在Python中,可以通过多种方法来显示直方图的数字,如使用Matplotlib、Seaborn等库。使用Matplotlib的hist函数、使用Seaborn的histplot函数、通过调用bar_label方法等。下面详细介绍其中一种方法:使用Matplotlib的hist函数,并在直方图上显示每个柱的数字。
一、MATPLOTLIB绘制直方图
Matplotlib是Python中最常用的数据可视化库之一,能够非常方便地绘制各种图表。绘制直方图是其基本功能之一。
1、导入必要的库
首先,我们需要导入Matplotlib库和其他必要的库,如numpy。
import matplotlib.pyplot as plt
import numpy as np
2、生成示例数据
为了绘制直方图,我们需要一些数据。我们可以使用numpy库生成随机数据。
data = np.random.randn(1000)
3、绘制直方图
使用Matplotlib的hist函数绘制直方图。
plt.hist(data, bins=30, edgecolor='black')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要计算每个柱的高度,并在相应的位置添加文本。
n, bins, patches = plt.hist(data, bins=30, edgecolor='black')
for i in range(len(patches)):
plt.text(patches[i].get_x() + patches[i].get_width() / 2, patches[i].get_height(), str(int(patches[i].get_height())), ha='center', va='bottom')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram with Numbers')
plt.show()
二、SEABORN绘制直方图
Seaborn是基于Matplotlib的高级数据可视化库,提供了更简洁的API和更漂亮的默认样式。使用Seaborn绘制直方图也非常简单。
1、导入必要的库
首先,我们需要导入Seaborn库和其他必要的库,如Matplotlib和numpy。
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
2、生成示例数据
同样,我们可以使用numpy库生成随机数据。
data = np.random.randn(1000)
3、绘制直方图
使用Seaborn的histplot函数绘制直方图。
sns.histplot(data, bins=30, kde=False)
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要获取直方图的高度,并在相应的位置添加文本。
ax = sns.histplot(data, bins=30, kde=False)
for p in ax.patches:
ax.annotate(str(int(p.get_height())), (p.get_x() + p.get_width() / 2, p.get_height()), ha='center', va='bottom')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram with Numbers')
plt.show()
三、PANDAS绘制直方图
Pandas是Python中最常用的数据分析库之一,提供了方便的数据处理和可视化方法。使用Pandas绘制直方图也非常简单。
1、导入必要的库
首先,我们需要导入Pandas库和其他必要的库,如Matplotlib和numpy。
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
2、生成示例数据
我们可以使用numpy库生成随机数据,并将其转换为Pandas的DataFrame。
data = pd.DataFrame({'value': np.random.randn(1000)})
3、绘制直方图
使用Pandas的hist方法绘制直方图。
ax = data['value'].plot.hist(bins=30, edgecolor='black')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram')
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要获取直方图的高度,并在相应的位置添加文本。
n, bins, patches = ax.hist(data['value'], bins=30, edgecolor='black')
for i in range(len(patches)):
ax.text(patches[i].get_x() + patches[i].get_width() / 2, patches[i].get_height(), str(int(patches[i].get_height())), ha='center', va='bottom')
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram with Numbers')
plt.show()
四、PLOTLY绘制直方图
Plotly是一个开源的数据可视化库,支持丰富的交互式图表。使用Plotly绘制直方图也非常简单。
1、导入必要的库
首先,我们需要导入Plotly库和其他必要的库,如numpy。
import plotly.express as px
import numpy as np
2、生成示例数据
我们可以使用numpy库生成随机数据。
data = np.random.randn(1000)
3、绘制直方图
使用Plotly的express模块中的histogram函数绘制直方图。
fig = px.histogram(data, nbins=30)
fig.update_layout(title='Histogram', xaxis_title='Value', yaxis_title='Frequency')
fig.show()
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要获取直方图的高度,并在相应的位置添加文本。
import plotly.graph_objects as go
fig = px.histogram(data, nbins=30)
for bin in fig.data[0]['histfunc']:
fig.add_trace(go.Scatter(
x=[bin['x']],
y=[bin['y']],
text=[str(bin['y'])],
mode='text',
textposition='top center'
))
fig.update_layout(title='Histogram with Numbers', xaxis_title='Value', yaxis_title='Frequency')
fig.show()
五、其他绘图库
除了上述提到的Matplotlib、Seaborn、Pandas和Plotly,还有其他一些绘图库也可以用于绘制直方图,如Bokeh、Altair等。
Bokeh是一个交互式数据可视化库,支持浏览器中的交互式图表。使用Bokeh绘制直方图也非常简单。
1、导入必要的库
首先,我们需要导入Bokeh库和其他必要的库,如numpy。
from bokeh.plotting import figure, show
from bokeh.io import output_notebook
import numpy as np
2、生成示例数据
我们可以使用numpy库生成随机数据。
data = np.random.randn(1000)
3、绘制直方图
使用Bokeh的figure函数创建一个绘图对象,并使用quad函数绘制直方图。
hist, edges = np.histogram(data, bins=30)
p = figure(title='Histogram', x_axis_label='Value', y_axis_label='Frequency')
p.quad(top=hist, bottom=0, left=edges[:-1], right=edges[1:], line_color='black')
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要计算每个柱的高度,并在相应的位置添加文本。
for i in range(len(hist)):
p.text(x=(edges[i] + edges[i+1]) / 2, y=hist[i], text=[str(hist[i])], text_align='center', text_baseline='middle')
show(p)
Altair是一个声明式数据可视化库,基于Vega和Vega-Lite。使用Altair绘制直方图也非常简单。
1、导入必要的库
首先,我们需要导入Altair库和其他必要的库,如pandas和numpy。
import altair as alt
import pandas as pd
import numpy as np
2、生成示例数据
我们可以使用numpy库生成随机数据,并将其转换为Pandas的DataFrame。
data = pd.DataFrame({'value': np.random.randn(1000)})
3、绘制直方图
使用Altair的Chart函数创建一个绘图对象,并使用mark_bar函数绘制直方图。
chart = alt.Chart(data).mark_bar().encode(
x=alt.X('value', bin=alt.Bin(maxbins=30)),
y='count()'
).properties(
title='Histogram'
)
4、显示直方图上的数字
为了在直方图上显示每个柱的数字,我们需要计算每个柱的高度,并在相应的位置添加文本。
text = chart.mark_text(
align='center',
baseline='middle',
dy=-10
).encode(
text='count()'
)
chart + text
总结
在Python中,可以通过多种方法来显示直方图的数字,如使用Matplotlib、Seaborn、Pandas、Plotly、Bokeh、Altair等库。每种方法都有其优点和适用场景,选择合适的方法可以更好地满足数据可视化的需求。希望通过本文的介绍,能够帮助大家掌握在直方图上显示数字的方法,提高数据可视化的能力。
相关问答FAQs:
如何在Python中绘制直方图并显示数值?
在Python中,可以使用Matplotlib库来绘制直方图并显示每个条形的数值。首先,确保已安装Matplotlib。可以通过pip install matplotlib
来安装。然后,使用plt.bar
函数绘制直方图,并使用plt.text
函数在每个条形上方显示数值。
使用哪些库可以绘制直方图并显示数值?
除了Matplotlib,Seaborn也是一个非常流行的可视化库,能够轻松绘制直方图并显示数值。Seaborn基于Matplotlib,提供了更高层次的抽象,使用起来更为简便。可以使用seaborn.histplot
函数绘制直方图,并通过设置stat='count'
来显示每个条形的数值。
如何自定义直方图的外观和显示方式?
在绘制直方图时,可以通过Matplotlib的多种参数自定义外观,例如颜色、边框、宽度等。使用plt.bar
时,可以调整color
和edgecolor
参数,plt.text
可以设置字体大小和颜色。在显示数值时,可以选择将数值放置在条形的顶部或内部,具体取决于数据的可读性。
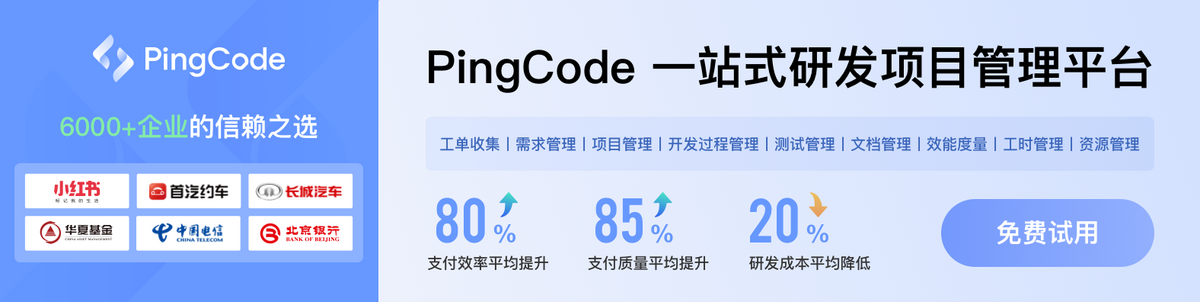