup
库用于解析HTML和XML文档,它可以轻松地从网页中提取所需的数据。你可以将requests
库获取的网页内容传递给BeautifulSoup
进行解析。以下是一个简单的示例:
from bs4 import BeautifulSoup
import requests
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.text)
二、发送HTTP请求
发送HTTP请求是爬取网页数据的第二步。通过发送HTTP请求,获取网页的HTML内容。可以使用requests
库来实现这一点。
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.status_code)
print(response.text)
在发送HTTP请求时,可以添加请求头、参数等,以模拟真实的浏览器行为,避免被网站屏蔽。
三、解析网页内容
解析网页内容是爬取网页数据的第三步。可以使用BeautifulSoup
库来解析HTML文档,从中提取所需的数据。
from bs4 import BeautifulSoup
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
通过BeautifulSoup
库,可以轻松地获取网页中的特定元素,如标题、段落、链接等。
四、提取所需数据
提取所需数据是爬取网页数据的第四步。可以使用BeautifulSoup
库提供的各种方法来提取网页中的特定元素。
title = soup.title.text
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
print(paragraph.text)
五、存储数据
存储数据是爬取网页数据的最后一步。可以将提取的数据存储到文件、数据库等。以下是一个将数据存储到CSV文件的示例:
import csv
data = [{'title': title, 'paragraph': p.text} for p in paragraphs]
with open('data.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.DictWriter(file, fieldnames=['title', 'paragraph'])
writer.writeheader()
writer.writerows(data)
六、使用Scrapy框架
Scrapy
是一个功能强大的爬虫框架,适合需要爬取大量数据或需要更复杂的爬虫逻辑的项目。使用Scrapy
框架可以更高效地进行数据爬取。
1. 安装Scrapy
首先,使用pip安装Scrapy:
pip install scrapy
2. 创建Scrapy项目
创建Scrapy项目,进入项目目录,生成爬虫:
scrapy startproject myproject
cd myproject
scrapy genspider myspider example.com
3. 编写爬虫代码
编辑生成的爬虫代码myspider.py
,编写爬取逻辑:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
paragraphs = response.css('p::text').getall()
yield {
'title': title,
'paragraphs': paragraphs,
}
4. 运行爬虫
使用以下命令运行爬虫:
scrapy crawl myspider -o data.json
这样,爬取的数据将被存储到data.json
文件中。
七、处理反爬机制
在进行网页数据爬取时,可能会遇到网站的反爬机制。为了绕过反爬机制,可以采取以下措施:
1. 添加请求头
在发送HTTP请求时,添加请求头以模拟真实的浏览器行为:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
2. 使用代理
使用代理可以隐藏真实的IP地址,避免被网站屏蔽:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, headers=headers, proxies=proxies)
3. 设置请求延迟
在发送HTTP请求时,设置请求延迟以避免频繁访问同一网站:
import time
time.sleep(5)
response = requests.get(url, headers=headers)
八、处理动态网页
有些网页是动态生成的,使用传统的静态爬虫方法无法获取其内容。可以使用Selenium库来处理动态网页。
1. 安装Selenium
首先,使用pip安装Selenium:
pip install selenium
2. 安装浏览器驱动
下载并安装与浏览器版本匹配的浏览器驱动,如ChromeDriver或GeckoDriver。
3. 使用Selenium爬取动态网页
使用Selenium模拟浏览器访问网页,并获取其内容:
from selenium import webdriver
设置浏览器驱动路径
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
driver.get('https://example.com')
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
driver.quit()
九、处理登录机制
有些网站需要登录才能访问其内容。可以使用requests
库或Selenium
库来模拟登录过程。
1. 使用Requests库模拟登录
使用requests
库发送POST请求进行登录,并维护会话:
import requests
login_url = 'https://example.com/login'
data = {
'username': 'your_username',
'password': 'your_password'
}
session = requests.Session()
session.post(login_url, data=data)
response = session.get('https://example.com/protected_page')
print(response.text)
2. 使用Selenium库模拟登录
使用Selenium
库模拟用户在浏览器中登录:
from selenium import webdriver
driver = webdriver.Chrome(executable_path='path/to/chromedriver')
driver.get('https://example.com/login')
输入用户名和密码
username_input = driver.find_element_by_name('username')
password_input = driver.find_element_by_name('password')
username_input.send_keys('your_username')
password_input.send_keys('your_password')
提交登录表单
login_button = driver.find_element_by_xpath('//button[@type="submit"]')
login_button.click()
获取登录后的页面内容
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
driver.quit()
十、处理验证码
有些网站在登录时需要输入验证码。可以使用第三方服务或OCR技术来处理验证码。
1. 使用第三方服务
可以使用第三方服务,如2Captcha,来处理验证码。需要先注册并获取API密钥。
import requests
captcha_image_url = 'https://example.com/captcha_image'
captcha_image_response = requests.get(captcha_image_url)
captcha_image_data = captcha_image_response.content
api_key = 'your_2captcha_api_key'
captcha_response = requests.post(
'https://2captcha.com/in.php',
files={'file': captcha_image_data},
data={'key': api_key}
)
captcha_id = captcha_response.text.split('|')[1]
等待验证码识别完成
import time
time.sleep(20)
captcha_result_response = requests.get(
f'https://2captcha.com/res.php?key={api_key}&action=get&id={captcha_id}'
)
captcha_text = captcha_result_response.text.split('|')[1]
print(captcha_text)
2. 使用OCR技术
可以使用OCR技术,如Tesseract,来识别验证码。需要安装Tesseract和其Python绑定库pytesseract
。
import pytesseract
from PIL import Image
captcha_image = Image.open('path/to/captcha_image.png')
captcha_text = pytesseract.image_to_string(captcha_image)
print(captcha_text)
十一、处理多页面数据
有时候需要从多个页面提取数据,可以使用循环或递归的方法来处理多页面数据。
1. 使用循环处理多页面数据
使用循环遍历多个页面,并提取数据:
import requests
from bs4 import BeautifulSoup
base_url = 'https://example.com/page/'
for page_num in range(1, 11):
url = f'{base_url}{page_num}'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
items = soup.find_all('div', class_='item')
for item in items:
print(item.text)
2. 使用递归处理多页面数据
使用递归方法遍历多个页面,并提取数据:
import requests
from bs4 import BeautifulSoup
def scrape_page(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
items = soup.find_all('div', class_='item')
for item in items:
print(item.text)
next_page = soup.find('a', class_='next')
if next_page:
next_page_url = next_page['href']
scrape_page(next_page_url)
start_url = 'https://example.com/page/1'
scrape_page(start_url)
十二、处理数据清洗与存储
在提取数据后,可能需要对数据进行清洗和存储。可以使用Pandas库来处理数据清洗,并将数据存储到CSV、Excel等格式。
1. 安装Pandas
首先,使用pip安装Pandas:
pip install pandas
2. 数据清洗与存储
使用Pandas库进行数据清洗,并将数据存储到CSV文件:
import pandas as pd
data = [{'title': title, 'paragraph': p.text} for p in paragraphs]
df = pd.DataFrame(data)
df.drop_duplicates(inplace=True)
df.to_csv('data_cleaned.csv', index=False, encoding='utf-8')
十三、总结
爬取网页数据的过程包括选择合适的Python库、发送HTTP请求、解析网页内容、提取所需数据、存储数据等步骤。在实际操作中,还需要处理反爬机制、动态网页、登录机制、验证码、多页面数据等问题。通过综合使用requests
、BeautifulSoup
、Scrapy
、Selenium
等库和工具,可以高效地完成数据爬取任务。希望这篇文章能够帮助你更好地理解和掌握Python爬取数据的技术。
相关问答FAQs:
在使用Python爬取数据时,应该选择哪些库?
在Python中,常用的爬虫库包括Requests和BeautifulSoup。Requests库用于发送HTTP请求,而BeautifulSoup则可以解析HTML和XML文档,方便提取数据。Scrapy是一个功能强大的框架,适合大规模的数据爬取。选择合适的库取决于项目的复杂性和数据格式。
爬虫过程中如何处理网站的反爬虫机制?
许多网站会实施反爬虫措施以保护自己的数据。使用User-Agent伪装、设置请求间隔、使用代理IP以及模拟浏览器行为都是常见的方法。此外,遵循网站的robots.txt文件规则以及避免过于频繁的请求,可以有效降低被封禁的风险。
在爬取数据后,如何对数据进行清洗和存储?
爬取到的数据通常需要清洗,以去除重复项、修正格式或填补缺失值。Pandas库在数据处理方面非常强大,可以方便地进行数据清洗和分析。存储数据的方式可以多样化,包括保存为CSV文件、Excel文件,或使用数据库如SQLite、MySQL等,具体选择应根据数据量和后续分析需求来决定。
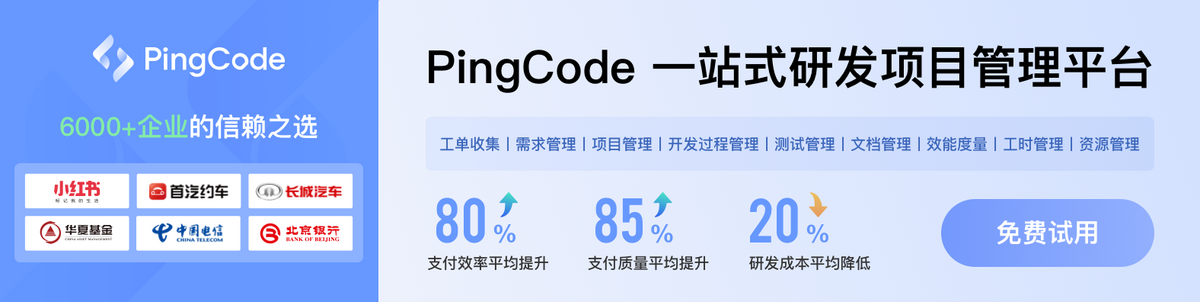